- If you want to follow, create a new MFC Application named Corporate
and accept all options, that is, click Finish on the wizard
- Using the Add Resource (Project -> Add Resource...) dialog box,
add a new Dialog
- Change its ID to IDD_LOGIN_DLG and its Caption to Members Login
- Design the dialog as follows:
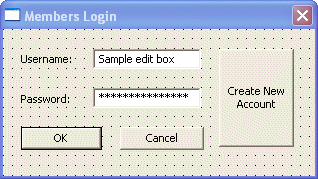 |
Control |
ID |
Caption |
Other Properties |
Static Text |
|
Username |
|
Edit Control |
IDC_USERNAME |
|
|
Static Text |
|
Password |
|
Edit Control |
IDC_PASSWORD |
|
Password: True |
Button |
IDC_NEWACCOUNT_BTN |
Create New Account |
Multiline: True |
|
- Add a new class for the dialog box and save it as CLoginDlg. Make
sure the class is based on CDialog
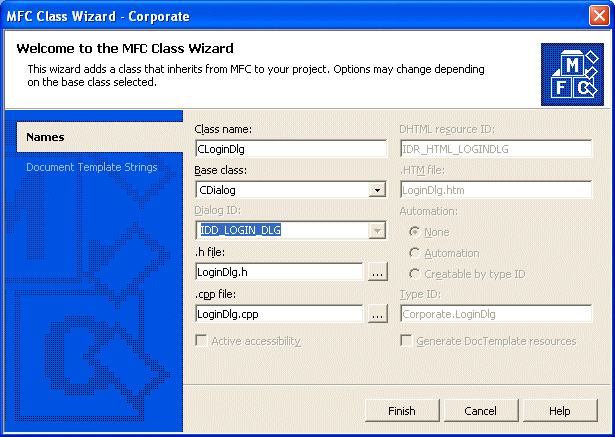
- Add the following variables associated to the controls:
ID |
Control Variable |
Value Variable |
|
Type |
Name |
Type |
Name |
IDC_USERNAME |
CEdit |
m_EditUsername |
|
|
IDC_USERNAME |
|
|
CString |
m_Username |
IDC_PASSWORD |
|
|
CString |
m_Password |
- Using the Add Resource dialog box, add a new Dialog
- Change its ID to IDD_NEWACCOUNT_DLG and its Caption to New
Account Creation
- Design the dialog as follows:
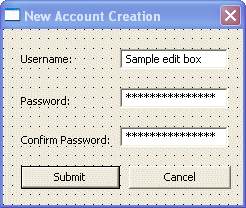 |
Control |
ID |
Caption |
Other Properties |
Static Text |
|
Username |
|
Edit Control |
IDC_USERNAME |
|
|
Static Text |
|
Password |
|
Edit Control |
IDC_PASSWORD |
|
Password: True |
Static Text |
|
Confirm Password |
|
Edit Control |
IDC_CONFIRM_PASSWORD |
|
Password: True |
Button |
IDOK |
Submit |
|
|
- Add a new class for the dialog box and save it as CNewAccountDlg. Make
sure the class is based on CDialog
- Add the following variables associated to the controls:
ID |
Control Variable |
Value Variable |
|
Type |
Name |
Type |
Name |
IDC_USERNAME |
CEdit |
m_EditUsername |
|
|
IDC_USERNAME |
|
|
CString |
m_Username |
IDC_PASSWORD |
CEdit |
m_EditPassword |
|
|
IDC_PASSWORD |
|
|
CString |
m_Password |
IDC_CONFIRM_PASSWORD |
|
|
CString |
m_ConfirmPassword |
- While still in the second dialog box, double-click the Submit button
and implement its event as follows:
void CNewAccountDlg::OnBnClickedOk()
{
// TODO: Add your control notification handler code here
UpdateData();
// Make sure the user provided a username
if( m_Username == "" )
{
AfxMessageBox("You must provide a username");
m_EditUsername.SetFocus();
return;
}
// Don't allow a blank password
if( m_Password == "" )
{
AfxMessageBox("Blank passwords are not allowed\n"
"Please provide a password");
m_EditPassword.SetFocus();
return;
}
// The password and the blank password must be the same
if( m_ConfirmPassword != m_Password )
{
AfxMessageBox("The passwords you provided are not the same");
m_EditPassword.SetFocus();
return;
}
FILE *fleCredentials;
try {
// Create a new file or open the existing one
fleCredentials = fopen("credentials.crd", "a+");
// Add the username to the file
fprintf(fleCredentials, "%s ", (LPCTSTR)m_Username);
// Add the password to the file
fprintf(fleCredentials, "%s\n", (LPCTSTR)m_Password);
// After using it, close the file
fclose(fleCredentials);
}
catch(...)
{
// Did something go wrong???
AfxMessageBox("Could not validate the credentials");
}
UpdateData(FALSE);
OnOK();
}
|
- Display the first dialog box, double-click its Create New Account
button and implement its event as follows:
// LoginDlg.cpp : implementation file
//
#include "stdafx.h"
#include "Corporate.h"
#include "LoginDlg.h"
#include ".\logindlg.h"
#include "NewAccountDlg.h"
// CLoginDlg dialog
IMPLEMENT_DYNAMIC(CLoginDlg, CDialog)
CLoginDlg::CLoginDlg(CWnd* pParent /*=NULL*/)
: CDialog(CLoginDlg::IDD, pParent)
, m_Username(_T(""))
, m_Password(_T(""))
{
}
. . .
void CLoginDlg::OnBnClickedNewaccountBtn()
{
// TODO: Add your control notification handler code here
CNewAccountDlg Dlg;
Dlg.DoModal();
}
|
- Double-click the OK button and implement its event as follows:
void CLoginDlg::OnBnClickedOk()
{
// TODO: Add your control notification handler code here
UpdateData();
char UsernameFromFile[20], PasswordFromFile[20];
FILE *fleCredentials;
bool ValidLogin = false;
if( m_Username == "" )
{
AfxMessageBox("You must provide a username and a password or click Cancel");
return;
}
if( m_Password == "" )
{
AfxMessageBox("Invalid Login");
return;
}
try {
// Open the file for reading
fleCredentials = fopen("credentials.crd", "r");
// Scan the file from beginning to end
while( !feof(fleCredentials) )
{
// Read a username
fscanf(fleCredentials, "%s", UsernameFromFile);
// Compare the typed username with the username from the file
if( strcmp((LPCTSTR)m_Username, UsernameFromFile) == 0 )
{
// With the current username, retrieve the corresponding password
fscanf(fleCredentials, "%s", PasswordFromFile);
// Compare the typed password with the one on file
if( strcmp((LPCTSTR)m_Password, PasswordFromFile) == 0 )
{
ValidLogin = true;
}
else
ValidLogin = false;
}
}
if( ValidLogin == true )
OnOK();
else
{
AfxMessageBox("Invalid Credentials. Please try again");
this->m_EditUsername.SetFocus();
}
fclose(fleCredentials);
}
catch(...)
{
AfxMessageBox("Could not validate the credentials");
}
UpdateData(FALSE);
}
|
- In the Solution Explorer, double-click the Corporate.cpp file and
change its InitInstance() event as follows:
// Corporate.cpp : Defines the class behaviors for the application.
//
#include "stdafx.h"
#include "Corporate.h"
#include "MainFrm.h"
#include "ChildFrm.h"
#include "CorporateDoc.h"
#include "CorporateView.h"
#include "LoginDlg.h"
#ifdef _DEBUG
#define new DEBUG_NEW
#endif
// CCorporateApp
BEGIN_MESSAGE_MAP(CCorporateApp, CWinApp)
ON_COMMAND(ID_APP_ABOUT, OnAppAbout)
// Standard file based document commands
ON_COMMAND(ID_FILE_NEW, CWinApp::OnFileNew)
ON_COMMAND(ID_FILE_OPEN, CWinApp::OnFileOpen)
// Standard print setup command
ON_COMMAND(ID_FILE_PRINT_SETUP, CWinApp::OnFilePrintSetup)
END_MESSAGE_MAP()
// CCorporateApp construction
CCorporateApp::CCorporateApp()
{
// TODO: add construction code here,
// Place all significant initialization in InitInstance
}
// The one and only CCorporateApp object
CCorporateApp theApp;
// CCorporateApp initialization
BOOL CCorporateApp::InitInstance()
{
// InitCommonControls() is required on Windows XP if an application
// manifest specifies use of ComCtl32.dll version 6 or later to enable
// visual styles. Otherwise, any window creation will fail.
InitCommonControls();
CWinApp::InitInstance();
// Initialize OLE libraries
if (!AfxOleInit())
{
AfxMessageBox(IDP_OLE_INIT_FAILED);
return FALSE;
}
AfxEnableControlContainer();
// Standard initialization
// If you are not using these features and wish to reduce the size
// of your final executable, you should remove from the following
// the specific initialization routines you do not need
// Change the registry key under which our settings are stored
// TODO: You should modify this string to be something appropriate
// such as the name of your company or organization
SetRegistryKey(_T("Local AppWizard-Generated Applications"));
LoadStdProfileSettings(4); // Load standard INI file options (including MRU)
// Register the application's document templates. Document templates
// serve as the connection between documents, frame windows and views
CMultiDocTemplate* pDocTemplate;
pDocTemplate = new CMultiDocTemplate(IDR_CorporateTYPE,
RUNTIME_CLASS(CCorporateDoc),
RUNTIME_CLASS(CChildFrame), // custom MDI child frame
RUNTIME_CLASS(CCorporateView));
if (!pDocTemplate)
return FALSE;
AddDocTemplate(pDocTemplate);
// Prepare to display the Login dialog box
CLoginDlg Dlg;
// Display the dialog box and find out if the user clicked OK
if( Dlg.DoModal() == IDOK )
{
// create main MDI Frame window
CMainFrame* pMainFrame = new CMainFrame;
if (!pMainFrame || !pMainFrame->LoadFrame(IDR_MAINFRAME))
return FALSE;
m_pMainWnd = pMainFrame;
// call DragAcceptFiles only if there's a suffix
// In an MDI app, this should occur immediately after setting m_pMainWnd
// Parse command line for standard shell commands, DDE, file open
CCommandLineInfo cmdInfo;
ParseCommandLine(cmdInfo);
// Dispatch commands specified on the command line. Will return FALSE if
// app was launched with /RegServer, /Register, /Unregserver or /Unregister.
if (!ProcessShellCommand(cmdInfo))
return FALSE;
// The main window has been initialized, so show and update it
pMainFrame->ShowWindow(m_nCmdShow);
pMainFrame->UpdateWindow();
}
return TRUE;
}
|
- Execute the application. Make sure you create an account first
|