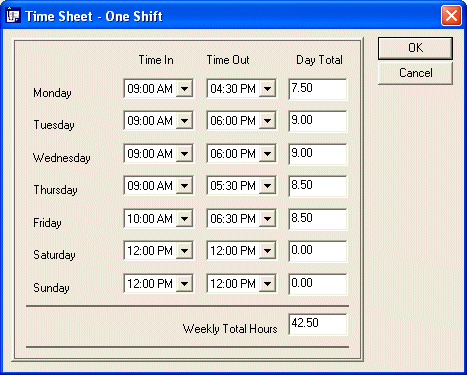 |
A time sheet is a form that employees fill out to indicate how much time they have spent performing a job. Traditional time shift consist of a piece of paper that employees use to mark their time. With the spread of computer applications, many companies now implement a computer-based time sheet where employees enter or select their time.
There are two main ways you can let the users specify their time on a time shift:
- You can let the users type the beginning and end of their shifts, they would have the option of formats such as 8:00AM, 8:00 AM, 8AM, 08: 00AM or 08 AM. They could also use formats such as 2PM, 02PM, 02:00PM, 14:00, 14:00PM or 14:00AM. As you see, some of these formats don’t make sense and some are difficult for you to decipher. Remember that the computer, including the compiler, doesn’t think and cannot make mistakes. This means that it would be your job to imagine all types of scenarios and tell the compiler what you do. For example, you can write an algorithm that would identify 2PM, 02PM, 2:00PM, 02:00 PM, and 14:00 as meaning exactly the same thing. This is not impossible; it’s a job and somebody just has to do it
- You can use a Windows control that would let the user select the time instead of typing it. In this case, you can design the formats or use those of a control. This minimizes, but certainly doesn’t completely eliminate, the possibility of errors.
For this exercise, we will use combo boxes on a time sheet, we will design our own formats and the user will just have to select the time he starts working and select the time he ends his shift.
The simplest computer-based time sheet consists of one shift where the user signs IN and then signs OUT. To implement this, you can design a combo box that has the predefined times. After the user has selected the times, all you have to do is to subtract the beginning of the shift to the end of the shift, get a time between 0 included and 24 excluded, and you are fine.
The application we will write can be implemented in any version of Microsoft Visual Studio or Microsoft Visual C++. The version is not important. |
- Start Microsoft Visual Studio or Microsoft Visual C++.
- On the main menu, click File -> New…
- To create the project, from the New dialog box, click the Projects property sheet
- Click MFC AppWizard (exe)
- In the Project Name edit box, type TSOneShift
and click OK
- In the MFC AppWizard – Step 1, click the Dialog Based radio button and click Next
- In the MFC AppWizard – Step 2 of 4, in the edit box, type Time Sheet – One Shift and click Next
- In the MFC AppWizard – Step 3 of 4, click the No Thanks radio button
and click Next
- In the MFC AppWizard Step 4 of 4, in the classes list, click
CTSOneShiftDlg to select it.
- Change the Class Name to CTimeSheet
- Change the Header File to TimeSheet.cpp
- Change the Implementation File to TimeSheet.cpp
- Click Finish and click OK
|
Designing the Application |
|
- On the dialog box, click the TODO line and press Delete.
- On the Dialog toolbar, click the Toggle Grid button
.
Here is the dialog we are going to design:
Drag the lower right corner of the dialog to get an area of 307 x
211.
-
Move the buttons to the right side of the dialog.
-
From the Toolbox, click the Picture control
and draw a rectangle on the dialog.
-
Right-click the picture rectangle and click Properties.
-
Set the properties of the rectangle to Type = Frame; Styles: Border
= unchecked; Extended Styles: Modal Frame = checked.
-
In the top section of the dialog but inside the frame, add three Static Text controls captioned Time In, Time Out, and Day Total
-
On the left side of the dialog but inside the frame, add 7
vertically aligned Static Text controls captioned Monday, Tuesday, Wednesday, Thursday, Friday, Saturday, and Sunday.
-
From the Toolbox, click the Combo Box control . Under the Time In text, click to add a combo box.
-
Using the Properties dialog, change the ID of the new combo box to IDC_MONDAYIN
-
Click the Data property sheet. In the list box, click and type 00:00 AM and press Ctrl + Enter.
-
Type 00:30 AM and press Ctrl + Enter.
-
In the same way, add the times as follows: 01:00 AM, 01:30 AM, 02:00 AM, 02:30 AM, 03:00 AM, 03:30 AM, 04:00 AM, 04:30 AM, 05:00 AM, 05:30 AM, 06:00 AM, 06:30 AM, 07:00 AM, 07:30 AM, 08:00 AM, 08:30 AM, 09:00 AM, 09:30 AM, 10:00 AM, 10:30 AM, 11:00 AM, 11:30 AM, 12:00 PM, 12:30 PM, 01:00 PM, 01:30 PM, 02:00 PM, 02:30 PM, 03:00 PM, 03:30 PM, 04:00 PM, 04:30 PM, 05:00 PM, 05:30 PM, 06:00 PM, 06:30 PM, 07:00 PM, 07:30 PM, 08:00 PM, 08:30 PM, 09:00 PM, 09:30 PM, 10:00 PM, 10:30 PM, 11:00 PM, and 11:30 PM
-
Click the Style combo box. Change the Type to Drop List and Owner Draw = No,
Sort = unchecked, Vertical Scroll = checked.
-
You should expand the combo box so it can display 6 to 8 items at a time. To do that, on the dialog, click the arrow of the combo box, click the arrow again. You should see a dotted rectangle on the combo box, drag the lower border down.
To preview the combo box, click the Test button on the Dialog toolbar or press Ctrl + T. Click the arrow of the combo box and see how many items the combo box can display at a time. Press Esc to close the dialog. If the combo box didn’t display enough items, you can expand it again.
-
To duplicate the combo box, on the dialog, right-click the Monday In combo box and click Copy.
-
Right-click in the dialog and click Paste.
-
Move the pasted combo box to the right of the previous one. Change its ID to IDC_MONDAYOUT
-
Copy both combo boxes and paste them on the same dialog. Move the newly pasted combo boxes under the other ones.
-
Paste the combo boxes again and again until you get all the desired 14 combo boxes.
32. For the previously created combo boxes and the new ones, set the Ids as follows:
IDC_MONDAYIN, IDC_MONDAYOUT, IDC_TUESDAYIN, IDC_TUESDAYOUT,
IDC_WEDNESDAYIN, IDC_WEDNESDAYOUT, IDC_THURSDAYIN, IDC_THURSDAYOUT,
IDC_FRIDAYIN, IDC_FRIDAYOUT, IDC_SATURDAYIN, IDC_SATURDAYOUT,
IDC_SUNDAYIN, and IDC_SUNDAYOUT.
-
Add a series of Edit Boxes on the right side of each OUT combo box. Set the Ids of the new edit boxes as
IDC_MONDAY, IDC_TUESDAY, IDC_WEDNESDAY, IDC_THURSDAY, IDC_FRIDAY,
IDC_SATURDAY, and IDC_SUNDAY.
-
Add one more Edit Box under the existing ones and set its ID to IDC_TOTAL
-
Embellish your dialog as you see fit and save your application.
|
Programming the Application |
|
- Press Ctrl + W to access the ClassWizard dialog box.
- Click the Member Variables property sheet.
- Double-click each Control ID and create the variables as follows:
- Click OK to close the ClassWizard.
- In the Workspace, click the ClassView property sheet and expand the
TSOneShift Classes folder.
- Expand the CTimeSheet folder
- Double-click the OnInitDialog() function to display it.
- To make sure the combo boxes display default values when the dialog box comes up, change the function as follows:
BOOL CTimeSheet::OnInitDialog()
{
CDialog::OnInitDialog();
// Add "About..." menu item to system menu.
// IDM_ABOUTBOX must be in the system command range.
ASSERT((IDM_ABOUTBOX & 0xFFF0) == IDM_ABOUTBOX);
ASSERT(IDM_ABOUTBOX < 0xF000);
CMenu* pSysMenu = GetSystemMenu(FALSE);
if (pSysMenu != NULL)
{
CString strAboutMenu;
strAboutMenu.LoadString(IDS_ABOUTBOX);
if (!strAboutMenu.IsEmpty())
{
pSysMenu->AppendMenu(MF_SEPARATOR);
pSysMenu->AppendMenu(MF_STRING, IDM_ABOUTBOX, strAboutMenu);
}
}
SetIcon(m_hIcon, TRUE); // Set big icon
SetIcon(m_hIcon, FALSE); // Set small icon
// TODO: Add extra initialization here
// Monday Default Values
m_MondayIn.SetCurSel(18);
m_MondayOut.SetCurSel(34);
// Tuesday Default Values
m_TuesdayIn.SetCurSel(18);
m_TuesdayOut.SetCurSel(34);
// Tuesday Default Values
m_WednesdayIn.SetCurSel(18);
m_WednesdayOut.SetCurSel(34);
// Tuesday Default Values
m_ThursdayIn.SetCurSel(18);
m_ThursdayOut.SetCurSel(34);
// Monday Default Values
m_FridayIn.SetCurSel(18);
m_FridayOut.SetCurSel(34);
// Monday Default Values
m_SaturdayIn.SetCurSel(24);
m_SaturdayOut.SetCurSel(24);
// Monday Default Values
m_SundayIn.SetCurSel(24);
m_SundayOut.SetCurSel(24);
return TRUE; // return TRUE unless you set the focus to a control
}
|
- Assuming that a typical employee works 8 hours a day on weekdays and 0 hour on the weekend, we can display the default values of week days and the total.
Therefore, initialize the edit boxes in the constructor of the
CTimeSheet class as follows:
CTimeSheet::CTimeSheet(CWnd* pParent /*=NULL*/)
: CDialog(CTimeSheet::IDD, pParent)
{
//{{AFX_DATA_INIT(CTimeSheet)
m_Monday = _T("8.00");
m_Tuesday = _T("8.00");
m_Wednesday = _T("8.00");
m_Thursday = _T("8.00");
m_Friday = _T("8.00");
m_Saturday = _T("0.00");
m_Sunday = _T("0.00");
m_Total = _T("40.00");
//}}AFX_DATA_INIT
m_hIcon = AfxGetApp()->LoadIcon(IDR_MAINFRAME);
}
|
- When using the dialog box, the user sets his or her shift by selecting the times from the combo boxes. In order to minimize the time errors, we need to make sure the user doesn’t set a starting shift that occurs after the end shift.
When the user clicks the arrow of a combo box to change its value, an event called OnChange is fired (from the combo box) (and sent to the operating system). Therefore, we must get that event and associate our code to it.
Press Ctrl + W to access the ClassWizard.
- From the Message Maps property sheet, in the Object Ids list, click
IDC_MONDAYIN. In the Messages list, scroll down and double-click
CBN_SELCHANGE.
- Change that name of the function to OnSelChangeMondayIn and click OK
- Click Edit Code and implement the function as follows:
void CTimeSheet::OnSelChangeMondayIn()
{
// InSelection is the time selected on this combo box
int InSelection = m_MondayIn.GetCurSel();
// OutSelection is the time set on the next combo box
int OutSelection = m_MondayOut.GetCurSel();
// Whenever the user decides to change the time on this one,
// make sure the new time doesn't occur after the next time value
// If the user tries to set a starting time that occurs AFTER the
// end time, maybe the user didn't work and wants to set the time to 0
if( InSelection > OutSelection )
m_MondayIn.SetCurSel(OutSelection);
}
|
- In the same way, we must avoid an end time that occurs before the starting time.
Press Ctrl + W to access the ClassWizard
- In the Object Ids list of the Message Maps property sheet,click
IDC_MONDAYOUT.
- In the Messages list, scroll down and double-click CBN_SELCHANGE
- Change the name of the function to OnSelChangeMondayOut and click OK.
- Click Edit Code and implement the function as follows:
void CTimeSheet::OnSelChangeMondayOut()
{
// Get the current starting time from the IN combo box
int InSelection = m_MondayIn.GetCurSel();
// Get the new selection that the user is trying to set
int OutSelection = m_MondayOut.GetCurSel();
// If the user tries to set a time that occurs BEFORE the starting time
// Maybe the user wants the shift to be set to 0
// In that case, set both times to the starting time
if( OutSelection < InSelection )
m_MondayOut.SetCurSel(InSelection);
}
|
- Use the same last two approaches to implement the IN and OUT combo box selections of the other days. When you finish,
their functions should appear as follows:
void CTimeSheet::OnSelChangeTuesdayIn()
{
int InSelection = m_TuesdayIn.GetCurSel();
int OutSelection = m_TuesdayOut.GetCurSel();
if( InSelection > OutSelection )
m_TuesdayIn.SetCurSel(OutSelection);
}
void CTimeSheet::OnSelChangeTuesdayOut()
{
int InSelection = m_TuesdayIn.GetCurSel();
int OutSelection = m_TuesdayOut.GetCurSel();
if( OutSelection < InSelection )
m_TuesdayOut.SetCurSel(InSelection);
}
void CTimeSheet::OnSelChangeWednesdayIn()
{
int InSelection = m_WednesdayIn.GetCurSel();
int OutSelection = m_WednesdayOut.GetCurSel();
if( InSelection > OutSelection )
m_WednesdayIn.SetCurSel(OutSelection);
}
void CTimeSheet::OnSelChangeWednesdayOut()
{
int InSelection = m_WednesdayIn.GetCurSel();
int OutSelection = m_WednesdayOut.GetCurSel();
if( OutSelection < InSelection )
m_WednesdayOut.SetCurSel(InSelection);
}
void CTimeSheet::OnSelChangeThursdayIn()
{
int InSelection = m_ThursdayIn.GetCurSel();
int OutSelection = m_ThursdayOut.GetCurSel();
if( InSelection > OutSelection )
m_ThursdayIn.SetCurSel(OutSelection);
}
void CTimeSheet::OnSelChangeThursdayOut()
{
int InSelection = m_ThursdayIn.GetCurSel();
int OutSelection = m_ThursdayOut.GetCurSel();
if( OutSelection < InSelection )
m_ThursdayOut.SetCurSel(InSelection);
}
void CTimeSheet::OnSelChangeFridayIn()
{
int InSelection = m_FridayIn.GetCurSel();
int OutSelection = m_FridayOut.GetCurSel();
if( InSelection > OutSelection )
m_FridayIn.SetCurSel(OutSelection);
}
void CTimeSheet::OnSelChangeFridayOut()
{
int InSelection = m_FridayIn.GetCurSel();
int OutSelection = m_FridayOut.GetCurSel();
if( OutSelection < InSelection )
m_FridayOut.SetCurSel(InSelection);
}
void CTimeSheet::OnSelChangeSaturdayIn()
{
int InSelection = m_SaturdayIn.GetCurSel();
int OutSelection = m_SaturdayOut.GetCurSel();
if( InSelection > OutSelection )
m_SaturdayIn.SetCurSel(OutSelection);
}
void CTimeSheet::OnSelChangeSaturdayOut()
{
int InSelection = m_SaturdayIn.GetCurSel();
int OutSelection = m_SaturdayOut.GetCurSel();
if( OutSelection < InSelection )
m_SaturdayOut.SetCurSel(InSelection);
}
void CTimeSheet::OnSelChangeSundayIn()
{
int InSelection = m_SundayIn.GetCurSel();
int OutSelection = m_SundayOut.GetCurSel();
if( InSelection > OutSelection )
m_SundayIn.SetCurSel(OutSelection);
}
void CTimeSheet::OnSelChangeSundayOut()
{
int InSelection = m_SundayIn.GetCurSel();
int OutSelection = m_SundayOut.GetCurSel();
if( OutSelection < InSelection )
m_SundayOut.SetCurSel(InSelection);
}
|
- After the user has selected this time, we need to retrieve it and perform some calculation to get the time difference. The time difference will be expressed as a decimal value like 7.50 or 9.00.
The time that is displaying in a combo box is a string. Before performing any calculation, we need to convert this time to decimal value. To do this, we will use a function that can be called
every time the user changes the selection on a combo box.
In the Workspace, right-click the CTimeSheet folder and click Add Member Function…
- Set the Function Type to double and the Function Name to CTimeToDouble(CString Value)
- You should make the function private because we will not have any reason to make it available to external functions. Click OK and implement the function as follows:
double CTimeSheet::CTimeToDouble(CString Value)
{
int HourPortion;
double MinutePortion;
// Get rid of the empty space inside the string
Value.Replace(" ", "");
// Get the hour portion of the string
CString HourValue = Value.Left(2);
// Get the minute portion of the string
CString MinuteValue = Value.Mid(3,2);
// Get the AM PM portion of the string
CString AMPMValue = Value.Right(2);
// We need to take care of the 12 noon issue
// If the time is in the afternoon
if( AMPMValue == "PM" )
{
// Find out if the user selected 12 noon
if( HourValue == "12" )
HourPortion = atoi(HourValue); // Convert it to an integer
else // Otherwise, add 12 to it to convert it to military time
HourPortion = 12 + atoi(HourValue);
}
// But if the time happened in the morning
else
HourPortion = atoi(HourValue);// Simply convert the hour to integer
// Now for the minute portion
// If the minute part of the time selection is 00
if( MinuteValue == "00" )
MinutePortion = 0.00; // then the minute value is 0
else // Otherwise
MinutePortion = 0.50; // The minute part is half
// Add the hour portion and the minute portion
// and return the result, so another function can use it
return HourPortion + MinutePortion;
}
|
- Now that we are able to control the time sequence of the start and end of shifts, we need to display the numeric value of the corresponding day. Whenever the user changes the time display of one of the combo boxes, its value likely changes. We could use a button to calculate the time difference but event-driven programming allows us to catch the change using a common event that many text-based controls share. When the text of a control changes, the control sends an Update event. Normally, we don’t want the user to manually enter the time value in the edit box for each day. The reason we didn’t make the edit boxes Read-Only is because, just in case the dialog box or the combo boxes are not responding (this is unlikely but possible), we can allow the user to edit the time. The supervisor would have the responsibility of checking the shift hourly value.
When a change occurs on a combo box, we will use the Update event to calculate the new time difference and to display the result in the corresponding edit box.
In the private section of the TimeSheet.h file, declare the following functions:
private:
double CTimeToDouble(CString Value);
double UpdateMondayTime();
double UpdateTuesdayTime();
double UpdateWednesdayTime();
double UpdateThursdayTime();
double UpdateFridayTime();
double UpdateSaturdayTime();
double UpdateSundayTime();
};
|
- Implement the functions as follows:
double CTimeSheet::UpdateMondayTime()
{
UpdateData();
double Monday, MondayIn, MondayOut;
CString strMondayIn;
m_MondayIn.GetLBText(m_MondayIn.GetCurSel(), strMondayIn);
MondayIn = CTimeToDouble(strMondayIn);
CString strMondayOut;
m_MondayOut.GetLBText(m_MondayOut.GetCurSel(), strMondayOut);
MondayOut = CTimeToDouble(strMondayOut);
Monday = MondayOut - MondayIn;
m_Monday.Format("%.2f", Monday);
UpdateData(FALSE);
return Monday;
}
double CTimeSheet::UpdateTuesdayTime()
{
UpdateData();
double Tuesday, TuesdayIn, TuesdayOut;
CString strTuesdayIn;
m_TuesdayIn.GetLBText(m_TuesdayIn.GetCurSel(), strTuesdayIn);
TuesdayIn = CTimeToDouble(strTuesdayIn);
CString strTuesdayOut;
m_TuesdayOut.GetLBText(m_TuesdayOut.GetCurSel(), strTuesdayOut);
TuesdayOut = CTimeToDouble(strTuesdayOut);
Tuesday = TuesdayOut - TuesdayIn;
m_Tuesday.Format("%.2f", Tuesday);
UpdateData(FALSE);
return Tuesday;
}
double CTimeSheet::UpdateWednesdayTime()
{
UpdateData();
double Wednesday, WednesdayIn, WednesdayOut;
CString strWednesdayIn;
m_WednesdayIn.GetLBText(m_WednesdayIn.GetCurSel(), strWednesdayIn);
WednesdayIn = CTimeToDouble(strWednesdayIn);
CString strWednesdayOut;
m_WednesdayOut.GetLBText(m_WednesdayOut.GetCurSel(), strWednesdayOut);
WednesdayOut = CTimeToDouble(strWednesdayOut);
Wednesday = WednesdayOut - WednesdayIn;
m_Wednesday.Format("%.2f", Wednesday);
UpdateData(FALSE);
return Wednesday;
}
double CTimeSheet::UpdateThursdayTime()
{
UpdateData();
double Thursday, ThursdayIn, ThursdayOut;
CString strThursdayIn;
m_ThursdayIn.GetLBText(m_ThursdayIn.GetCurSel(), strThursdayIn);
ThursdayIn = CTimeToDouble(strThursdayIn);
CString strThursdayOut;
m_ThursdayOut.GetLBText(m_ThursdayOut.GetCurSel(), strThursdayOut);
ThursdayOut = CTimeToDouble(strThursdayOut);
Thursday = ThursdayOut - ThursdayIn;
m_Thursday.Format("%.2f", Thursday);
UpdateData(FALSE);
return Thursday;
}
double CTimeSheet::UpdateFridayTime()
{
UpdateData();
double Friday, FridayIn, FridayOut;
CString strFridayIn;
m_FridayIn.GetLBText(m_FridayIn.GetCurSel(), strFridayIn);
FridayIn = CTimeToDouble(strFridayIn);
CString strFridayOut;
m_FridayOut.GetLBText(m_FridayOut.GetCurSel(), strFridayOut);
FridayOut = CTimeToDouble(strFridayOut);
Friday = FridayOut - FridayIn;
m_Friday.Format("%.2f", Friday);
UpdateData(FALSE);
return Friday;
}
double CTimeSheet::UpdateSaturdayTime()
{
UpdateData();
double Saturday, SaturdayIn, SaturdayOut;
CString strSaturdayIn;
m_SaturdayIn.GetLBText(m_SaturdayIn.GetCurSel(), strSaturdayIn);
SaturdayIn = CTimeToDouble(strSaturdayIn);
CString strSaturdayOut;
m_SaturdayOut.GetLBText(m_SaturdayOut.GetCurSel(), strSaturdayOut);
SaturdayOut = CTimeToDouble(strSaturdayOut);
Saturday = SaturdayOut - SaturdayIn;
m_Saturday.Format("%.2f", Saturday);
UpdateData(FALSE);
return Saturday;
}
double CTimeSheet::UpdateSundayTime()
{
UpdateData();
double Sunday, SundayIn, SundayOut;
CString strSundayIn;
m_SundayIn.GetLBText(m_SundayIn.GetCurSel(), strSundayIn);
SundayIn = CTimeToDouble(strSundayIn);
CString strSundayOut;
m_SundayOut.GetLBText(m_SundayOut.GetCurSel(), strSundayOut);
SundayOut = CTimeToDouble(strSundayOut);
Sunday = SundayOut - SundayIn;
m_Sunday.Format("%.2f", Sunday);
UpdateData(FALSE);
return Sunday;
}
|
- Now that we can find out how many hours a user has for each day, we can retrieve
the value for each day and add the values to get the total number of hours that the user worked for the week. Since this calculation gives us a total, it is time to display that total in the Total edit box.
In the Workspace, right-click CTimeSheet and click Add member Function…
- Set the function type to void and the name of the function to CalcTotalTime
- You should make the function private. Click OK and implement the function as follows:
void CTimeSheet::CalcTotalTime()
{
double Mon, Tue, Wed, Thu, Fri, Sat, Sun, Total;
UpdateData();
Mon = UpdateMondayTime();
Tue = UpdateTuesdayTime();
Wed = UpdateWednesdayTime();
Thu = UpdateThursdayTime();
Fri = UpdateFridayTime();
Sat = UpdateSaturdayTime();
Sun = UpdateSundayTime();
Total = Mon + Tue + Wed + Thu + Fri + Sat + Sun;
m_Total.Format("%.2f", Total);
UpdateData(FALSE);
}
|
- If you test the program now, everything works fine but you would hardly notice because the combo boxes don’t have a mechanism to send their results to the Total edit box. Therefore, the last thing we need to do now is to use the effect that occurs when the value of a combo box has changed. To do this, access the
OnSelChange… functions we created earlier and at the end of each, call the
CalcTotalTime() function. When you have finished, the functions should look as follows:
void CTimeSheet::OnSelChangeMondayIn()
{
// InSelection is the time selected on this combo box
int InSelection = m_MondayIn.GetCurSel();
// OutSelection is the time set on the next combo box
int OutSelection = m_MondayOut.GetCurSel();
// Whenever the user decides to change the time on this one,
// make sure the new time doesn't occur after the next time value
// If the user tries to set a starting time that occurs AFTER the
// end time, maybe the user didn't work and wants to set the time to 0
if( InSelection > OutSelection )
m_MondayIn.SetCurSel(OutSelection);
CalcTotalTime();
}
void CTimeSheet::OnSelChangeMondayOut()
{
...
CalcTotalTime();
}
void CTimeSheet::OnSelChangeTuesdayIn()
{
...
CalcTotalTime();
}
void CTimeSheet::OnSelChangeTuesdayOut()
{
...
CalcTotalTime();
}
void CTimeSheet::OnSelChangeWednesdayIn()
{
...
CalcTotalTime();
}
void CTimeSheet::OnSelChangeWednesdayOut()
{
...
CalcTotalTime();
}
void CTimeSheet::OnSelChangeThursdayIn()
{
...
CalcTotalTime();
}
void CTimeSheet::OnSelChangeThursdayOut()
{
...
CalcTotalTime();
}
void CTimeSheet::OnSelChangeFridayIn()
{
...
CalcTotalTime();
}
void CTimeSheet::OnSelChangeFridayOut()
{
...
CalcTotalTime();
}
void CTimeSheet::OnSelChangeSaturdayIn()
{
...
CalcTotalTime();
}
void CTimeSheet::OnSelChangeSaturdayOut()
{
...
CalcTotalTime();
}
void CTimeSheet::OnSelChangeSundayIn()
{
...
CalcTotalTime();
}
void CTimeSheet::OnSelChangeSundayOut()
{
...
CalcTotalTime();
}
|
- Test your program.
Program
|
|
|