Introduction to Radio Buttons
|
|
|
A radio button is a control that appears as a
(proportionately big) dot surrounded by a round box
.
In reality, a radio button is accompanied by one or more other radio buttons
that appear and behave as a group. The user decides which button is valid by
selecting only one of them. When a radion button gets clicked, its round box
fills with a (big) dot:
.
|
When one button is selected, the other round buttons
of the (same) group are empty
.
The user can select another by clicking a new choice, which empties the
previous selection. Here is an example from the Microsoft Windows 95 Shut
Down dialog box:

This technique of selecting is referred to as
mutually-exclusive.
To indicate what a radio butto is used for, it may be
accompanied by static text. That text is simply a string and it can be
positioned to the left, above, to the right, or under the round box.
Practical
Learning: Introducing Radio Buttons
|
|
- Start Microsoft Visual Studio
- To start a new project, on the main menu, click File -> New
Project...
- In the middle list, click MFC Application and set the name to
Operations
- Click OK
- In the first page of the MFC Application Wizard, click Next
- In the second page of the wizard, click Dialog Based and click
Next
- In the third page of the wizard, click Finish
Creating Radio ButtonsCreating Radio Buttons
|
|
Radio buttons usually come in a group. Based on this,
before creating radio buttons, you should first add a group box (or any
appropriate container) to a dialog box (or to the object that will host
the radio buttons; an example would be a Picture frame).
To visually create a radio button, on the Toolbox,
click the Radio Button object and click the desired area on the host
window. In the same way, add one or more radio buttons.
As a Windows control, the radio button is based on the
CButton class which, like all other constrols, is based
on CWnd. As far as the Win32 and the MFC libraries are
concerned, a radio button is first of all, a button. Therefore, to
programmatically create a radio button, declare a variable or a pointer to
CButton using its default constructor. Like all other MFC
controls, the CButton class provides a Create()
member function to initialize it. The syntax of this member function for a
button is:
BOOL Create(LPCTSTR lpszCaption,
DWORD dwStyle,
const RECT& rect,
CWnd* pParentWnd,
UINT nID);
The lpszCaption argument is the string of the
label that accomponies the radio button. The differences of radio buttons
are set using the dwStyle argument. A radio button must have the
BS_RADIOBUTTON value.
When setting the rect argument, provide
enough space for the control because, by default, it will be confined to
that rectangle and cannot display beyond that. Here are two examples:
BOOL CExerciseDlg::OnInitDialog()
{
CDialog::OnInitDialog();
// Set the icon for this dialog. The framework does this automatically
// when the application's main window is not a dialog
SetIcon(m_hIcon, TRUE); // Set big icon
SetIcon(m_hIcon, FALSE); // Set small icon
// TODO: Add extra initialization here
CButton *rdoSmall = new CButton;
CButton *rdoMedium = new CButton;
rdoSmall->Create(Something,
WS_CHILD | WS_VISIBLE | BS_RADIOBUTTON,
CRect(130, 40, 200, 50), this, 0x12);
rdoMedium->Create(Something,
WS_CHILD | WS_VISIBLE | BS_RADIOBUTTON,
CRect(130, 70, 200, 80), this, 0x14);
return TRUE; // return TRUE unless you set the focus to a control
}
Practical
Learning: Creating Radio Buttons
|
|
- Design the dialog box as follows:
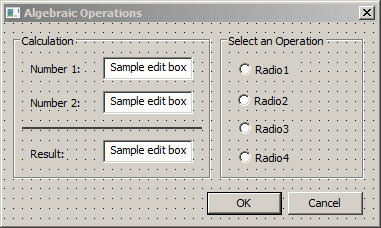 |
Control |
Caption |
ID |
Align Text |
Group Box |
 |
Calculation |
|
|
Static Text |
 |
Number &1: |
|
|
Edit Control |
 |
|
IDC_OPERAND1 |
Right |
Static Text |
 |
Number &2: |
|
|
Edit Control |
 |
|
IDC_OPERAND2 |
Right |
Picture |
|
|
|
|
Static Control |
 |
Result: |
|
|
Edit Control |
 |
|
IDC_RESULT |
Right |
Group Box |
 |
Select an Operation |
|
|
Radio Button |
 |
|
|
|
Radio Button |
 |
|
|
|
Radio Button |
 |
|
|
|
Radio Button |
 |
|
|
|
|
- Right-click each of the edit controls and click Add Variable...
Then create the variables as follows:
ID |
Category |
Type |
Name |
IDC_OPERAND1 |
Value |
double |
m_Operand1 |
IDC_OPERAND2 |
Value |
double |
m_Operand2 |
IDC_RESULT |
Value |
double |
m_Result |
Primary Characteristics of Radio Buttons
|
|
.
The Caption of a Radio Button
|
|
The text a radio button displays is referred to as its
caption. That text should be explicit enough for the user to figure out
the role of an item in the group. To visually specify the caption of a
radio button, after adding the control, replace the value of the Caption
field in the Properties window. If you are programmatically creating the
control, pass its caption as the first argument. Here are examples:
BOOL CExerciseDlg::OnInitDialog()
{
CDialog::OnInitDialog();
// Set the icon for this dialog. The framework does this automatically
// when the application's main window is not a dialog
SetIcon(m_hIcon, TRUE); // Set big icon
SetIcon(m_hIcon, FALSE); // Set small icon
// TODO: Add extra initialization here
CButton *rdoSmall = new CButton;
CButton *rdoMedium = new CButton;
rdoSmall->Create(_T("Small"),
WS_CHILD | WS_VISIBLE | BS_RADIOBUTTON,
CRect(130, 40, 200, 50), this, 0x12);
rdoMedium->Create(_T("Medium"),
WS_CHILD | WS_VISIBLE | BS_RADIOBUTTON,
CRect(130, 70, 200, 80), this, 0x14);
return TRUE; // return TRUE unless you set the focus to a control
}
If the control exists already, to programmaticalyl
change its caption, call the CWnd::SetWindowText() member
function.
Practical
Learning: Setting the Captions of Radio Buttons
|
|
- On the dialog box, click the first radio button
- In the Properties window, click Caption and type &Addition
- Change the captions of the other radio buttons as follows:
- Design the dialog box as follows:
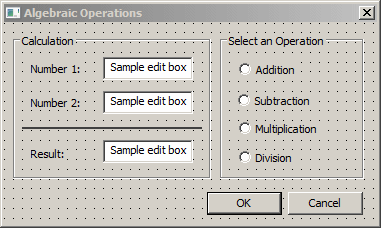 |
Control |
Caption |
ID |
Group Box |
 |
Select an Operation |
|
Radio Button |
 |
&Addition |
IDC_ADDITION |
Radio Button |
 |
&Subtraction |
IDC_SUBTRACTION |
Radio Button |
 |
&Multiplication |
IDC_MULTIPLICATION |
Radio Button |
 |
&Division |
IDC_DIVISION |
|
The Selected Radio Button
|
|
As described already, to select a radio button, the
user can click it. To indicate to the user that a button has been
selected, it should display a dot in its round box. To visually allow the
user to automatically display its dot, in the Properties window, set the
Auto property to True. To programmatically allow the user to select a
radio button, when creating it, add the BS_AUTORADIOBUTTON
style. Here are examples:
int CExerciseDlg::OnCreate(LPCREATESTRUCT lpCreateStruct)
{
if (CDialogEx::OnCreate(lpCreateStruct) == -1)
return -1;
// TODO: Add your specialized creation code here
CWnd *ctlGroupBox = new CWnd;
CButton *rdoSmall = new CButton;
CButton *rdoMedium = new CButton;
CButton *rdoLarge = new CButton;
ctlGroupBox->Create(_T("BUTTON"),
_T("Pizza Size"),
WS_CHILD | WS_VISIBLE | BS_GROUPBOX,
CRect(10, 10, 200, 160),
this,
IDC_PIZZASIZE);
rdoSmall->Create(_T("Small"),
WS_CHILD | WS_VISIBLE | BS_AUTORADIOBUTTON,
CRect(40, 40, 120, 60), this, 0x12);
rdoMedium->Create(_T("Medium"),
WS_CHILD | WS_VISIBLE | BS_AUTORADIOBUTTON,
CRect(40, 70, 120, 100), this, 0x14);
rdoLarge->Create(_T("Large"),
WS_CHILD | WS_VISIBLE | BS_AUTORADIOBUTTON,
CRect(40, 100, 120, 140), this, 0x16);
return 0;
}
While using the radio buttons, the user is presented
with the controls and requested to select one. To make a selection, the
user must click the desired choice. The control selected fills its circle
with a (big) dot. To programmatically change the state of a radio button,
you have various options.
The easiest way to select a radio button consists of
calling the CWnd::CheckRadioButton() member function
(actually, you should call this member function as
CDialog::CheckRadioButton()). Its syntax is:
void CheckRadioButton(int nIDFirstButton,
int nIDLastButton,
int nIDCheckButton);
To use this member function, you must know the
identifiers of the radio buttons involved. The nIDFirstButton is
the identifier of the first radio button of the group. The nIDLastButton
is the identifier of the last radio button of the group. The last
argument, nIDCheckButton is the identifier of the radio button that must
be selected. Here is an example:
void CExerciseDlg::OnBtnSelect2()
{
// TODO: Add your control notification handler code here
CheckRadioButton(IDC_RADIO1, IDC_RADIO3, IDC_RADIO3);
}
To change the state of a radio button, you can call
the CButton::SetCheck() member function. Its syntax is:
void SetCheck(int nCheck);
The nCheck value indicates how to fill the
control's circle. To fill it with the selection dot, pass the value of 0.
To deselect a radio button, pass the argument as 1. Here is an example:
void CExerciseDlg::OnBtnSelect2()
{
// TODO: Add your control notification handler code here
CButton *btnSecond;
btnSecond = reinterpret_cast<CButton *>(GetDlgItem(IDC_RADIO2));
btnSecond->SetCheck(1);
}
To find out what radio button of a group is selected,
you can call the CWnd::GetCheckedRadioButton(). Its syntax is:
int GetCheckedRadioButton( int nIDFirstButton, int nIDLastButton );
When calling this member function, you must specify
the identifier of the first radio button of the group as
nIDFirstButton and the identifier of the last radio button of the
group as nIDLastButton. The member function returns the
identifier of the radio button whose circle is filled.
Alternatively, to find the selected radio button, call
the CButton::GetCheck() member function. Its syntax is:
int GetCheck( ) const;
This member function returns 0 if the radio button is
not selected. Otherwise, it returns 1 if the radio button is selected.
If you want to want to find whether a particular radio
button is currently selected, you can call the
CWnd::IsDlgButtonChecked() member function. Its syntax:
UINT IsDlgButtonChecked(int nIDButton) const;
When calling this member function, pass the identifier
of the radio button as the nIDButton argument. This member function will
check the state of the radio button. If the radio button is selected, the
member function will return 1. If the radio button is not selected, the
member function returns 0.
Because a radio is first of all a control of
CButton type, when it is clicked, it generates the
BN_CLICKED message. You can use this message to take action in
response to the user's.
We have mentioned already that a control can have two
types of variables: Control or Value. For a radio button, you can declare
or add either a CButton or a regular C++ variable. If you
decide to use a CButton variable, you can call the
necessary member function to take advantage of the class. On the other
hand, if you want to use a value instead, you can add a variable for only
the first radio button of the group, the radio button that has the Group
property set to true. The variable should be an integer, such as
int. In such a case, each radio button of the group can be
referred to by a number. The first radio button of the group would have
the index 0, the second would be 1, etc.
Here is an example:
Header File
// ExerciseDlg.h : header file
//
#pragma once
// CExerciseDlg dialog
class CExerciseDlg : public CDialogEx
{
// Construction
public:
CExerciseDlg(CWnd* pParent = NULL); // standard constructor
// Dialog Data
enum { IDD = IDD_CONTROLS_DIALOG };
protected:
virtual void DoDataExchange(CDataExchange* pDX); // DDX/DDV support
// Implementation
protected:
HICON m_hIcon;
// Generated message map functions
virtual BOOL OnInitDialog();
afx_msg void OnPaint();
afx_msg HCURSOR OnQueryDragIcon();
DECLARE_MESSAGE_MAP()
public:
int m_Gender;
CString m_Value;
afx_msg void OnBnClickedMale();
afx_msg void OnBnClickedFemale();
afx_msg void OnBnClickedDontKnow();
};
Source File
Let the// ExerciseDlg.cpp : implementation file
//
#include "stdafx.h"
#include "Controls.h"
#include "ExerciseDlg.h"
#include "afxdialogex.h"
#ifdef _DEBUG
#define new DEBUG_NEW
#endif
// CExerciseDlg dialog
CExerciseDlg::CExerciseDlg(CWnd* pParent /*=NULL*/)
: CDialogEx(CExerciseDlg::IDD, pParent), m_Gender(0), m_Value(_T(""))
{
m_hIcon = AfxGetApp()->LoadIcon(IDR_MAINFRAME);
}
void CExerciseDlg::DoDataExchange(CDataExchange* pDX)
{
CDialogEx::DoDataExchange(pDX);
DDX_Text(pDX, IDC_VALUE, m_Value);
}
BEGIN_MESSAGE_MAP(CExerciseDlg, CDialogEx)
ON_WM_PAINT()
ON_WM_QUERYDRAGICON()
ON_BN_CLICKED(IDC_MALE, &CExerciseDlg::OnBnClickedMale)
ON_BN_CLICKED(IDC_FEMALE, &CExerciseDlg::OnBnClickedFemale)
ON_BN_CLICKED(IDC_DONT_KNOW, &CExerciseDlg::OnBnClickedDontKnow)
END_MESSAGE_MAP()
// CExerciseDlg message handlers
BOOL CExerciseDlg::OnInitDialog()
{
CDialogEx::OnInitDialog();
// Set the icon for this dialog. The framework does this automatically
// when the application's main window is not a dialog
SetIcon(m_hIcon, TRUE); // Set big icon
SetIcon(m_hIcon, FALSE); // Set small icon
// TODO: Add extra initialization here
return TRUE; // return TRUE unless you set the focus to a control
}
// If you add a minimize button to your dialog, you will need the code below
// to draw the icon. For MFC applications using the document/view model,
// this is automatically done for you by the framework.
void CExerciseDlg::OnPaint()
{
if (IsIconic())
{
CPaintDC dc(this); // device context for painting
SendMessage(WM_ICONERASEBKGND,
reinterpret_cast<WPARAM>(dc.GetSafeHdc()), 0);
// Center icon in client rectangle
int cxIcon = GetSystemMetrics(SM_CXICON);
int cyIcon = GetSystemMetrics(SM_CYICON);
CRect rect;
GetClientRect(&rect);
int x = (rect.Width() - cxIcon + 1) / 2;
int y = (rect.Height() - cyIcon + 1) / 2;
// Draw the icon
dc.DrawIcon(x, y, m_hIcon);
}
else
{
CDialogEx::OnPaint();
}
}
// The system calls this function to obtain the cursor to display while the user drags
// the minimized window.
HCURSOR CExerciseDlg::OnQueryDragIcon()
{
return static_cast<HCURSOR>(m_hIcon);
}
void CExerciseDlg::OnBnClickedMale()
{
// TODO: Add your control notification handler code here
m_Value = _T("Man");
UpdateData(FALSE);
}
void CExerciseDlg::OnBnClickedFemale()
{
// TODO: Add your control notification handler code here
m_Value = _T("Woman");
UpdateData(FALSE);
}
void CExerciseDlg::OnBnClickedDontKnow()
{
// TODO: Add your control notification handler code here
m_Value = _T("Unknown");
UpdateData(FALSE);
}
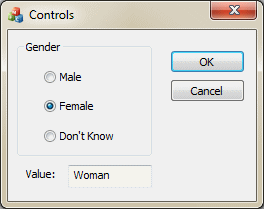
Practical
Learning: Selecting a Radio Button
|
|
- On the dialog box, double-click the Addition radio button
- Implement its event as follows:
void COperationsDlg::OnBnClickedAddition()
{
// TODO: Add your control notification handler code here
UpdateData();
m_Result = m_Operand1 + m_Operand2;
UpdateData(FALSE);
}
- Return to the dialog box and double-click the Subtraction radio
button
- Implement its event as follows:
void COperationsDlg::OnBnClickedSubtraction()
{
// TODO: Add your control notification handler code here
UpdateData();
m_Result = m_Operand1 - m_Operand2;
UpdateData(FALSE);
}
- Return to the dialog box and double-click the Multiplication radio
button
- Implement its event as follows:
void COperationsDlg::OnBnClickedMultiplication()
{
// TODO: Add your control notification handler code here
UpdateData();
m_Result = m_Operand1 * m_Operand2;
UpdateData(FALSE);
}
- Return to the dialog box and double-click the Division radio
button
- Implement its event as follows:
void COperationsDlg::OnBnClickedDivision()
{
// TODO: Add your control notification handler code here
UpdateData();
m_Result = m_Operand1 / m_Operand2;
UpdateData(FALSE);
}
- To execute the application to test the radio buttons, press F5
- Enter some values in the edit boxes and click the radio buttons
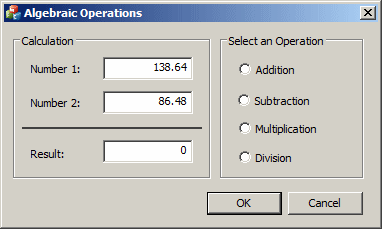
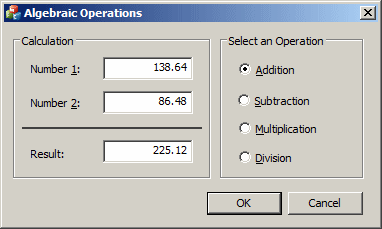
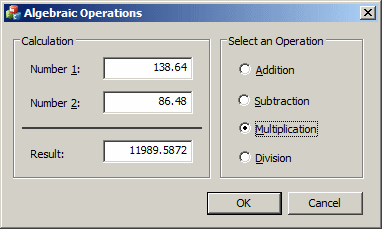
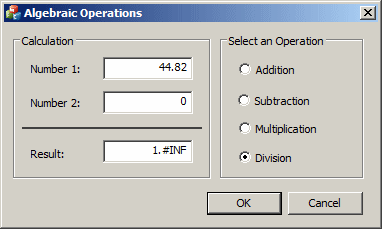
- Close the dialog box and return to your programming environment
Separating Radio Buttons in Groups
|
|
For either the user or the programmer, radio buttons
must behave as entities of one group. When you freshly add radio buttons
to a host, they are created as a group. If your dialog box will be made of
only a few radio buttons, you may not need to do much. Imagine you want to
create a dialog box with more than one set of radio buttons. In this case,
you must separate them into groups. To do that, set the Group property of
each new button of a group to True. To do this programmatically, add the
WS_GROUP style to the CButton object.
Imagine you will use two sets of group buttons in a
dialog box. Add the first radio button and set its Group property to True
or add the WS_GROUP value to its style. Then, add the
other radio buttons of the set but set their Group property to False or do
not add the WS_GROUP value to their styles.
When starting the new set, add a new radio button and
set its Group property to True. Add the other radio buttons with the Group
property set to False or without the WS_GROUP style.
Practical
Learning: Selecting Radio Buttons
|
|
- To start a new application, on the main menu, click File -> New
Project...
- In the middle list, click MFC Application and set the name to
ClarksvilleIceScream1
- Press Enter
- In the first page of the MFC Application Wizard, click Next
- In the second page, click Dialog Based and click Next
- Click Finish
- Design the dialog box as follows:
 |
Control |
Caption |
Group Box |
 |
Flavors |
Radio Button |
 |
Vanilla |
Radio Button |
 |
Cream of Cocoa |
Radio Button |
 |
Chocolate Chip |
Radio Button |
 |
Butter Pecan |
Radio Button |
 |
Chunky Butter |
Radio Button |
 |
Strawberry Vanilla |
Radio Button |
 |
Chocolate Cookies |
Group Box |
 |
Containers |
Radio Button |
 |
Cup |
Radio Button |
 |
Cone |
Radio Button |
 |
Bowl |
Group Box |
 |
Ingredients |
Radio Button |
 |
None |
Radio Button |
 |
M && M |
Radio Button |
 |
Mixed Nuts |
Group Box |
 |
Scoops |
Radio Button |
 |
One |
Radio Button |
 |
Two |
Radio Button |
 |
Three |
|
- To execute the application, press F5
- Click the radio buttons from different group boxes and notice that
all radio buttons seem to belong to the same group
- Close the dialog box and return to your programming environment
- On the dialog box, click the Vanilla radio button
- In the Properties window, double-click Group to set its value to
True
- On the dialog box, click the Cup radio button
- In the Properties window, double-click Group to set its value to
True
- On the dialog box, click the None radio button
- In the Properties window, double-click Group to set its value to
True
- On the dialog box, click the One radio button
- In the Properties window, double-click Group to set its value to
True
- Press F5 to execute the application
- Click the radio buttons in different sections
- Close the dialog box and return to your programming environment