Practical
Learning: Using the Outlook Bar
|
|
- In the Resource View, right-click LifePlanning -> Add -> Resource...
- In the Add Resource dialog box, click Bitmap and click New
- In the Properties window, change the following values:
Color: 24
bit Height: 32 Width: 96
- In the Resource View, click IDB_BITMAP1
- In the Properties window, click ID, type IDB_OUTLOOK
and press Enter
- Design the bitmap as follows:

- Access the LifePlanningSheet.h file and declare a CImageList
variable named m_OutlookImages:
#pragma once
#include "ComputerScience.h"
#include "InformationTechnology.h"
#include "History.h"
#include "CulinaryServices.h"
#include "Careers.h"
// CLifePlanningSheet
class CLifePlanningSheet : public CMFCPropertySheet
{
DECLARE_DYNAMIC(CLifePlanningSheet)
public:
CLifePlanningSheet();
virtual ~CLifePlanningSheet();
private:
CComputerScience pgeComputerScience;
CInformationTechnology pgeInformationTechnology;
CHistory pgeHistory;
CCulinaryServices pgeCulinaryServices;
CCareers pgeCareers;
CImageList m_OutlookImages;
protected:
DECLARE_MESSAGE_MAP()
};
- Access the LifePlanningSheet.cpp file and change it as follows:
CLifePlanningSheet::CLifePlanningSheet()
{
// 1. Classic property sheet
/* AddPage(&pgeComputerScience);
AddPage(&pgeInformationTechnology);
SetLook(CMFCPropertySheet::PropSheetLook_Tabs); */
// 2. Microsoft OneNote tabs
/* AddPage(&pgeComputerScience);
AddPage(&pgeInformationTechnology);
AddPage(&pgeHistory);
AddPage(&pgeCulinaryServices);
SetLook(CMFCPropertySheet::PropSheetLook_OneNoteTabs); */
// 3. Outlook Bar
AddPage(&pgeComputerScience);
AddPage(&pgeHistory);
AddPage(&pgeCulinaryServices);
m_OutlookImages.Create(IDB_OUTLOOK, 32, 1, RGB(128, 128, 128));
SetIconsList(m_OutlookImages);
SetLook(CMFCPropertySheet::PropSheetLook_OutlookBar);
}
- To execute, press F5
- Click the Culinary Services icon
- Position the mouse on Computer Science
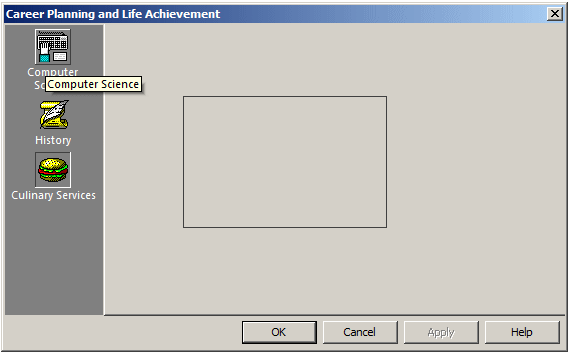
- Close the property sheet
- Close the dialog box and return to your programming environment
Instead of using tabs or buttons, you can create strings
that each would behave like a link. The user can create a label to access a
property page. To get this behavior, call the SetLook()
member function and apply the PropSheetLook_List option.
As seen for the Outlook bar, if you use a list of
strings, there would be a bar on the left side of the property sheet and
that bar would be equipped with the strings.
Practical
Learning: Using a List
|
|
- Change the LifePlanningSheet.cpp file it as follows:
CLifePlanningSheet::CLifePlanningSheet()
{
// 1. Classic property sheet
/* AddPage(&pgeComputerScience);
AddPage(&pgeInformationTechnology);
SetLook(CMFCPropertySheet::PropSheetLook_Tabs); */
// 2. Microsoft OneNote tabs
/* AddPage(&pgeComputerScience);
AddPage(&pgeInformationTechnology);
AddPage(&pgeHistory);
AddPage(&pgeCulinaryServices);
SetLook(CMFCPropertySheet::PropSheetLook_OneNoteTabs); */
// 3. Outlook Bar
/* AddPage(&pgeComputerScience);
AddPage(&pgeHistory);
AddPage(&pgeCulinaryServices);
m_OutlookImages.Create(IDB_OUTLOOK, 32, 1, RGB(128, 128, 128));
SetIconsList(m_OutlookImages);
SetLook(CMFCPropertySheet::PropSheetLook_OutlookBar); */
// 4. List
AddPage(&pgeComputerScience);
AddPage(&pgeInformationTechnology);
AddPage(&pgeCulinaryServices);
SetLook(CMFCPropertySheet::PropSheetLook_List, 150);
}
- To execute, press F5
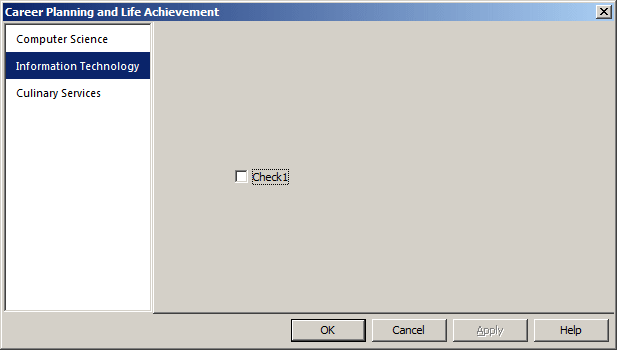
- Close the property sheet
- Close the dialog box and return to your programming environment
Using a Tree List of Nodes
|
|
One of the characteristics of a list of labels is that
each string is independent of the others. As alternative, you can create a
tree of labels so that it would show that some labels belong to a group, and
consequently some property pages work together. The labels in this case are
called nodes.
If you use a tree list, the property sheet displays a
bar on the left side. The list on that side can have strings like those of
the previously seen list. To enhance it, you can create one or more nodes
that behave as parents to other nodes. When a node is used as a parent, if
the user clicks it, it expands to show its child nodes. If the user clicks
that parent node again, it collapses to hid its child nodes. In all cases,
if the user clicks a node on the left side, its property page displays on
the right side.
To get a tree list, first call the SetLook()
member function and apply the CMFCPropertySheet::PropSheetLook_Tree.
If you stop there, the property sheet would behave like that of the list of
labels. If you want an actual list, you must create. Fortunately, it is easy
as long as you follow the right steps.
To let you create a tree list, the
CMFCPropertySheet class is equipped with the
AddTreeCategory() member function. Its syntax is:
CMFCPropertySheetCategoryInfo* AddTreeCategory(
LPCTSTR lpszLabel,
int nIconNum = -1,
int nSelectedIconNum = -1,
const CMFCPropertySheetCategoryInfo* pParentCategory = NULL
);
When calling this function get a reference to the
CMFCPropertySheetCategoryInfo object it returns. This function takes
four argument but only one is required. The first argument is the string of
the parent node. You can pass just that argument and the property sheet
would work fine. If you want a node to display an icon, pass the zero-based
index of that icon as the second argument. In this case, you can also pass a
third argument as the icon that would display when the parent node is
selected. If the node you are currently creating is the top node, pass the
third argument as NULL. If that node has its own parent, pass its reference
as the fourth argument.
After calling this member function, call the the
AddPageToTree() member function. Its syntax is:
void AddPageToTree(
CMFCPropertySheetCategoryInfo* pCategory,
CMFCPropertyPage* pPage,
int nIconNum=-1,
int nSelIconNum=-1
);
The first argument is a reference to a parent node you
must have previously created. The second argument is a reference to a
property page, the exact same argument you would pass for a regular property
page in a Win32 property sheet. If the node should display an icon, pass the
optional third argument. Also, you can optionally pass the fourth argument
to specify what icon the node should display when it is selected.
Practical
Learning: Using a Tree List
|
|
- Change the LifePlanningSheet.cpp file it as follows:
CLifePlanningSheet::CLifePlanningSheet()
{
// 1. Classic property sheet
/* AddPage(&pgeComputerScience);
AddPage(&pgeInformationTechnology);
SetLook(CMFCPropertySheet::PropSheetLook_Tabs); */
// 2. Microsoft OneNote tabs
/* AddPage(&pgeComputerScience);
AddPage(&pgeInformationTechnology);
AddPage(&pgeHistory);
AddPage(&pgeCulinaryServices);
SetLook(CMFCPropertySheet::PropSheetLook_OneNoteTabs); */
// 3. Outlook Bar
/* AddPage(&pgeComputerScience);
AddPage(&pgeHistory);
AddPage(&pgeCulinaryServices);
m_OutlookImages.Create(IDB_OUTLOOK, 32, 1, RGB(128, 128, 128));
SetIconsList(m_OutlookImages);
SetLook(CMFCPropertySheet::PropSheetLook_OutlookBar); */
// 4. List
/* AddPage(&pgeComputerScience);
AddPage(&pgeInformationTechnology);
AddPage(&pgeCulinaryServices);
SetLook(CMFCPropertySheet::PropSheetLook_List, 150); */
// 5. Tree List
SetLook(CMFCPropertySheet::PropSheetLook_Tree, 150);
CMFCPropertySheetCategoryInfo * pscComputers = AddTreeCategory(_T("Computers"));
AddPageToTree(pscComputers, &pgeComputerScience);
AddPageToTree(pscComputers, &pgeInformationTechnology);
AddPage(&pgeHistory);
AddPage(&pgeCulinaryServices);
}
- To execute, press F5
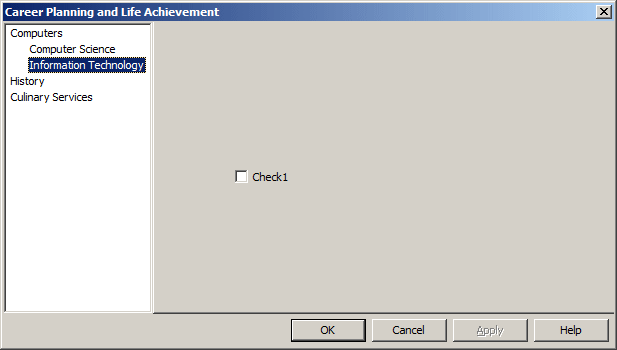
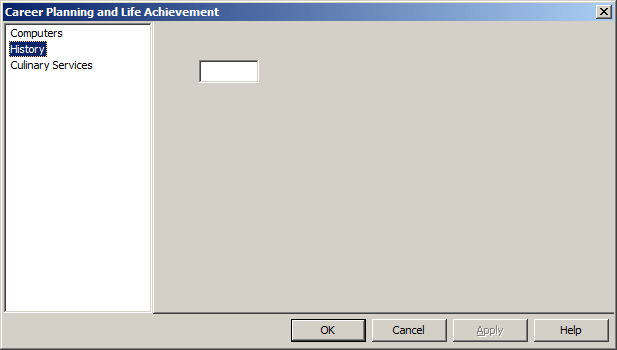
- Close the property sheet
- Close the dialog box and return to your programming environment
- In the Resource View, right-click LifePlanning -> Add -> Resource...
- In the Add Resource dialog box, click Bitmap and click New
- In the Properties window, change the following values:
Color: 24
bit Height: 16 Width: 64
- In the Resource View, click IDB_BITMAP2
- In the Properties window, click ID, type IDB_TREELIST
and press Enter
- Design the bitmap as follows:

- Access the LifePlanningSheet.h file and declare a CImageList
variable named m_OutlookImages:
#pragma once
#include "ComputerScience.h"
#include "InformationTechnology.h"
#include "History.h"
#include "CulinaryServices.h"
#include "Careers.h"
// CLifePlanningSheet
class CLifePlanningSheet : public CMFCPropertySheet
{
DECLARE_DYNAMIC(CLifePlanningSheet)
public:
CLifePlanningSheet();
virtual ~CLifePlanningSheet();
private:
CComputerScience pgeComputerScience;
CInformationTechnology pgeInformationTechnology;
CHistory pgeHistory;
CCulinaryServices pgeCulinaryServices;
CCareers pgeCareers;
CImageList m_OutlookImages;
CImageList m_TreeListImages;
protected:
DECLARE_MESSAGE_MAP()
};
- Access the LifePlanningSheet.cpp file and change it as follows:
CLifePlanningSheet::CLifePlanningSheet()
{
// 1. Classic property sheet
/* AddPage(&pgeComputerScience);
AddPage(&pgeInformationTechnology);
SetLook(CMFCPropertySheet::PropSheetLook_Tabs); */
// 2. Microsoft OneNote tabs
/* AddPage(&pgeComputerScience);
AddPage(&pgeInformationTechnology);
AddPage(&pgeHistory);
AddPage(&pgeCulinaryServices);
SetLook(CMFCPropertySheet::PropSheetLook_OneNoteTabs); */
// 3. Outlook Bar
/* AddPage(&pgeComputerScience);
AddPage(&pgeHistory);
AddPage(&pgeCulinaryServices);
m_OutlookImages.Create(IDB_OUTLOOK, 32, 1, RGB(128, 128, 128));
SetIconsList(m_OutlookImages);
SetLook(CMFCPropertySheet::PropSheetLook_OutlookBar); */
// 4. List
/* AddPage(&pgeComputerScience);
AddPage(&pgeInformationTechnology);
AddPage(&pgeCulinaryServices);
SetLook(CMFCPropertySheet::PropSheetLook_List, 150); */
// 5. Tree List
// Add the icons to the image list
m_TreeListImages.Create(IDB_TREELIST, 16, 1, RGB(255, 255, 255));
// Indicate that the tree list will use icons
SetIconsList(m_TreeListImages);
// Indicate that the property sheet will use a tree list
SetLook(CMFCPropertySheet::PropSheetLook_Tree, 185);
// Create the top node and name it College Majors
CMFCPropertySheetCategoryInfo * pscCollgeMajors =
AddTreeCategory(_T("College Majors"), 0, 1);
// Under the College Majors node, create a category named Computers Related
CMFCPropertySheetCategoryInfo * pscComputers =
AddTreeCategory(_T("Computers Related"), 2, 3, pscCollgeMajors);
// Add the pages to the category
AddPageToTree(pscComputers, &pgeComputerScience);
AddPageToTree(pscComputers, &pgeInformationTechnology);
// Add two categories to the College Majors node
AddPageToTree(pscCollgeMajors, &pgeHistory);
AddPageToTree(pscCollgeMajors, &pgeCulinaryServices);
// Add a node that doesn't have a parent
AddPage(&pgeCareers);
}
- To execute, press F5
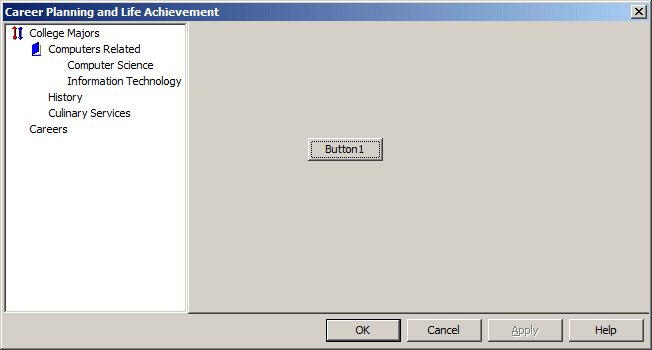
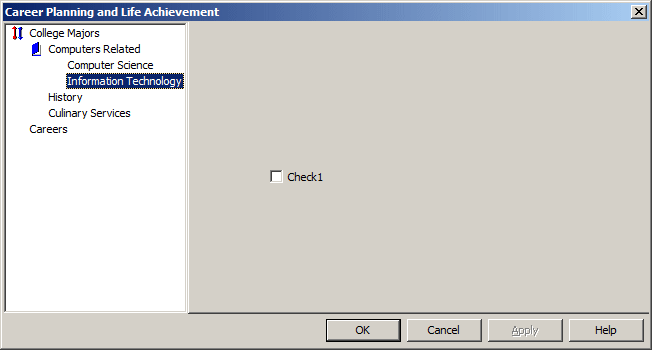
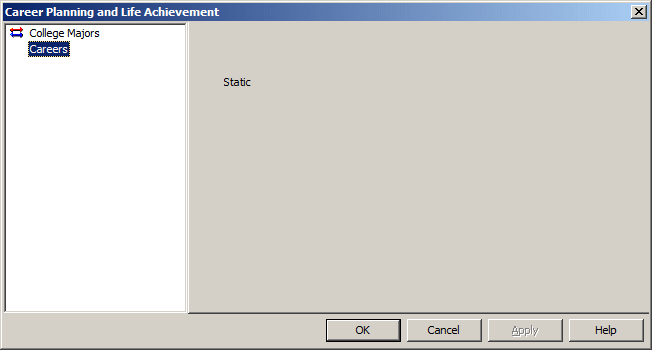
- Close the property sheet
- Close the dialog box and return to your programming environment
- Change the design of the bitmap as follows:

- Access the LifePlanningSheet.cpp file and change it as follows:
CLifePlanningSheet::CLifePlanningSheet()
{
// 1. Classic property sheet
/* AddPage(&pgeComputerScience);
AddPage(&pgeInformationTechnology);
SetLook(CMFCPropertySheet::PropSheetLook_Tabs); */
// 2. Microsoft OneNote tabs
/* AddPage(&pgeComputerScience);
AddPage(&pgeInformationTechnology);
AddPage(&pgeHistory);
AddPage(&pgeCulinaryServices);
SetLook(CMFCPropertySheet::PropSheetLook_OneNoteTabs); */
// 3. Outlook Bar
/* AddPage(&pgeComputerScience);
AddPage(&pgeHistory);
AddPage(&pgeCulinaryServices);
m_OutlookImages.Create(IDB_OUTLOOK, 32, 1, RGB(128, 128, 128));
SetIconsList(m_OutlookImages);
SetLook(CMFCPropertySheet::PropSheetLook_OutlookBar); */
// 4. List
/* AddPage(&pgeComputerScience);
AddPage(&pgeInformationTechnology);
AddPage(&pgeCulinaryServices);
SetLook(CMFCPropertySheet::PropSheetLook_List, 150); */
// 5. Tree List
// Add the icons to the image list
m_TreeListImages.Create(IDB_TREELIST, 16, 1, RGB(255, 255, 255));
// Indicate that the tree list will use icons
SetIconsList(m_TreeListImages);
// Indicate that the property sheet will use a tree list
SetLook(CMFCPropertySheet::PropSheetLook_Tree, 185);
// Create the top node and name it College Majors
CMFCPropertySheetCategoryInfo * pscCollgeMajors =
AddTreeCategory(_T("College Majors"), 0, 1);
// Under the College Majors node, create a category named Computers Related
CMFCPropertySheetCategoryInfo * pscComputers =
AddTreeCategory(_T("Computers Related"), 0, 1, pscCollgeMajors);
// Add the pages to the category
AddPageToTree(pscComputers, &pgeComputerScience);
AddPageToTree(pscComputers, &pgeInformationTechnology);
// Add two categories to the College Majors node
AddPageToTree(pscCollgeMajors, &pgeHistory);
AddPageToTree(pscCollgeMajors, &pgeCulinaryServices);
// Add a node that doesn't have a parent
AddPage(&pgeCareers);
}
- Press F5 to execute
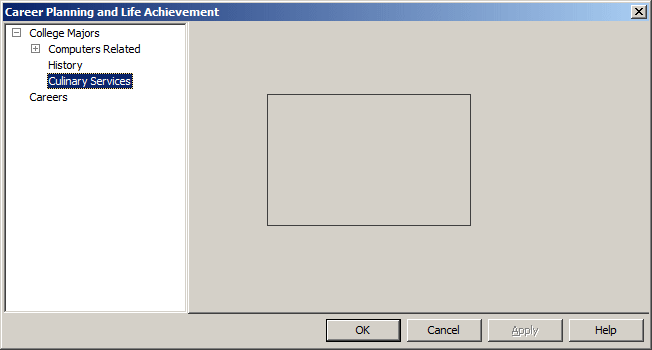
- Close the property sheet
- Close the dialog box and return to your programming environment
|
|