One of the most regular ways you will use the
CArchive class consists of storing or retrieving values through their
Serialize() member function. To do this directly and easily in the
class, the CArchive class overloads two operators: <<
and >>.
To store one or more values, the CArchive class
provides the << operator. The values you can store are of MFC, BYTE,
short, WORD, int, unsigned int, long,
LONG, DWORD, float, or double types. The class also
provides an exception handler for each kind. Therefore, the syntaxes for
this operator for the different data types are:
friend CArchive& operator <<( CArchive& ar, const CObject* pOb );
throw( CArchiveException, CFileException );
|
CArchive& operator <<( BYTE by );
throw( CArchiveException, CFileException );
|
CArchive& operator <<( WORD w );
throw( CArchiveException, CFileException );
|
CArchive& operator <<( int i );
throw( CArchiveException, CFileException );
|
CArchive& operator <<( LONG l );
throw( CArchiveException, CFileException );
|
CArchive& operator <<( DWORD dw );
throw( CArchiveException, CFileException );
|
CArchive& operator <<( float f );
throw( CArchiveException, CFileException );
|
CArchive& operator <<( double d );
throw( CArchiveException, CFileException );
|
As you may have realized, there is only one version of
the Serialize() member function. That is, this member
function is called once for both storing and retrieving. Therefore, when
using it, you must specify what operation you want to perform. This checking
process can be performed by calling the CArchive::IsStoring() Boolean
member function. Its syntax is:
BOOL IsStoring() const;
This member function returns TRUE if you are storing
data. If the answer is then TRUE, you can use the << operator to store each
necessary value.
Practical
Learning: Archiving Some Values
|
|
- On the dialog box, double-click the Save button
- Implement its event as follows:
void CCPAR1Dlg::OnBnClickedSaveBtn()
{
UpdateData(TRUE);
if( m_CustomerName.GetLength() == 0 )
{
AfxMessageBox(L"You must enter the name of the customer whose car was repaired.");
return;
}
if( (m_Make.GetLength() == 0) || (m_Model.GetLength() == 0) )
{
AfxMessageBox(L"You must type the make and model of the car that was repaired.");
return;
}
if( m_FileSave.GetLength() == 0 )
{
AfxMessageBox(L"You must enter a name for the file to save.");
return;
}
CFile fleRepairOrder;
fleRepairOrder.Open(m_FileSave + L".rpr", CFile::modeCreate | CFile::modeWrite);
CArchive ar(&fleRepairOrder, CArchive::store);
ar << m_CustomerName << m_PhoneNumber << m_Make << m_Model << m_Year
<< m_ProblemDescription << m_PartsCost << m_LaborCost << m_Total;
ar.Close();
fleRepairOrder.Close();
OnBnClickedResetBtn();
UpdateData(FALSE);
}
- Execute the application to test
- Enter the Customer Name as Edward Rearson
- Enter the Phone # as (301) 105-0448
- Enter a Make as Ford
- Enter the Model as Taurus
- Enter the Year as 2005
- Enter the Problem Description as The customer wanted all 4
tires replaced
- Enter the Parts Cost as 341.80
- Enter the Labor Cost as 95.50
- In the Save Repair Order As, type EdwardRearson
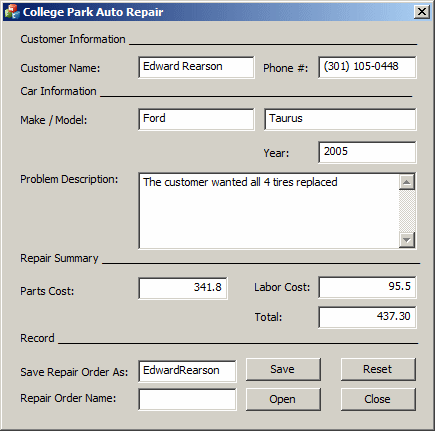
- Click Save
- Create another repair order before clicking Save
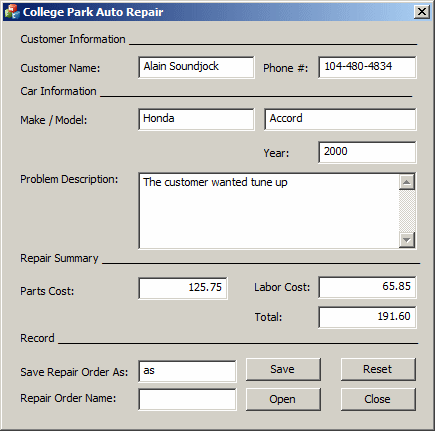
- Close the dialog box and return to your programming environment
As opposed to storing value(s), to retrieve (a)
value(s), the CArchive class provides the >> operator. It
comes in the following syntaxes:
friend CArchive& operator >>( CArchive& ar, CObject *& pOb );
throw( CArchiveException, CFileException, CMemoryException );
|
friend CArchive& operator >>( CArchive& ar, const CObject *& pOb );
throw( CArchiveException, CFileException, CMemoryException );
|
CArchive& operator >>( BYTE& by );
throw( CArchiveException, CFileException );
|
CArchive& operator >>( WORD& w );
throw( CArchiveException, CFileException );
|
CArchive& operator >>( int& i );
throw( CArchiveException, CFileException );
|
CArchive& operator >>( LONG& l );
throw( CArchiveException, CFileException );
|
CArchive& operator >>( DWORD& dw );
throw( CArchiveException, CFileException );
|
CArchive& operator >>( float& f );
throw( CArchiveException, CFileException );
|
CArchive& operator >>( double& d );
throw( CArchiveException, CFileException );
|
This indicates that you can retrieve an MFC object, a
BYTE, a short, a WORD, an int, an unsigned int,
a long, a LONG, a DWORD, a float, or a double
value.
As mentioned above, the Serialize()
member function is used for both operations. Therefore, when using this
member function, you should first check what operation is being performed.
This can be done by calling the IsLoading() member
function. Its syntax is:
BOOL IsLoading() const;
This member function returns TRUE if you are retrieving
a value or some values. If it is true, you can then use the >> operator to
retrieve the value(s).
Practical
Learning: Reading Values From an Archive
|
|
- On the dialog box, double-click the Open button
- Implement its event as follows:
void CCPAR1Dlg::OnBnClickedOpenBtn()
{
UpdateData(TRUE);
if( m_FileOpen.GetLength() == 0 )
{
AfxMessageBox(L"You must enter a name for the file to open.");
return;
}
CFile fleRepairOrder;
fleRepairOrder.Open(m_FileOpen + L".rpr", CFile::modeRead);
CArchive ar(&fleRepairOrder, CArchive::load);
ar >> m_CustomerName >> m_PhoneNumber >> m_Make >> m_Model >> m_Year
>> m_ProblemDescription >> m_PartsCost >> m_LaborCost >> m_Total;
ar.Close();
fleRepairOrder.Close();
UpdateData(FALSE);
}
- Execute the application to test the dialog box
- In the File to Open edit control, enter the name of the file you
previously created (BG) and click Open
- Close the dialog box and return to your programming environment
Automatic File Processing
|
|
Automatic File Processing consists of saving or opening
a file with little to no intervention from the user. This technique is used
to provide values that should be automatically provided to the user. This
technique also allows the user to create or add a new value to a list that
appears to be incomplete.
|
|