Launch Applications
|
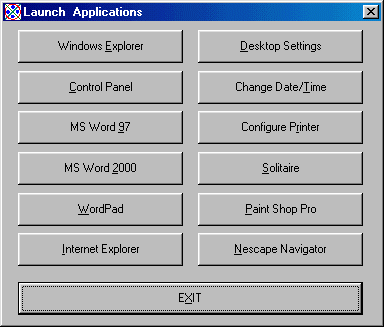 |
|
1 - |
|
Start Microsoft Visual C++. |
2 - |
a/ |
Create a Dialog-based application
called Applications. |
|
b/ |
Change the title of the dialog to Launch
Applications.
|
|
c/ |
Create the necessary buttons
corresponding to the applications you want to launch.
Here is an example that I use to make my point. The hard drive I am
working on is made of different partitions and different applications are
installed all over the place, for example MS Office 97 is installed in E:\Program
Files, MS Office 2000 is installed in D:\Program Files, Microsoft Internet Explorer is on C:\Program Files,
Netscape Navigator is installed in D:\Program Files, and Jasc Paint Shop Pro
6 is installed in D:\Program Files. There are other stuffs on F:\. To
launch an application, you should know where it is located.
Since I don't know your computer, I can't figure which applications you
have or want to use. The applications located in C:\Windows
are easy to launch. To show you how you can launch other applications, I
will assume that you have Microsoft Internet Explorer; if you are using
Netscape, the process is the same. Create the following buttons:
Button ID: |
Button
Caption |
IDC_EXPLORER_BTN |
Windows &Explorer |
IDC_CTLPANEL_BTN |
&Control Panel |
IDC_DESKTOP_BTN |
&Desktop Settings |
IDC_DATETIME_BTN |
Change Date/&Time |
IDC_PRINTERS_BTN |
Configure P&rinter |
IDC_WORDPAD_BTN |
&WordPad |
IDC_SOLITAIRE_BTN |
&Solitaire |
IDC_IE_BTN |
&Internet Explorer |
IDOK |
E&XIT |
We will first implement the launching of the applications that are easy
to locate.
Many applications that are installed with your computer are located in C:\Windows.
You can open any of them by simply calling it from the WinExec()
function.
The WinExec() function takes two arguments, the first one specifies the location
or Command Line of the executable file or application, the second one
specifies how the window will be displayed when the application
starts.
|
|
d/ |
Right-click on the Windows
Explorer button and choose Class Wizard. |
|
e/ |
In the MFC ClassWizard dialog, make sure that the Message Maps property
sheet is selected, the Class Name is CApplicationsDlg, and
IDC_EXPLORER_BTN
is selected in the Object IDs list box. In the Messages list box, click BN_CLICKED, click the
Add Function... button, accept the suggested name of
the function by clicking OK, and click the Edit Code button.
|
f/ |
Implement the new function as follows:
void CApplicationsDlg::OnExplorerBtn()
{
WinExec("Explorer.exe", SW_MAXIMIZE);
}
|
g/ |
Press Ctrl + W to access the ClassWizard.
|
h/ |
In the Message Maps property sheet, in the Object IDs list box, click
IDC_CTLPANEL_BTN. In the Messages list box, double-click BN_CLICKED. Change
the name of the function to OnControlPanelBtn and click OK. Click Edit Code.
|
i/ |
Implement the function as follows:
void CApplicationsDlg::OnControlPanelBtn()
{
WinExec("Control.exe", SW_NORMAL);
}
|
j/ |
Right-click anywhere on the function and choose ClassWizard. Add an
OnDesktopBtn() function based on IDC_DESKTOP_BTN.
|
|
k/ |
Implement the function as follows:
void CApplicationsDlg::OnDesktopBtn()
{
WinExec("Control.exe Desktop", SW_NORMAL);
}
|
|
l/ |
On the main menu, click View ->
ClassWizard... |
|
m/ |
Create an OnDateTimeBtn() function
based on IDC_DATETIME_BTN. |
|
n/ |
Implement the function as follows:
void CApplicationsDlg::OnDateTimeBtn()
{
WinExec("Control.exe Date/Time", SW_NORMAL);
}
|
|
o/ |
Implement the OnPrintersBtn() (based on the IDC_PRINTERS_BTN) function as
follows:
void CApplicationsDlg::OnPrintersBtn()
{
WinExec("Control.exe Printers", SW_NORMAL);
}
|
|
p/ |
Implement the OnWordPadBtn() and the OnSolitaireBtn() functions as follows:
|
|
|
void CApplicationsDlg::OnWordPadBtn()
{
WinExec("Write.exe", SW_MAXIMIZE);
}
void CApplicationsDlg::OnSolitaireBtn()
{
WinExec("Sol.exe", SW_NORMAL);
}
|
|
f/ |
The only problem with the other applications is to get their command line or
location.
Invoke the ClassWizard dialog. Create a function based on IDC_IE_BTN,
call the function OnIntExplorerBtn.
Edit its code as follows:
|
|
|
void CApplicationsDlg::OnIntExporerBtn()
{
WinExec(, SW_MAXIMIZE);
}
|
|
g/ |
Here is one step you can follow to locate Internet Explorer and implement
its function.
On the Taskbar, click Start -> Programs, there are
probably two Internet Explorer(s) in the Programs group, one would be a program folder (its icon looks exactly like that of Accessories), the
other would an application (its icon would be a blue e); right-click the
Internet
Explorer application and choose Properties.
|
|
h/ |
In the Internet Explorer
Properties dialog, click the Shortcut property sheet (that property sheet
should already be selected by default). The target edit box should be
highlighted, otherwise, highlight it. Right-click in the selected target
box and choose Copy.
Here is another way
you can copy an application's command line.
First, you need to locate the application's executable file: you could
do a search on the name of the application (for example, click Start ->
Search -> Files or Folders... In the Find What, type notepad and press
Enter) or you could use Windows Explorer. Most (commercial) applications
are installed in C:\Program Files
Here is an example:
a) Open Windows Explorer.
b) Let's say you want to launch WordPad. By experience, we know that
WordPad is located in C:\Program Files\Accessories. Therefore, expand the
C: drive, expand the Program Files folder, and click Accessories.
c) The whole path should be displaying in the Address combo box. If it
displays like this you have
to ask the computer to display the whole path. To do that, on the main
menu of Windows Explorer, click Tools -> Folder Options... In the
Folder Options dialog, click the View property sheet. Click the Display
The Full Path In the Address Bar check box:
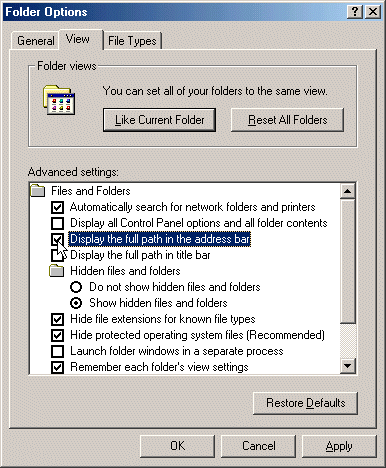
Now you should have the whole path.
Right-click in the Address combo box where the path is displaying. And
click Copy.

d) All you have to do now is to paste the path to your code.
|
|
i/ |
In Visual C++, click between the opening parenthesis and the comma inside
the WinExec() function. On the main menu, click Edit ->
Paste.
Double each one of the backslashes in the function so that when you
finish, the function should look as follows:
|
|
|
void CApplicationsDlg::OnIntExporerBtn()
{
WinExec("C:\\Program Files\\Internet Explorer\\IEXPLORE.EXE",
SW_MAXIMIZE);
}
|
|
|
|
j/ |
To execute this
application, on the main menu, click Build -> Set Active
Configuration... Click Applications - Win32 Release and click
OK. |
|
k/ |
Build and execute the
exercise. |
|
|
Source Code |
|