 |
Introduction to Conditional Statements |
|
If a Condition is True
Introduction
A conditional statement is an expression that produces a True or False result. To create the expression, you use the Boolean operators we studied in the previous lesson.
Creating a Boolean Condition
To check whether an expression is true or not in order to use its Boolean result, you can create an If...Then statement. The formula to follow is:
If condition Then statement
The condition can be the type of Boolean operation we studied in the previous lesson. That is, it can have the following formula:
operand1 Boolean-operator operand2
If the condition produces a True result, then the statement executes. This can be illustrated as follows:
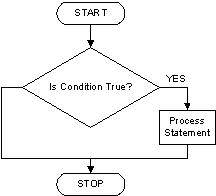
If the conditional statement is short, you can write the condition and the statement on the same line. Here is an example:
<%@ Page Language="VB" %>
<!DOCTYPE html>
<html>
<head>
<script runat="server">
Sub btnCalculateClick(ByVal sender As Object, ByVal e As EventArgs)
Dim pricePerCCF As Double
Dim monthlyCharges As Double
Dim consumption As Double
pricePerCCF = 50.0
monthlyCharges = 0.0
consumption = CDbl(txtConsumption.Text)
If consumption >= 0.50 Then pricePerCCF = 35.00
txtPricePerCCF.Text = pricePerCCF
pricePerCCF = CDbl(txtPricePerCCF.Text)
monthlyCharges = consumption * pricePerCCF
txtMonthlyCharges.Text = monthlyCharges
End Sub
</script>
<title>Gas Utility Company</title>
</head>
<body>
<div align="center">
<form id="frmBillPreparation" runat="server">
<h3>Gas Utility Company</h3>
<table border=0>
<tr>
<td>Consumption:</td>
<td><asp:TextBox id="txtConsumption"
runat="server" /></td>
</tr>
<tr>
<td> </td>
<td style="text-align: center">
<asp:Button id="btnCalculate"
text="Calculate"
OnClick="btnCalculateClick"
runat="server"></asp:Button></td>
</tr>
<tr>
<td>Price Per CCF:</td>
<td><asp:TextBox id="txtPricePerCCF" runat="server" /></td>
</tr>
<tr>
<td>Monthly Charges:</td>
<td><asp:TextBox id="txtMonthlyCharges" runat="server" /></td>
</tr>
</table>
</form>
</div>
</body>
</html>
Here is an example of using the webpage:
If the conditional statement is long, you can write the If...Then expression one line and the statement on the next line but then you must indicate the end of the conditional statement with End If. It is also a good idea (to make your code easy to read) to indent the statement. The formula to follow is:
If condition Then
statement
End If
Here is an example:
<script runat="server">
Sub btnCalculateClick(ByVal sender As Object, ByVal e As EventArgs)
Dim pricePerCCF As Double
Dim monthlyCharges As Double
Dim consumption As Double
pricePerCCF = 50.0
monthlyCharges = 0.0
consumption = CDbl(txtConsumption.Text)
If consumption >= 0.50 Then
pricePerCCF = 35.00
End If
txtPricePerCCF.Text = pricePerCCF
pricePerCCF = CDbl(txtPricePerCCF.Text)
monthlyCharges = consumption * pricePerCCF
txtMonthlyCharges.Text = monthlyCharges
End Sub
</script>
You can also write the statement on its own line even
if the statement is short enough to fit on the same line with the If...Then expression. If the statement contains, or must use, more that one line of code, you must create the statements in the line next to the If...Then expression. Here is an example:
<%@ Page Language="VB" %>
<!DOCTYPE html>
<html>
<head>
<script language="VB" runat="server">
Sub btnCalculateClick(ByVal sender As Object, ByVal e As EventArgs)
Dim weeklySalary As Double
Dim hourlySalary As Double
Dim timeWorked As Double
Dim overtime As Double
Dim overtimePay As Double
weeklySalary = 0.0
hourlySalary = CDbl(txtHourlySalary.Text)
timeWorked = CDbl(txtTimeWorked.Text)
weeklySalary = hourlySalary * timeWorked
If timeWorked > 40.0 Then
overtime = timeWorked - 40.0
overtimePay = hourlySalary * 1.5 * overtime
weeklySalary = (hourlySalary * 40.0) + overtimePay
End If
txtWeeklySalary.Text = weeklySalary
End Sub
</script>
<title>Payroll Preparation</title>
</head>
<body>
<div align="center">
<form id="frmPayroll" runat="server">
<h3>Payroll Preparation</h3>
<table border=0>
<tr>
<td style="text-align: left">
<asp:Label ID="lblHourlySalary" runat="server" Text="Hourly Salary:"></asp:Label>
</td>
<td style="text-align: left">
<asp:TextBox id="txtHourlySalary" style="Width:65px"
text="0.00" runat="server" /></td>
</tr>
<tr>
<td style="text-align: left">
<asp:Label ID="lblTimeWorked" runat="server" Text="Time Worked:"></asp:Label></td>
<td style="text-align: left">
<asp:TextBox id="txtTimeWorked" text="0.00" runat="server" style="Width:65px" />
<asp:Button id="btnCalculate"
text = "Calculate"
OnClick = "btnCalculateClick"
runat="server"></asp:Button></td>
</tr>
<tr>
<td style="text-align: left">
<asp:Label ID="lblWeeklySalary" runat="server" Text="Weekly Salary:"></asp:Label></td>
<td style="text-align: left">
<asp:TextBox id="txtWeeklySalary" text="0.00" style="Width:65px" runat="server" /></td>
</tr>
</table>
</form>
</div>
</body>
</html>
If a Condition is False
Something Else
To address an alternative to an If...Then expression, you can use the Else condition. The formula to follow is:
If condition Then)
statement1
Else
statement2
End If
If the condition produces a True result, then statement1 would execute. If the condition is
false, statement2 would execute. Here is an example:
<%@ Page Language="VB" %>
<!DOCTYPE html>
<html>
<head runat="server">
<script runat="server">
Sub btnCalculateClick(ByVal sender As Object, ByVal e As EventArgs)
Dim side As Double
Dim perimeter As Double
Dim area As Double
If txtSide.Text = "" Then
side = 0.00
Else
side = txtSide.Text
End If
perimeter = side * 4
area = side * side
txtPerimeter.Text = perimeter
txtArea.Text = area
End Sub
</script>
<title>Geometry: Square</title>
</head>
<body>
<div align="center">
<h2>Geometry: Square</h2>
<form id="frmSquare" method="get" runat="server">
<table>
<tr>
<td><asp:Label id="lblSide" Text="Side:" runat="server"></asp:Label></td>
<td>
<asp:TextBox Runat="server" id="txtSide"></asp:TextBox>
<asp:Button Runat="server" id="btnCalculate"
Text="Calculate"
OnClick="btnCalculateClick"></asp:Button></td>
</tr>
<tr>
<td><asp:Label id="lblPerimeter" Text="Perimeter:"
runat="server"></asp:Label></td>
<td><asp:TextBox Runat="server"
id="txtPerimeter"></asp:TextBox></td>
</tr>
<tr>
<td><asp:Label id="lblArea" Text="Area:" runat="server"></asp:Label></td>
<td><asp:TextBox Runat="server"
id="txtArea"></asp:TextBox></td>
</tr>
</table>
</form>
</div>
</body>
</html>
Here is an example of running the program:
Like the If statement, the Else statement
can cover more than one line of code, in which case the lines should be
indented. Here is an example:
<%@ Page Language="VB" %>
<!DOCTYPE html>
<html>
<head>
<script language="VB" runat="server">
Sub btnCalculateClick(ByVal sender As Object, ByVal e As EventArgs)
Dim weeklySalary As Double
Dim hourlySalary As Double
Dim timeWorked As Double
Dim overtime As Double
Dim overtimePay As Double
weeklySalary = 0.0
hourlySalary = CDbl(txtHourlySalary.Text)
timeWorked = CDbl(txtTimeWorked.Text)
If timeWorked <= 40.0 Then
weeklySalary = hourlySalary * timeWorked
Else
overtime = timeWorked - 40.0
overtimePay = hourlySalary * 1.50 * overtime
weeklySalary = (hourlySalary * 40.0) + overtimePay
End If
txtWeeklySalary.Text = FormatNumber(weeklySalary)
End Sub
</script>
<title>Payroll Preparation</title>
</head>
<body>
<div align="center">
<form id="frmPayroll" runat="server">
<h3>Payroll Preparation</h3>
<table border=0>
<tr>
<td style="text-align: left">
<asp:Label ID="lblHourlySalary" runat="server" Text="Hourly Salary:"></asp:Label>
</td>
<td style="text-align: left">
<asp:TextBox id="txtHourlySalary" style="Width:65px"
text="0.00" runat="server" /></td>
</tr>
<tr>
<td style="text-align: left">
<asp:Label ID="lblTimeWorked" runat="server" Text="Time Worked:"></asp:Label></td>
<td style="text-align: left">
<asp:TextBox id="txtTimeWorked" text="0.00" runat="server" style="Width:65px" />
<asp:Button id="btnCalculate"
text = "Calculate"
OnClick = "btnCalculateClick"
runat="server"></asp:Button></td>
</tr>
<tr>
<td style="text-align: left">
<asp:Label ID="lblWeeklySalary" runat="server" Text="Weekly Salary:"></asp:Label></td>
<td style="text-align: left">
<asp:TextBox id="txtWeeklySalary" text="0.00" style="Width:65px" runat="server" /></td>
</tr>
</table>
</form>
</div>
</body>
</html>
Here is an example of using the webpage:
Here is another example of using the webpage:
The If Operator
To provide a simple way to create a conditional statement,
the Visual Basic language provides the If operator. The formula to use it
is:
If(boolean-condition, true-result, false-result)
The.boolean-condition is first evaluated. If it
produces a true result, the second argument is produced. If not, the third
argument is produced. Here is an example:
<script runat="server">
Sub btnCalculateClick(ByVal sender As Object, ByVal e As EventArgs)
Dim side As Double
Dim perimeter As Double
Dim area As Double
side = If(txtSide.Text = "", 0.00, txtSide.Text)
perimeter = side * 4
area = side * side
txtPerimeter.Text = perimeter
txtArea.Text = area
End Sub
</script>
What Else If a Condition is True?
An If...Then...Else conditional statement can process only two statements. If you need to process more
conditions, you can add an ElseIf condition. The formula to follow is:
If Condition1 Then
statement1
ElseIf condition2 Then
statement2
End If
The first condition, condition1, would be checked. If
condition1 is true, then statement1 would execute. If condition1
is false, then condition2 would be checked. If condition2
is true, then statement2 would execute. Any other result would be
ignored.
If you need to process more than two conditions, you can
add more ElseIf sections. The formula to follow would be:
If condition1 Then
statement1
ElseIf condition2 Then
statement2
ElseIf condition3 Then
Statement3
. . .
ElseIf condition-n Then
Statement-n
End If
Here is an example:
<%@ Page Language="VB" %>
<!DOCTYPE html>
<html>
<head>
<script runat="server">
Sub btnFindClick(ByVal sender As Object, ByVal e As EventArgs)
Dim chemicalSymbol As String
Dim atomicNumber As String
Dim elementName As String
Dim atomicMass As Single
If txtChemicalSymbol.Text = "" Then
chemicalSymbol = "H"
Else
chemicalSymbol = txtChemicalSymbol.Text
End If
If chemicalSymbol = "H" Then
atomicNumber = 1
elementName = "Hydrogen"
atomicMass = 1.0079
ElseIf chemicalSymbol = "He" Then
atomicNumber = 2
elementName = "Helium"
atomicMass = 4.002682
ElseIf chemicalSymbol = "Li" Then
atomicNumber = 3
elementName = "Lithium"
atomicMass = 6.941
ElseIf chemicalSymbol = "Be" Then
atomicNumber = 4
elementName = "Berylium"
atomicMass = 9.0122
ElseIf chemicalSymbol = "B" Then
atomicNumber = 5
elementName = "Boron"
atomicMass = 10.811
End If
lblAtomicNumber.Text = atomicNumber
lblElementName.Text = elementName
lblAtomicMass.Text = CStr(atomicMass)
End Sub
</script>
<title>Chemistry: Compound Properties</title>
</head>
<body>
<div align="center">
<form id="frmChemistry" runat="server">
<h3>Chemistry: Compound Properties</h3>
<table border=0>
<tr>
<td>Chemical Symbol:</td>
<td><asp:TextBox id="txtChemicalSymbol" style="width: 40px" runat="server" />
<asp:Button id="btnFind" text="Find"
OnClick="btnFindClick"
runat="server"></asp:Button></td>
</tr>
<tr>
<td>Atomic Number</td>
<td><asp:Label id="lblAtomicNumber" runat="server"></asp:Label></td>
</tr>
<tr>
<td>Element Name:</td>
<td><asp:Label id="lblElementName" runat="server" /></td>
</tr>
<tr>
<td>Atomic Mass:</td>
<td><asp:Label id="lblAtomicMass" runat="server" /></td>
</tr>
</table>
</form>
</div>
</body>
</html>
Here is an example of using the webpage:
Whatever Else?
If none of the conditions matches, you can add a last Else condition. The formulas
to follow are:
If condition1 Then
statement1
ElseIf condition2 Then
statement2
Else
else-statement
End If
or
If condition1 Then
statement1
ElseIf condition2 Then
statement2
ElseIf condition3 Then
Statement3
. . .
ElseIf condition-n Then
Statement-n
Else
else-statement
End If
Here is an example:
<%@ Page Language="VB" %>
<!DOCTYPE html>
<html>
<head>
<script runat="server">
Sub btnFindClick(ByVal sender As Object, ByVal e As EventArgs)
Dim chemicalSymbol As String
Dim atomicNumber As String
Dim elementName As String
Dim atomicMass As Single
If txtChemicalSymbol.Text <> "" Then
chemicalSymbol = txtChemicalSymbol.Text
End If
If chemicalSymbol = "H" Then
atomicNumber = 1
elementName = "Hydrogen"
atomicMass = 1.0079
ElseIf chemicalSymbol = "He" Then
atomicNumber = 2
elementName = "Helium"
atomicMass = 4.002682
ElseIf chemicalSymbol = "Li" Then
atomicNumber = 3
elementName = "Lithium"
atomicMass = 6.941
ElseIf chemicalSymbol = "Be" Then
atomicNumber = 4
elementName = "Berylium"
atomicMass = 9.0122
ElseIf chemicalSymbol = "B" Then
atomicNumber = 5
elementName = "Boron"
atomicMass = 10.811
Else
atomicNumber = 0
elementName = "Unknown"
atomicMass = 0.0000
End If
lblAtomicNumber.Text = atomicNumber
lblElementName.Text = elementName
lblAtomicMass.Text = CStr(atomicMass)
End Sub
</script>
<title>Chemistry: Compound Properties</title>
</head>
<body>
<div align="center">
<form id="frmChemistry" runat="server">
<h3>Chemistry: Compound Properties</h3>
<table border=0>
<tr>
<td>Chemical Symbol:</td>
<td><asp:TextBox id="txtChemicalSymbol" style="width: 40px" runat="server" />
<asp:Button id="btnFind" text="Find"
OnClick="btnFindClick"
runat="server"></asp:Button></td>
</tr>
<tr>
<td>Atomic Number</td>
<td><asp:Label id="lblAtomicNumber" runat="server"></asp:Label></td>
</tr>
<tr>
<td>Element Name:</td>
<td><asp:Label id="lblElementName" runat="server" /></td>
</tr>
<tr>
<td>Atomic Mass:</td>
<td><asp:Label id="lblAtomicMass" runat="server" /></td>
</tr>
</table>
</form>
</div>
</body>
</html>
Here is an example of using the webpage:
Accessories for Conditional Statements
Something Is
The Visual Basic language provides a comparison operator
used to compared two objects of classes. The operator is named Is. The formula to use it is:
comparison-result = object1 Is object2
The Is operator compares the references of two
objects, not the values of the objects. If the references point to the same
object, the comparison produces a True result. Otherwise, the comparison is
False.
Something Is Not
To let you find out if two objects are not the same, the Visual Basic language provides
the IsNot comparison operator. The formula to use it is:
comparison-result = object1 IsNot object2
The IsNot operator compares the references of two
objects. If the objects are the same, the comparison produces True result; otherwise,
it produces
False.
If Something Is Nothing
Remember that when an object is created, if the New keyword
is not used to allocate memory for it, the object is said to be Nothing.
Also remember that, if an object was already difined, if for some or any reason you
want to undefine it, you can assign Nothing to it. Here is an
example we saw in Lesson 07:
<%@ Page Language="VB" %>
<!DOCTYPE html>
<html>
<head>
<script Language="VB" runat="server">
Class Employee
End Class
</script>
<title>Department Store - Employee Record</title>
</head>
<body>
<h4>Department Store - Employee Record</h4>
<%
Dim staff As New Employee
staff = Nothing
%>
</body>
</html>
At any time, to find out whether an object is
currently defined or it is not holding a clear value, use the Is operator to compare it to the Nothing object.
Here are examples:
<%@ Page Language="VB" %>
<!DOCTYPE html>
<html>
<head>
<script runat="server">
Class Employee
End Class
</script>
<title>Department Store - Employee Record</title>
</head>
<body>
<h4>Department Store - Employee Record</h4>
<%
Dim staff As New Employee
Response.Write("Dim staff As New Employee")
If staff Is Nothing Then
Response.Write("<br>The staff member is currently not defined.")
Else
Response.Write("<br>The staff member has a defined object.")
End If
staff = Nothing
Response.Write("<br><br>staff = Nothing")
If staff Is Nothing Then
Response.Write("<br>The staff member is currently not defined.")
Else
Response.Write("<br>The staff member has a defined object.")
End If
%>
</body>
</html>
This would produce:

The If Operator for Nothing
To provide a simple way to find out if a value or an object
is Nothing, the If operator provides the following formula:
If(object/value is nothing, object/value-result)
If the first argument is nothing, the second argument is
produced.
Going To a Statement
In the flow of your code, you can jump from one statement or line to another. This is made possible with the GoTo keyword. Before using it, first create or insert a name on a particular section of your procedure or function. The name, also called a label, is made of one word and it can be anything. That name is followed by a colon ":". Here
is an example:
<script language="VB" runat="server">
Sub btnCalculateClick(ByVal sender As Object, ByVal e As EventArgs)
' Do some thing(s) here
RegularTime:
' Do some other thing(s) here
End Sub
After creating the label, if a condition calls to jump to the label, type GoTo followed by the label. In the same way, you can create as many labels as you
want. The name of each label must be unique in the same procedure. Here is an example:
<%@ Page Language="VB" %>
<!DOCTYPE html>
<html>
<head>
<script language="VB" runat="server">
Sub btnCalculateClick(ByVal sender As Object, ByVal e As EventArgs)
Dim weeklySalary As Double
Dim hourlySalary As Double
Dim timeWorked As Double
Dim overtime As Double
Dim overtimePay As Double
weeklySalary = 0.0
hourlySalary = CDbl(txtHourlySalary.Text)
timeWorked = CDbl(txtTimeWorked.Text)
If timeWorked > 40.0 Then
GoTo GetOvertimeApproval
Else
GoTo RegularTime
End If
RegularTime:
weeklySalary = hourlySalary * timeWorked
GoTo DisplayWeeklySalary
GetOvertimeApproval:
overtime = timeWorked - 40.0
overtimePay = hourlySalary * 1.50 * overtime
weeklySalary = (hourlySalary * 40.0) + overtimePay
lblApproval.Text = "Make sure you have received approval for paid overtime."
GoTo DisplayWeeklySalary
DisplayWeeklySalary:
txtWeeklySalary.Text = FormatNumber(weeklySalary)
End Sub
</script>
<title>Payroll Preparation</title>
</head>
<body>
<div align="center">
<form id="frmPayroll" runat="server">
<h3>Payroll Preparation</h3>
<table border=0>
<tr>
<td style="text-align: left">
<asp:Label id="lblHourlySalary" runat="server" Text="Hourly Salary:"></asp:Label>
</td>
<td style="text-align: left">
<asp:TextBox id="txtHourlySalary" style="Width:65px"
text="0.00" runat="server" /></td>
</tr>
<tr>
<td style="text-align: left">
<asp:Label id="lblTimeWorked" runat="server" Text="Time Worked:"></asp:Label></td>
<td style="text-align: left">
<asp:TextBox id="txtTimeWorked" text="0.00" runat="server" style="Width:65px" />
<asp:Button id="btnCalculate"
text = "Calculate"
OnClick = "btnCalculateClick"
runat="server"></asp:Button></td>
</tr>
<tr>
<td style="text-align: left">
<asp:Label id="lblWeeklySalary" runat="server" Text="Weekly Salary:"></asp:Label></td>
<td style="text-align: left">
<asp:TextBox id="txtWeeklySalary" text="0.00" style="Width:65px" runat="server" /></td>
</tr>
</table>
<asp:Label ID="lblApproval" runat="server" Text=""></asp:Label>
</form>
</div>
</body>
</html>
Here is an example of using the webpage:

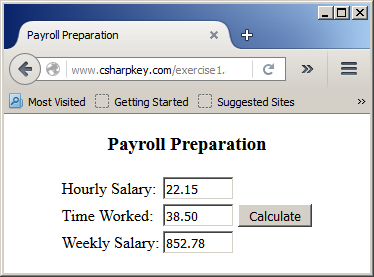

Negating a Conditional Statement
As you should be aware by now, Boolean algebra stands by two
values, True and False, that are opposite each other. In fact, Visual Basic provides an
operator that can be used to reverse a Boolean value or to negate a Boolean
expression. This is the role of the Not operator. The formula to use it is:
Not Expression
To use the operator, type Not followed by a
logical expression. The expression can be a simple Boolean value. Here is
an example:
<%@ Page Language="VB" %>
<!DOCTYPE html>
<html>
<head>
<script language="VB" runat="server">
</script>
<title>Payroll Preparation</title>
</head>
<body>
<%
Dim IsMarried As Boolean
Response.Write("Employee is married: " & IsMarried)
Response.Write("<br>Employee is married: " & Not IsMarried)
%>
</body>
</html>
This would produce:

In this case, the Not operator is used to change the
logical value of the variable. When a Boolean variable has been "notted", its logical value has
changed. If the logical value was True, it would be changed to False
and vice versa. Therefore, you can inverse the logical value of a Boolean variable
by "notting" or not "notting" it. Here is an example:
<script runat="server">
Sub btnFindClick(ByVal sender As Object, ByVal e As EventArgs)
Dim chemicalSymbol As String
If Not txtChemicalSymbol.Text = "" Then
chemicalSymbol = txtChemicalSymbol.Text
End If
End Sub
</script>
To make the code easier to read, it is a good idea to pu the
negative expression in parentheses
<%@ Page Language="VB" %>
<!DOCTYPE html>
<html>
<head>
<script runat="server">
Sub btnFindClick(ByVal sender As Object, ByVal e As EventArgs)
Dim chemicalSymbol As String
Dim atomicNumber As String
Dim elementName As String
Dim atomicMass As Single
If Not (txtChemicalSymbol.Text = "") Then
chemicalSymbol = txtChemicalSymbol.Text
End If
If chemicalSymbol = "H" Then
atomicNumber = 1
elementName = "Hydrogen"
atomicMass = 1.0079
ElseIf chemicalSymbol = "He" Then
atomicNumber = 2
elementName = "Helium"
atomicMass = 4.002682
ElseIf chemicalSymbol = "Li" Then
atomicNumber = 3
elementName = "Lithium"
atomicMass = 6.941
ElseIf chemicalSymbol = "Be" Then
atomicNumber = 4
elementName = "Berylium"
atomicMass = 9.0122
ElseIf chemicalSymbol = "B" Then
atomicNumber = 5
elementName = "Boron"
atomicMass = 10.811
Else
atomicNumber = 0
elementName = "Unknown"
atomicMass = 0.0000
End If
lblAtomicNumber.Text = atomicNumber
lblElementName.Text = elementName
lblAtomicMass.Text = CStr(atomicMass)
End Sub
</script>
<title>Chemistry: Compound Properties</title>
</head>
<body>
<div align="center">
<form id="frmChemistry" runat="server">
<h3>Chemistry: Compound Properties</h3>
<table border=0>
<tr>
<td>Chemical Symbol:</td>
<td><asp:TextBox id="txtChemicalSymbol" style="width: 40px" runat="server" />
<asp:Button id="btnFind" text="Find"
OnClick="btnFindClick"
runat="server"></asp:Button></td>
</tr>
<tr>
<td>Atomic Number</td>
<td><asp:Label id="lblAtomicNumber" runat="server"></asp:Label></td>
</tr>
<tr>
<td>Element Name:</td>
<td><asp:Label id="lblElementName" runat="server" /></td>
</tr>
<tr>
<td>Atomic Mass:</td>
<td><asp:Label id="lblAtomicMass" runat="server" /></td>
</tr>
</table>
</form>
</div>
</body>
</html>
Here is an example of using the webpage:
Conditional Statements and Functions
Introduction
When performing its assignment, a function can encounter
different situations, some of which would need to be checked for
a true value, a false value or a negation. Therefore, a conditional statement can
assist a procedure or function to decide what action to take or what value to
produce.
Conditional Returns
A function can return only one value but you can make it
produce a result
that depends on some condition. Here are two examples:
<%@ Page Language="VB" %>
<!DOCTYPE html>
<html>
<head>
<script language="VB" runat="server">
Function GetDiscountRate(ByVal price As Double, ByVal days As Integer) As Integer
Dim discountRate As Double
If days > 70 Then
discountRate = 70
ElseIf days > 50 Then
discountRate = 50
ElseIf days > 30 Then
discountRate = 35
ElseIf days > 15 Then
discountRate = 15
End If
Return discountRate
End Function
Function CalculateMarketPrice(ByVal price As Double, ByVal Optional rate As Integer = 0.00) As Integer
Dim discountAmt As Double
Dim markedPrice As Double
If rate = 0.00 Then
markedPrice = 0.00
Else
markedPrice = price * rate / 100.0
End If
Return markedPrice
End Function
Sub btnCreateRecordClick(ByVal sender As Object, ByVal e As EventArgs)
Dim unitPrice As Double
Dim markedPrice As Double
Dim discountRate As Double
Dim daysInStore As Integer
Dim discountedAmount As Double
markedPrice = 0.00
daysInStore = CInt(txtDaysInStore.Text)
unitPrice = CDbl(txtUnitPrice.Text)
discountRate = GetDiscountRate(unitPrice, daysInStore)
If discountRate = 0.00 Then
discountedAmount = CalculateMarketPrice(unitPrice)
Else
discountedAmount = CalculateMarketPrice(unitPrice, discountRate)
End If
markedPrice = unitPrice - discountedAmount
lblItemNumber.Text = txtItemNumber.Text
lblItemName.Text = txtItemName.Text
LblDiscountAmount.Text = FormatCurrency(discountedAmount)
lblMarkedPrice.Text = FormatCurrency(markedPrice)
End Sub
</script>
<title>Department Store - Inventory Creation</title>
</head>
<body>
<div align="center">
<form id="frmStoreItem" runat="server">
<h3>Department Store - Inventory Creation</h3>
<table>
<tr>
<td>Item #:</td>
<td><asp:TextBox id="txtItemNumber" runat="server" /></td>
</tr>
<tr>
<td>Item Name:</td>
<td><asp:TextBox id="txtItemName" style="width: 250px;" runat="server" /></td>
</tr>
<tr>
<td>Unit Price:</td>
<td><asp:TextBox id="txtUnitPrice" runat="server" /></td>
</tr>
<tr>
<td>Days in Store:</td>
<td><asp:TextBox id="txtDaysInStore" runat="server" /></td>
</tr>
<tr>
<td> </td>
<td><asp:Button id="btnCreateSaleRecord"
text="Create Sale Record"
OnClick="btnCreateRecordClick"
runat="server"></asp:Button> </td>
</tr>
</table>
<h3>Department Store - Store Inventory</h3>
<table>
<tr>
<td>Item #:</td>
<td><asp:Label id="lblItemNumber" runat="server" /></td>
</tr>
<tr>
<td>Item Name:</td>
<td><asp:Label id="lblItemName" runat="server" /></td>
</tr>
<tr>
<td>Discount Amouont:</td>
<td><asp:Label id="LblDiscountAmount" runat="server" /></td>
</tr>
<tr>
<td>Marked Price:</td>
<td><asp:Label id="lblMarkedPrice" runat="server" /></td>
</tr>
</table>
</form>
</div>
</body>
</html>
Here is an example of using the webpage:
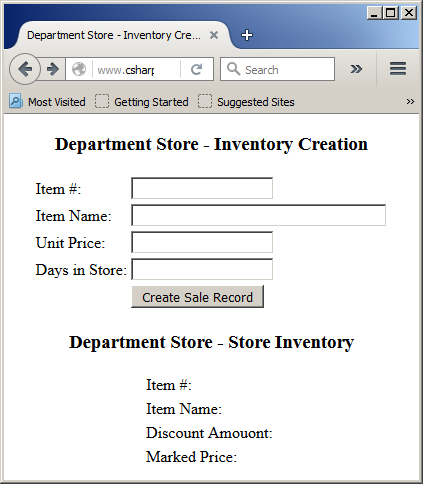


In our examples, we first declared a variable that was going
to hold the value to return. This is not always necessary. If you already have
the value or the expression to return, you can precede it with the Return. You
can repeat this for each section where the value or expression can/must be
returned. Here are examples:
<%@ Page Language="VB" %>
<!DOCTYPE html>
<html>
<head>
<script language="VB" runat="server">
Function GetDiscountRate(ByVal price As Double, ByVal days As Integer) As Integer
If days > 70 Then
Return 70
ElseIf days > 50 Then
Return 50
ElseIf days > 30 Then
Return 35
Else
Return 15
End If
End Function
Function CalculateMarketPrice(ByVal price As Double, ByVal Optional rate As Integer = 0.00) As Integer
Dim discountAmt As Double
Dim markedPrice As Double
If rate = 0.00 Then
Return 0.00
Else
Return price * rate / 100.0
End If
End Function
Sub btnCreateRecordClick(ByVal sender As Object, ByVal e As EventArgs)
Dim unitPrice As Double
Dim markedPrice As Double
Dim discountRate As Double
Dim daysInStore As Integer
Dim discountedAmount As Double
markedPrice = 0.00
daysInStore = CInt(txtDaysInStore.Text)
unitPrice = CDbl(txtUnitPrice.Text)
discountRate = GetDiscountRate(unitPrice, daysInStore)
If discountRate = 0.00 Then
discountedAmount = CalculateMarketPrice(unitPrice)
Else
discountedAmount = CalculateMarketPrice(unitPrice, discountRate)
End If
markedPrice = unitPrice - discountedAmount
lblItemNumber.Text = txtItemNumber.Text
lblItemName.Text = txtItemName.Text
LblDiscountAmount.Text = FormatCurrency(discountedAmount)
lblMarkedPrice.Text = FormatCurrency(markedPrice)
End Sub
</script>
<title>Department Store - Inventory Creation</title>
</head>
<body>
<div align="center">
<form id="frmStoreItem" runat="server">
<h3>Department Store - Inventory Creation</h3>
<table>
<tr>
<td>Item #:</td>
<td><asp:TextBox id="txtItemNumber" runat="server" /></td>
</tr>
<tr>
<td>Item Name:</td>
<td><asp:TextBox id="txtItemName" runat="server" /></td>
</tr>
<tr>
<td>Unit Price:</td>
<td><asp:TextBox id="txtUnitPrice" runat="server" /></td>
</tr>
<tr>
<td>Days in Store:</td>
<td><asp:TextBox id="txtDaysInStore" runat="server" /></td>
</tr>
<tr>
<td> </td>
<td><asp:Button id="btnCreateSaleRecord"
text="Create Sale Record"
OnClick="btnCreateRecordClick"
runat="server"></asp:Button> </td>
</tr>
</table>
<h3>Department Store - Store Inventory</h3>
<table>
<tr>
<td>Item #:</td>
<td><asp:Label id="lblItemNumber" runat="server" /></td>
</tr>
<tr>
<td>Item Name:</td>
<td><asp:Label id="lblItemName" style="width: 250px;" runat="server" /></td>
</tr>
<tr>
<td>Discount Amouont:</td>
<td><asp:Label id="LblDiscountAmount" runat="server" /></td>
</tr>
<tr>
<td>Marked Price:</td>
<td><asp:Label id="lblMarkedPrice" runat="server" /></td>
</tr>
</table>
</form>
</div>
</body>
</html>
Here is an example of using the webpage
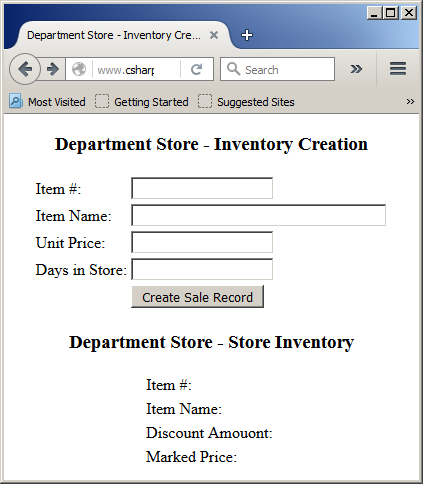


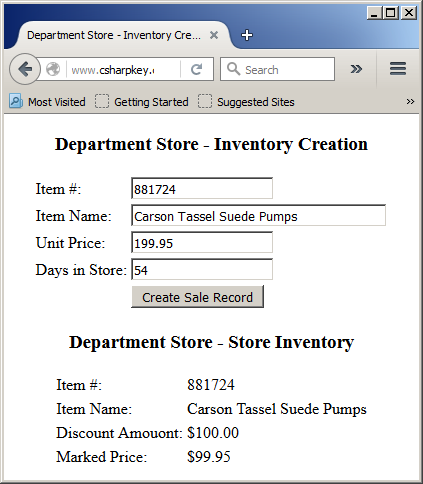
Introduction to Built-In Boolean Functions
Introduction
The Visual Basic language contains many functions that can assist you in dealing with conditional statements. Also many classes have built-in methods that produce a Boolean value. For example, the class of every object used in Visual Basic is equipped with a method named Equals. It can be used to find out if one object or value is equal to another.
If a Value is Numeric
Remember that any value that a visitor enters in a text box is primarily considered as text. Before using or converting a value that is supposed to be numeric, to let you checke whether it is a number, Visual Basic provides a function named IsNumeric. This function takes one argument as the value to check. If the argument is an integer or a floating-point number, the function returns True. If not, it returns False. Here is an example:
<%@ Page Language="VB" %>
<!DOCTYPE html>
<html>
<head>
<script runat="server">
Sub btnEvaluateClick(ByVal sender As Object, ByVal e As EventArgs)
Dim creditScore As Integer
Dim hasGoodCredit As Boolean
If IsNumeric(txtCreditScore.Text) Then
creditScore = CInt(txtCreditScore.Text)
Else
creditScore = 500
txtCreditScore.Text = "500"
End If
hasGoodCredit = (creditScore >= 680)
lblMessage.Text = "The applicant has good credit: " & CStr(hasGoodCredit) & "."
End Sub
</script>
<title>Loan Evaluation</title>
</head>
<body>
<form id="frmGrade" runat="server">
<h3>Loan Evaluation</h3>
<p>Enter Customer Credit Score:
<asp:TextBox id="txtCreditScore" style="width: 60px"
runat="server" />
<asp:Button id="btnEvaluate"
text = "Evaluate"
runat="server" OnClick="btnEvaluateClick"></asp:Button></p>
<p><asp:Label id="lblMessage" runat="server" /></p>
</form>
</body>
</html>
Here is an example of using the webpage:


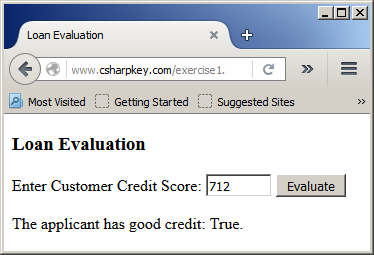
If an Object Is Nothing
When you declare a variable, especially when you create an object (declaring a variable from a class), you should initialize, but you can declare a variable without immediately initializing. This technique creates the risk of using a variable that has not yet been initializing, which can cause errors sometimes. To let you find out if a variable has been previously initialized or whether the variable currently holds a significant value, the Visual Basic library provides a function named IsNothing. This function takes one argument as the object or the expression to check. The IsNothing() function produces a True result if the argument holds a value. Otherwise, it returns False.
If a Variable is a Reference Type
As you probably know already, when you declare a variable from a class, the variable is stored in an area of computer referred to as the heap. Such a variable is referred to as a reference type. At any time, to let you check whether a variable is a reference type, Visual Basic provides a function named IsReference. This function takes one argument that is the object or an expression to check.
The Condition-Based Function
To assist you with checking a condition and its alternative,
the Visual Basic language provides a function named IIf. This function operates like an If...Then...Else conditional statement. It takes three required arguments and returns a result of a type of your choice (actually an Object). It can be presented as follows:
Public Function IIf(ByVal Expression As Boolean,
ByVal TruePart As Object,
ByVal FalsePart As Object) As Object
The condition to check, a Boolean expression, is passed as the first argument:
- If that Expression is true, the function returns the value of the second argument, the TruePart. The third argument or last argument is ignored
- If the Expression is false, the first argument is ignored and the function returns the value of the second argument
Here is an example:
<%@ Page Language="VB" %>
<!DOCTYPE html>
<html>
<head>
<script runat="server">
Sub btnEvaluateClick(ByVal sender As Object, ByVal e As EventArgs)
Dim decision As String
Dim creditScore As Integer
Dim hasGoodCredit As Boolean
creditScore = CInt(txtCreditScore.Text)
hasGoodCredit = (creditScore >= 680)
decision = IIf(hasGoodCredit = True, "is approved", "has been denied")
lblMessage.Text = "Decision: The loan " & decision & "."
End Sub
</script>
<title>Loan Evaluation</title>
</head>
<body>
<form id="frmGrade" runat="server">
<h3>Loan Evaluation</h3>
<p>Enter Customer Credit Score:
<asp:TextBox id="txtCreditScore" style="width: 60px"
runat="server" />
<asp:Button id="btnEvaluate"
text = "Evaluate"
runat="server" OnClick="btnEvaluateClick"></asp:Button></p>
<p>
<asp:Label id="lblMessage" runat="server" /></p>
</form>
</body>
</html>
Here is an example of using the webpage:


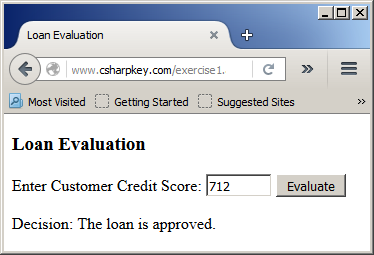
|