 |
Data Reading and Formatting |
|
|
When interacting with a web page, if it contains a web form
that requests some values, the user may be asked to enter them in the available
controls. Consider the following web page:
|
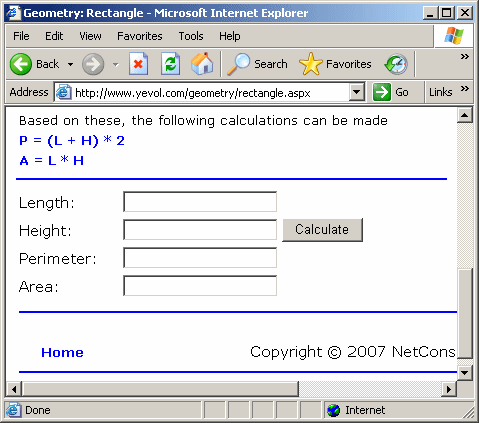
In this case, a visitor is expected to provide the length
and the height of a rectangle. The visitor would then submit those values to you
so you can calculate the perimeter and the area.
Practical
Learning: Introducing Data Reading
|
|
- Start Microsoft Visual
Studio or Microsoft Visual Web Developer
- To create a web site, on the
main menu, click File -> New -> Web Site... (or File -> New Web
Site)
-
Make sure the Language combo box is set to Visual C#.
Click the combo box on the left side of Browse, press End, and press Delete
a few times to delete the default name of the web site
- Change it to geometry4
- Click OK
- In the Solution Explorer, right-click C:\...\geometry4\ and click New
Folder
- Type images as the name of the new folder and press Enter
- Copy each of the following pictures to your My Documents folder:
- On the main menu, click Web Site -> Add Existing Item...
- Locate the folder where you saved the pictures, select each and press
Enter
- In the Solution Explorer, right-click Default.aspx and click Rename
- Change the name to index.aspx and press Enter
- To create a CSS file, on the main menu, click Web Site -> Add New
Item...
- In the Templates list, click Style Sheet
- Set the name to geometry and press Enter
- Enter the following code in the file:
body
{
font-size: 11pt;
color: black;
font-family: Verdana, Tahoma, Arial, Sans-Serif;
}
hr { color: #0000ff }
.maintitle
{
color: blue;
font-family: 'Times New Roman' , Garamond, Georgia, Serif;
text-align: center;
font-size: 24pt;
font-weight: bold;
text-decoration: none;
}
.parajust
{
font-family: Verdana, Tahoma, Arial, Sans-Serif;
font-size: 10pt;
font-weight: normal;
text-align: justify;
}
.centeredtext
{
font-family: Verdana, Tahoma, Arial, Sans-Serif;
font-size: 10pt;
font-weight: normal;
text-align: center;
}
.centered
{
text-align: center;
}
.formula
{
font-weight: bold;
font-size: 10pt;
color: blue;
font-family: Verdana, Helvetica, Tahoma, Sans-Serif;
}
a:link {
font-family: Verdana, Helvetica, Arial, Sans Serif;
color: #0000FF;
font-size: 10pt;
font-weight: bold;
text-decoration: none }
a:alink {
font-family: Verdana, Helvetica, Arial, Sans Serif;
color: #FF00FF;
font-size: 10pt;
font-weight: bold;
text-decoration: none }
a:visited
{
font-family: Verdana, Helvetica, Arial, Sans Serif;
color: Green;
font-size: 10pt;
font-weight: bold;
text-decoration: none;
}
a:hover
{
font-family: Verdana, Helvetica, Arial, Sans Serif;
color: #FF0000;
font-size: 10pt;
font-weight: Bold;
text-decoration: none;
}
- To add a new web page, on the main menu, click Web Site -> Add New
Item...
- Make sure Web Form is selected.
Set the Name to rectangle and click Add
- Change the file as follows:
<%@ Page Language="C#"
AutoEventWireup="true"
CodeFile="rectangle.aspx.cs"
Inherits="rectangle" %>
<!DOCTYPE html PUBLIC "-//W3C//DTD XHTML 1.0 Transitional//EN"
"http://www.w3.org/TR/xhtml1/DTD/xhtml1-transitional.dtd">
<html xmlns="http://www.w3.org/1999/xhtml" >
<head runat="server">
<link rel="Stylesheet" type="text/css" href="geometry.css" />
<title>Geometry: Rectangle</title>
</head>
<body>
<form id="frmRectangle"
runat="server"
method="get"
action="rectangle.aspx">
<div>
<table border="0" style="width: 660px">
<tr>
<td style="width: 100%">
<p class="maintitle">Geometry: Rectangle</p>
</td>
</tr>
</table>
<table border="0" width="660">
<tr>
<td>
<p class="parajust">A rectangle is a geometric figure made
of 4 sides connected to produce four right angles. The
horizontal measurement of the rectangle, the base, is its
length. The vertical measurement of the figure is referred
to as its height. In geometry, a rectangle can be
represented as follows:</p>
<p class="centered">
<img src="images/rectangle.gif" alt="Rectangle" />
</p>
<p class="parajust">To process a rectangle, you usually
must provide, or must be given, the length and the height.
You can then calculate the perimeter and the area. To
assist you with these calculations, you can use some
pre-established formulas. Suppose the values are
represented as follows:</p>
</td>
</tr>
</table>
<table style="width: 660px">
<tr>
<td style="width: 660px">
<strong>L: length</strong>
</td>
</tr>
<tr>
<td style="width: 660px">
<strong>H: height</strong>
</td>
</tr>
<tr>
<td style="width: 660px">
<strong>P: perimeter</strong>
</td>
</tr>
<tr>
<td style="width: 660px">
<strong>A: area</strong>
</td>
</tr>
</table>
<table border="0" style="width: 660px">
<tr>
<td style="width: 100%">
<p class="parajust">Based on these, the following
calculations can be made</p>
</td>
</tr>
<tr>
<td style="width: 100%">
<p class="formula">P = (L + H) * 2</p>
</td>
</tr>
<tr>
<td style="width: 100%">
<p class="formula">A = L * H</p>
</td>
</tr>
</table>
<hr />
<table>
<tr>
<td style="width: 100px">
<asp:Label ID="Label1"
runat="server"
Text="Length:">
</asp:Label></td>
<td style="width: 121px">
<asp:TextBox ID="txtLength"
runat="server">
</asp:TextBox></td>
<td style="width: 100px">
</td>
</tr>
<tr>
<td style="width: 100px">
<asp:Label ID="Label2"
runat="server"
Text="Height:">
</asp:Label></td>
<td style="width: 121px">
<asp:TextBox ID="txtHeight"
runat="server">
</asp:TextBox></td>
<td style="width: 100px">
<asp:Button ID="btnCalculate"
runat="server"
Text="Calculate" /></td>
</tr>
<tr>
<td style="width: 100px">
<asp:Label ID="Label3"
runat="server"
Text="Perimeter:">
</asp:Label></td>
<td style="width: 121px">
<asp:TextBox ID="txtPerimeter"
runat="server">
</asp:TextBox></td>
<td style="width: 100px">
</td>
</tr>
<tr>
<td style="width: 100px">
<asp:Label ID="Label4"
runat="server"
Text="Area:">
</asp:Label></td>
<td style="width: 121px">
<asp:TextBox ID="txtArea"
runat="server">
</asp:TextBox></td>
<td style="width: 100px">
</td>
</tr>
</table>
<table border="0" width="660">
<tr>
<td colspan="2">
<hr />
</td>
</tr>
<tr>
<td align="center" style="height: 18px">
<a href="index.aspx">Home</a>
</td>
<td align="center" style="height: 18px">
<p class="centered">Copyright © 2012 NetConsulting, Inc.</p>
</td>
</tr>
<tr>
<td colspan="2">
<hr />
</td>
</tr>
</table>
</div>
</form>
</body>
</html>
|
- To add a new web page, on the main menu, click Web Site -> Add New
Item...
- Make sure Web Form is selected.
Set the Name to square and click Add
- Change the file as follows:
<%@ Page Language="C#"
AutoEventWireup="true"
CodeFile="square.aspx.cs"
Inherits="square" %>
<!DOCTYPE html PUBLIC "-//W3C//DTD XHTML 1.0 Transitional//EN"
"http://www.w3.org/TR/xhtml1/DTD/xhtml1-transitional.dtd">
<html xmlns="http://www.w3.org/1999/xhtml" >
<head runat="server">
<link rel="Stylesheet" type="text/css" href="geometry.css" />
<title>Geometry: Square</title>
</head>
<body>
<form id="frmSquare" runat="server" method="post">
<div>
<table border="0" style="width: 660px">
<tr>
<td style="width: 100%">
<p class="maintitle">Geometry: Square</p>
</td>
</tr>
</table>
<table border="0" width="660">
<tr>
<td>
<p class="parajust">A square is a type of
<a href="rectangle.aspx">rectangle</a>
for which all 4 sides are equal. This means that the horizontal
measurement, the base or length and the height are equal. The
length of each side is then called the side of the square. A
square can be represented as follows:</p>
<p class="centered"><img src="images/square.gif" alt="Square" />
</p>
<p class="parajust">To process a square, you must have the
measure of the side. This would allow you to calculate the
perimeter and the area. To do this, you can use simple
geometric formulas. Consider the following:</p>
</td>
</tr>
</table>
<table>
<tr>
<td style="width: 660px">
<strong>S: side</strong>
</td>
</tr>
<tr>
<td style="width: 660px">
<strong>P: perimeter</strong>
</td>
</tr>
<tr>
<td style="width: 660px">
<strong>A: area</strong>
</td>
</tr>
</table>
<table border="0" style="width: 660px">
<tr>
<td style="width: 100%">
<p class="parajust">To calculate the values of a square, you can
use the following formulas:</p></td>
</tr>
<tr>
<td style="width: 100%">
<p class="formula">P = S * 4</p>
</td>
</tr>
<tr>
<td style="width: 100%">
<p class="formula">A = S * S</p>
</td>
</tr>
</table>
<hr />
<table>
<tr>
<td style="width: 100px">
<asp:Label ID="Label1"
runat="server"
Text="Side:"></asp:Label></td>
<td style="width: 121px">
<asp:TextBox ID="txtSide"
runat="server"></asp:TextBox></td>
<td style="width: 100px">
<asp:Button ID="btnCalculate"
runat="server"
Text="Calculate" /></td>
</tr>
<tr>
<td style="width: 100px">
<asp:Label ID="Label2"
runat="server"
Text="Perimeter"></asp:Label></td>
<td style="width: 121px">
<asp:TextBox ID="txtPerimeter"
runat="server">
</asp:TextBox></td>
<td style="width: 100px"></td>
</tr>
<tr>
<td style="width: 100px">
<asp:Label ID="Label3"
runat="server"
Text="Area:"></asp:Label></td>
<td style="width: 121px">
<asp:TextBox ID="txtArea"
runat="server">
</asp:TextBox></td>
<td style="width: 100px"></td>
</tr>
</table>
</div>
</form>
<table border="0" width="660">
<tr>
<td colspan="2">
<hr />
</td>
</tr>
<tr>
<td align="center">
<a href="index.aspx">
<strong><span style="font-size: 10pt">Home</span></strong>
</a>
</td>
<td align="center">
<p class="centered">Copyright © 2012 NetConsulting, Inc.</p>
</td>
</tr>
<tr>
<td colspan="2">
<hr />
</td>
</tr>
</table>
</body>
</html>
|
- Save the files
Everything the user types is primarily considered a string.
Therefore, if you want to get a number from the user, first get the string that
represents the value. Here is an example:
<%@ Page Language="C#" %>
<html>
<head>
<script runat="server">
private void Calculate()
{
string strLength;
strLength = txtLength.Text;
}
</script>
<title>Exercise</title>
</head>
<body>
<form id="frmExercise" method="post" runat="server">
<table>
<tr>
<td>Length:</td>
<td>
<asp:TextBox id="txtLength" AutoPostBack="True" runat="server">
</asp:TextBox>
</td>
<tr>
</table>
</form>
</body>
</html>
After getting the string, you must convert it to a number.
To perform this conversion, each data type of the .NET Framework is a class in
its own right. Each of these classes is equipped with a method named Parse. To use
the Parse() method, pass the string that you got from the user. Here is
an example:
<script runat="server">
private void Calculate()
{
string strLength;
double length = 0.00;
strLength = txtLength.Text;
Length = double.Parse(strLength);
}
</script>
An advanced but faster way to do this is to type the string
value in the parentheses of Parse. This has the same effect. Here is an
example:
<script runat="server">
private void Calculate()
{
double length = 0.00;
Length = double.Parse(txtLength.Text);
}
</script>
Practical
Learning: Reading Numeric Values
|
|
- If necessary, click the square.aspx tab to access its file.
In the lower-left section of the window, click the Design button and, on the
form, double-click the Calculate button
- To retrieve various numbers from the user, change the file as follows:
using System;
using System.Data;
using System.Configuration;
using System.Collections;
using System.Web;
using System.Web.Security;
using System.Web.UI;
using System.Web.UI.WebControls;
using System.Web.UI.WebControls.WebParts;
using System.Web.UI.HtmlControls;
public partial class square : System.Web.UI.Page
{
protected void Page_Load(object sender, EventArgs e)
{
}
protected void btnCalculate_Click(object sender,
EventArgs e)
{
double side = 0.00;
double perimeter, area;
side = double.Parse(txtSide.Text);
perimeter = side * 4;
area = side * side;
}
}
|
- Click the rectangle.aspx tab to access its file.
In the lower-left section of the window, click the Design button and, on the
form, double-click the Calculate button
- To retrieve various numbers from the user, change the file as follows:
using System;
using System.Data;
using System.Configuration;
using System.Collections;
using System.Web;
using System.Web.Security;
using System.Web.UI;
using System.Web.UI.WebControls;
using System.Web.UI.WebControls.WebParts;
using System.Web.UI.HtmlControls;
public partial class rectangle : System.Web.UI.Page
{
protected void Page_Load(object sender, EventArgs e)
{
}
protected void btnCalculate_Click(object sender, EventArgs e)
{
double length = 0.00;
double height = 0.00;
double perimeter;
double area;
length = double.Parse(txtLength.Text);
height = double.Parse(txtHeight.Text);
perimeter = (length + height) * 2;
area = length * height;
}
}
|
- Sale the files
Requesting Dates and Times
|
|
As done with the regular numbers, you can request a date
value from the user. This is also done by requesting a string from the user.
After the user has entered the string, you can then convert it to a DateTime
value. Here is an example:
<%@ Page Language="C#" %>
<html>
<head>
<script runat="server">
private void CheckTimeSheet()
{
DateTime StartDate;
StartDate = DateTime.Parse(txtStartDate.Text);
}
</script>
<title>Exercise</title>
</head>
<body>
<form id="frmExercise" method="post" runat="server">
<table>
<tr>
<td>Start Date:</td>
<td>
<asp:TextBox id="txtStartDate" AutoPostBack="True" runat="server">
</asp:TextBox>
</td>
<tr>
</table>
</form>
</body>
</html>
Just like any value you request from the user, a date or time value that
the user types must be valid, otherwise, the program would produce an error.
Because dates and times follow some rules for their formats, you should strive
to let the user know how you expect the value to be entered.
|
|