If you create a list whose number of items must be constant,
the user cannot add items beyond the maximum allowed number. Therefore, before
adding an item, you can first check whether the collection has a fixed size or
not. To give you this information, the IList interface is equipped with
a Boolean read-only property named IsFxedSize. This property simply lets
the user know whether the collection has a fixed number of items.
Here is an example of implementing this property:
public ref class CObjects : public IList
{
private:
int items;
array<Object ^> ^ objects;
public:
. . . No Change
virtual property bool IsFixedSize
{
bool get() { return false; }
}
virtual void CopyTo(Array ^, int);
virtual IEnumerator ^ GetEnumerator(void);
};
Practical
Learning: Implementing the IsFixedSize Property
|
|
- Access the Properties.h header file and add the following property:
#pragma once
#include "RentalProperty.h"
using namespace System;
using namespace System::Collections;
public ref class CProperties : public IList
{
private:
array<Object ^> ^ RentalProperties;
int counter;
public:
. . . No Change
virtual property bool IsFixedSize
{
bool get() { return false; }
}
virtual void CopyTo(Array ^, int);
virtual IEnumerator ^ GetEnumerator(void);
};
|
Most collections are meant to receive new items. If you
want, you can create a collection that cannot receive new values. To support
this, the IList interface is equipped with the Boolean IsReadOnly
property. If a collection is read-only, it would prevent the clients from
adding items to it.
Here is an example of implementing the IsReadOnly property:
public ref class CObjects : public IList
{
private:
int items;
array<Object ^> ^ objects;
public:
. . . No Change
virtual property bool IsReadOnly
{
bool get() { return false; }
}
. . . No Change
};
Practical
Learning: Implementing the IsReadOnly Property
|
|
- In the Properties.h header file, add the following property:
#pragma once
#include "RentalProperty.h"
using namespace System;
using namespace System::Collections;
public ref class CProperties : public IList
{
private:
array<Object ^> ^ RentalProperties;
int counter;
public:
. . . No Change
virtual property bool IsReadOnly
{
bool get() { return false; }
}
virtual void CopyTo(Array ^, int);
virtual IEnumerator ^ GetEnumerator(void);
};
|
- In the Class View, right-click CProperties -> Add ->
Function...
- Accept the Return Type as int and set the Function Name to
Add
- In the Parameter Type, type Object ^
- In the Parameter Name, type value and click Add
- Click the virtual check box
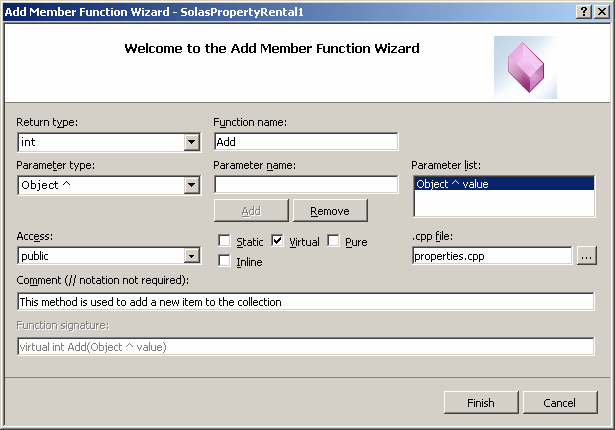
- Click Finish
- In the Class View, right-click CProperties -> Add ->
Function...
- Set the Return Type to void
- Set the Function Name to insert
- In the Parameter Type, select int
- In the Parameter Name, type index and click Add
- In the Parameter Type, type Object ^
- In the Parameter Name, type value and click Add
- Click the virtual check box
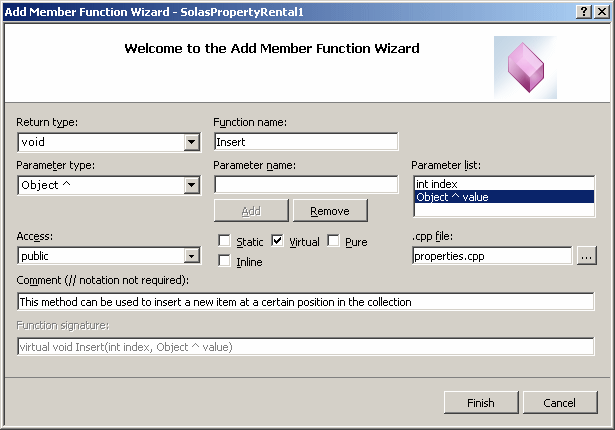
- Click Finish
- Open the Properties.h header file and add the following property
#pragma once
#include "RentalProperty.h"
using namespace System;
using namespace System::Collections;
public ref class CProperties : public IList
{
private:
array<Object ^> ^ RentalProperties;
int counter;
public:
. . . No Change
virtual property Object ^ default[int]
{
Object ^ get(int index) { return nullptr; }
void set(int index, Object ^ value) { }
}
virtual void CopyTo(Array ^, int);
virtual IEnumerator ^ GetEnumerator(void);
. . . No Change
};
|
- In the Class View, right-click CProperties -> Add ->
Function...
- Set the Return Type to bool
- Set the Function Name to Contains
- In the Parameter Type, type Object ^
- In the Parameter Name, type value and click Add
- Click the virtual check box

- Click Finish
- In the Class View, right-click CProperties -> Add ->
Function...
- Accept the Return Type as int and set the Function Name to IndexOf
- In the Parameter Type, type Object ^
- In the Parameter Name, type value and click Add
- Click the virtual check box
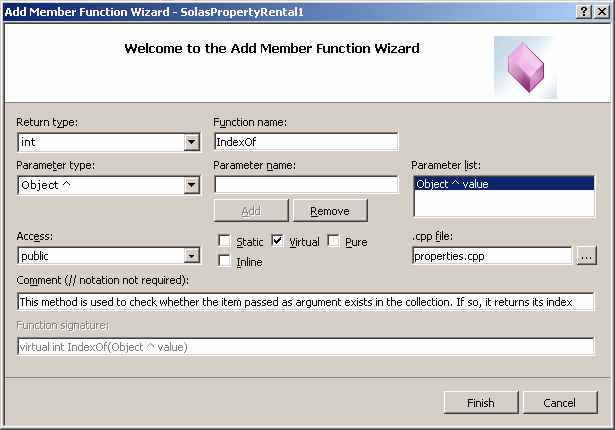
- Click Finish
- In the Class View, right-click CProperties -> Add ->
Function...
- Set the Return Type to void
- Set the Function Name to RemoveAt
- In the Parameter Type, select int
- In the Parameter Name, type index and click Add
- Click the virtual check box
- Click Finish
- In the Class View, right-click CProperties -> Add ->
Function...
- Set the Return Type to void
- Set the Function Name to Remove
- In the Parameter Type, select Object ^
- In the Parameter Name, type value and click Add
- Click the virtual check box
- Click Finish
- In the Class View, right-click CProperties -> Add ->
Function...
- Set the Return Type to void
- Set the Function Name to Clear
- Click the virtual check box
- Click Finish
#pragma once
#include "RentalProperty.h"
using namespace System;
using namespace System::Collections;
public ref class CProperties : public IList
{
private:
array<Object ^> ^ RentalProperties;
int counter;
public:
CProperties(void);
virtual property int Count
{
int get() { return counter; }
}
virtual property bool IsSynchronized
{
bool get() { return false; }
}
virtual property Object ^ SyncRoot
{
Object ^ get() { return this; }
}
virtual property bool IsFixedSize
{
bool get() { return false; }
}
virtual property bool IsReadOnly
{
bool get() { return false; }
}
virtual property Object ^ default[int]
{
Object ^ get(int index) { return nullptr; }
void set(int index, Object ^ value) { }
}
virtual void CopyTo(Array ^, int);
virtual IEnumerator ^ GetEnumerator(void);
// This method is used to add a new item to the collection
virtual int Add(Object ^ value);
// This method can be used to insert an item at
// a certain position inside the collection
virtual void Insert(int index, Object ^ value);
// This method is used to find out whether the item
// passed as argument exists in the collection
virtual bool Contains(Object ^ value);
// This method is used to check whether the item passed as
// argument exists in the collection. If so, it returns its index
virtual int IndexOf(Object ^ value);
// This method is used to delete the item positioned
// at the index passed as argument
virtual void RemoveAt(int index);
// This method first checks the existence of the item passed
// as argument. If the item exists, the method deletes it
virtual void Remove(Object ^ value);
// This methods deletes all items from the collection
virtual void Clear(void);
};
|
|
|