- Start Microsoft SQL Server Enterprise Manager or the SQL Query Analyzer
- Create a new database named SuperMarket
- Create a new table named Employees as follows:

- Fill it up with a few records as follows:
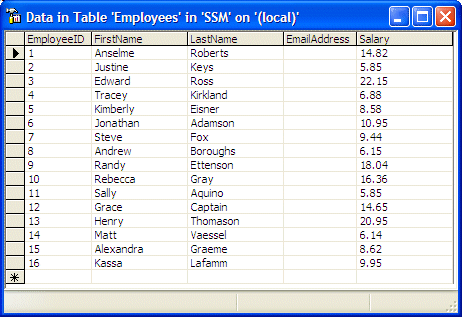
- Close the table
- In SQL Query Analyzer, open a new blank window and create a new procedure
by typing the following code:
-- =============================================
-- Procedure: CreateEmailAddress
-- Creates an email address for each employee
-- =============================================
IF EXISTS (SELECT name
FROM sysobjects
WHERE name = N'CreateEmailAddress'
AND type = 'P')
DROP PROCEDURE CreateEmailAddress
GO
CREATE PROCEDURE CreateEmailAddress
AS
UPDATE Employees
SET EmailAddress = LOWER(LastName) +
LOWER(LEFT(FirstName, 1)) +
'@supermarket.com'
FROM Employees
GO
|
- Execute the statement (F5)
- Start Visual C++ .NET and create a new Windows Forms Application named SuperMarket1
- In Server Explorer, expand the server that holds the above database and
the database itself followed by its Tables node
- Drag Employees to the form
- On the main menu, click Data -> Generate Dataset...
- Change the name of the dataset to dsEmployees and click OK
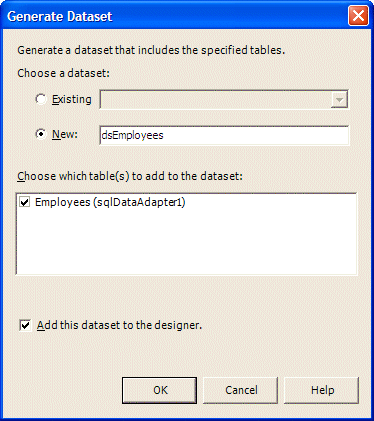
- Add a DataGrid control to the form as follows:
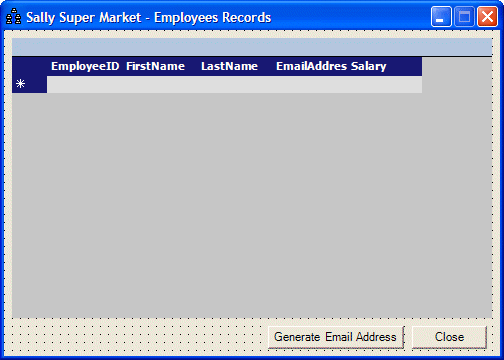 |
Control |
Name |
Text |
Other Properties |
Form |
|
|
StartPosition: CenterScreen |
DataGrid |
|
|
Anchor: Top, Bottom, Left, Right
AutoFormat: Professional 3
DataSource: dsEmployees1.Employees |
Button |
btnEmailAddr |
Generate Email Addresses |
Anchor: Bottom, Right |
Button |
btnClose |
Close |
Anchor: Bottom, Right |
|
- Double-click an empty the form to access its Load event
- Return to the form and double-click the Close button
- Implement the events as follows:
private: System::Void Form1_Load(System::Object * sender, System::EventArgs * e)
{
this->sqlDataAdapter1->Fill(this->dsEmployees1);
}
private: System::Void btnClose_Click(System::Object * sender, System::EventArgs * e)
{
Close();
}
|
- Test the application then close the form to return to Visual C++ .NET
- Double-click the Generate Email Addresses button and implement its event
as follows:
#pragma once
namespace SuperMarket1
{
using namespace System;
using namespace System::ComponentModel;
using namespace System::Collections;
using namespace System::Windows::Forms;
using namespace System::Data;
using namespace System::Drawing;
using namespace System::Data::SqlClient;
/// <summary>
/// Summary for Form1
///
/// WARNING: If you change the name of this class, you will need to change the
/// 'Resource File Name' property for the managed resource compiler tool
/// associated with all .resx files this class depends on. Otherwise,
/// the designers will not be able to interact properly with localized
/// resources associated with this form.
/// </summary>
public __gc class Form1 : public System::Windows::Forms::Form
{
public:
Form1(void)
{
InitializeComponent();
}
private:
/// <summary>
/// Required designer variable.
/// </summary>
System::ComponentModel::Container * components;
/// <summary>
/// Required method for Designer support - do not modify
/// the contents of this method with the code editor.
/// </summary>
void InitializeComponent(void)
{
. . .
}
private: System::Void Form1_Load(System::Object * sender, System::EventArgs * e)
{
this->sqlDataAdapter1->Fill(this->dsEmployees1);
}
private: System::Void btnClose_Click(System::Object * sender, System::EventArgs * e)
{
Close();
}
private: System::Void btnEmailAddr_Click(System::Object * sender, System::EventArgs * e)
{
// Create a new SQL command command, passing it the name of
// the stored procedure we want to call and attaching it to
// the existing SQL connection
SqlCommand* cmdNew = new SqlCommand(S"CreateEmailAddress", this->sqlConnection1);
// Create a new adapter and initialize it with the new SQL command
SqlDataAdapter *sdaNew = new SqlDataAdapter(cmdNew);
// Create a new data set
DataSet * dsEmployees = new DataSet();
// We need to specify the type of command we want to use.
// In this case, it will be a SQL Server stored procedure
cmdNew->CommandType = CommandType::StoredProcedure;
// It is time to update the data set with information from the data adapter
sdaNew->Fill(dsEmployees, S"Employees");
this->sqlDataAdapter1->Fill(this->dsEmployees1, S"Employees");
// Let the user know that the assignment has been carried
MessageBox::Show(S"An email address has been created for each employees");
}
};
}
|
- Test the application:
|