A static library is a library that you can create
independent of an application and use it in your program. It can be used
to extend the library of functions of a library you use in your
programming language. For example, Visual C++ (whether MFC or .Net) is significantly limited as
compared to Visual Basic in the areas of mathematics, finance, data
validation functions. Creating your own library can help reduce your work when
facing such assignments.
Creating a static is extremely easy. The problem you
would have is deciding what to put in the library.
- Start Microsoft Visual C++. On the main menu, click File -> new
Project...
- In the New Project dialog box, select Visual C++ Projects in the
Project Types list
- In the Templates list, click Empty Project (.Net)
- In the Project Name, type a name such as ExtLib and specify your desired folder in the Location
box
- Click OK
- To add a new form, on the main menu, click Project -> Add New
Item...
- In the Templates list of the Add New Item dialog box, click Windows
Form (.Net)
- In the Name edit box, type inputbox and press Enter
- Design the dialog box as follows:
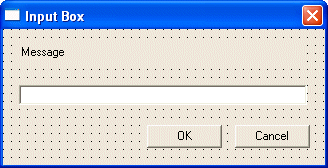 |
Control |
Name |
Text |
Additional Properties |
Form |
inputbox |
Input Box |
FormBorderStyle: FixedDialog
MaximizeBox: False
MinimizeBox: False |
Label |
lblMessage |
Message |
|
Text Box |
txtResult |
|
|
|
- Save All
- Right-click the form and click View Code
- Include a public Get method that can return the contents of
the text box of the form and, at the end of the header file, declare a
function as follows:
#pragma once
using namespace System;
using namespace System::ComponentModel;
using namespace System::Collections;
using namespace System::Windows::Forms;
using namespace System::Data;
using namespace System::Drawing;
namespace ExtLib
{
/// <summary>
/// Summary for inputbox
///
/// WARNING: If you change the name of this class, you will need to change the
/// 'Resource File Name' property for the managed resource compiler tool
/// associated with all .resx files this class depends on. Otherwise,
/// the designers will not be able to interact properly with localized
/// resources associated with this form.
/// </summary>
public __gc class frmInputBox : public System::Windows::Forms::Form
{
public:
frmInputBox(void)
{
InitializeComponent();
}
protected:
void Dispose(Boolean disposing)
{
if (disposing && components)
{
components->Dispose();
}
__super::Dispose(disposing);
}
private: System::Windows::Forms::Label * lblMessage;
private: System::Windows::Forms::TextBox * txtResult;
private: System::Windows::Forms::Button * btnCancel;
private: System::Windows::Forms::Button * btnOK;
private:
/// <summary>
/// Required designer variable.
/// </summary>
System::ComponentModel::Container* components;
/// <summary>
/// Required method for Designer support - do not modify
/// the contents of this method with the code editor.
/// </summary>
void InitializeComponent(void)
{
this->lblMessage = new System::Windows::Forms::Label();
this->txtResult = new System::Windows::Forms::TextBox();
this->btnCancel = new System::Windows::Forms::Button();
this->btnOK = new System::Windows::Forms::Button();
this->SuspendLayout();
//
// lblMessage
//
this->lblMessage->Location = System::Drawing::Point(16, 16);
this->lblMessage->Name = S"lblMessage";
this->lblMessage->Size = System::Drawing::Size(288, 23);
this->lblMessage->TabIndex = 0;
this->lblMessage->Text = S"Message";
//
// txtResult
//
this->txtResult->Location = System::Drawing::Point(16, 56);
this->txtResult->Name = S"txtResult";
this->txtResult->Size = System::Drawing::Size(288, 20);
this->txtResult->TabIndex = 1;
this->txtResult->Text = S"";
//
// btnCancel
//
this->btnCancel->DialogResult = System::Windows::Forms::DialogResult::Cancel;
this->btnCancel->Location = System::Drawing::Point(232, 96);
this->btnCancel->Name = S"btnCancel";
this->btnCancel->TabIndex = 2;
this->btnCancel->Text = S"Cancel";
//
// btnOK
//
this->btnOK->DialogResult = System::Windows::Forms::DialogResult::OK;
this->btnOK->Location = System::Drawing::Point(144, 96);
this->btnOK->Name = S"btnOK";
this->btnOK->TabIndex = 3;
this->btnOK->Text = S"OK";
//
// inputbox
//
this->AutoScaleBaseSize = System::Drawing::Size(5, 13);
this->ClientSize = System::Drawing::Size(322, 136);
this->Controls->Add(this->btnOK);
this->Controls->Add(this->btnCancel);
this->Controls->Add(this->txtResult);
this->Controls->Add(this->lblMessage);
this->FormBorderStyle = System::Windows::Forms::FormBorderStyle::FixedDialog;
this->MaximizeBox = false;
this->MinimizeBox = false;
this->Name = S"inputbox";
this->Text = S"Input Box";
this->ResumeLayout(false);
}
public:
String* GetResult(void)
{
return this->txtResult->Text;
}
};
String *InputBox(String *Msg, String *Example=0, String *Caption=0);
}
|
- Access the source file and change it as follows (if your namespace
has a different name, make sure you change it accordingly):
#include "inputbox.h"
namespace ExtLib
{
String *InputBox(String *Msg, String *Example, String *Caption)
{
frmInputBox *FIB = new frmInputBox;
if( FIB->ShowDialog() == DialogResult::OK )
return FIB->GetResult();
return 0;
}
}
|
- To save the project, on the main menu, click File -> Save All.
- To indicate that you are creating a library instead of an
executable, on the main menu, click Project -> ExtLib Properties...
- In the Property Pages, click the arrow of the Configuration Type and
click Static Library (.lib)
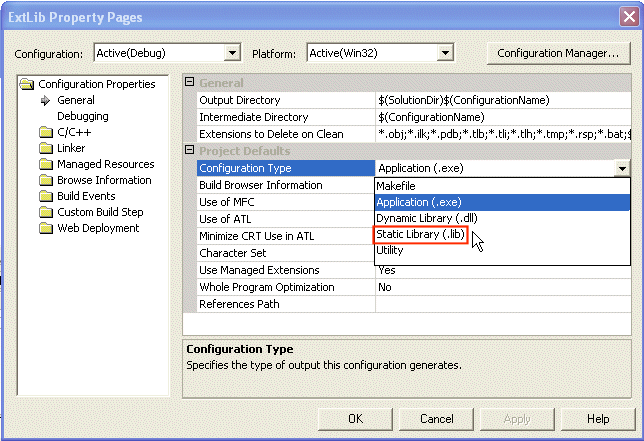
- Click OK
- To build the library, on the main menu, click Build -> Build
ExtLib.
At the end, you should receive Build: 1 succeeded, 0 failed, 0 skipped
|
|