One of the jobs performed when managing the nodes of
an XML file consists of adding a new element with its accompanying
attribute(s) to the list.
To add a new element with its attribute(s), you should
open it using the XmlDocument class to start. To actually add
element, you can use an XmlElement variable. The XmlDocument
class is equipped with the CreateElement() method that allows you
to add a new element. The XmlDocument::CreateElement() method
returns an XmlElement value that you can assign to your declared XmlElement.
Once you have created the new node, to add one or more attributes to it,
you can call the XmlElement::SetAttribute() method.
|
Practical Learning: Adding a New Element With Attributes
|
|
- Start Microsoft Visual C++ .Net and create a new Windows Forms Application
named CarInventory2
- Create a new XML file named Cars
- Complete it the following elements:
<?xml version="1.0" encoding="utf-8"?>
<carinfo>
<car Make="Ford" Model="Taurus" />
<car Make="Mazda" Model="626" />
</carinfo>
|
- Add a DataGrid control to the form
- Add a Button control to the form. Change its Text to Load
and its Name to btnLoad
- Add another Button to the form. Change its Text to Close and its Name to btnClose
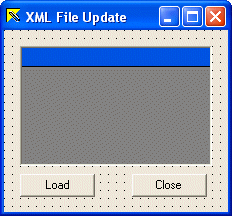
- Double-click an unoccupied area on the form
- Return to the form and double-click the Load and the Close buttons
- Implement the events as
follows:
#pragma once
namespace CarInventory2
{
using namespace System;
using namespace System::ComponentModel;
using namespace System::Collections;
using namespace System::Windows::Forms;
using namespace System::Data;
using namespace System::Drawing;
using namespace System::Xml;
/// <summary>
/// Summary for Form1
///
/// WARNING: If you change the name of this class, you will need to change the
/// 'Resource File Name' property for the managed resource compiler tool
/// associated with all .resx files this class depends on. Otherwise,
/// the designers will not be able to interact properly with localized
/// resources associated with this form.
/// </summary>
public __gc class Form1 : public System::Windows::Forms::Form
{
public:
Form1(void)
{
InitializeComponent();
}
protected:
void Dispose(Boolean disposing)
{
if (disposing && components)
{
components->Dispose();
}
__super::Dispose(disposing);
}
private: System::Windows::Forms::DataGrid * dataGrid1;
private: System::Windows::Forms::Button * btnLoad;
private: System::Windows::Forms::Button * btnClose;
private: System::Windows::Forms::GroupBox * groupBox1;
private: System::Windows::Forms::Label * label1;
private: System::Windows::Forms::TextBox * txtMake;
private: System::Windows::Forms::Label * label2;
private: System::Windows::Forms::TextBox * txtModel;
private: System::Windows::Forms::Button * btnAdd;
private:
/// <summary>
/// Required designer variable.
/// </summary>
System::ComponentModel::Container * components;
DataSet *dsCarInfo;
/// <summary>
/// Required method for Designer support - do not modify
/// the contents of this method with the code editor.
/// </summary>
void InitializeComponent(void)
. . .
private: System::Void btnLoad_Click(System::Object * sender, System::EventArgs * e)
{
dsCarInfo->ReadXml(S"Cars.xml");
this->dataGrid1->DataSource = dsCarInfo;
this->dataGrid1->DataMember = S"car";
}
private: System::Void btnClose_Click(System::Object * sender, System::EventArgs * e)
{
this->Close();
}
private: System::Void Form1_Load(System::Object * sender, System::EventArgs * e)
{
dsCarInfo = new DataSet(S"CarsInformation");
}
};
}
|
- Test the application:

- Close it and return to MSVC
- To prepare for new items, change the design of the form as follows:
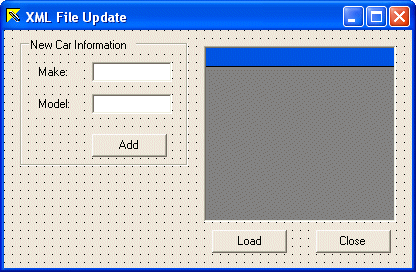
- Change the names of the new text boxes to txtMake and txtModel
- Change the name of the new button to btnAdd and double-click it
- Implement its event as follows:
private: System::Void btnAdd_Click(System::Object * sender, System::EventArgs * e)
{
// Retrieve the name of the make and the name of the model
String *strMake = this->txtMake->Text;
String *strModel = this->txtModel->Text;
// Check that there is a make car to add
if( strMake->Equals(S"") )
{
MessageBox::Show(S"No car make to add");
return;
}
// Check that there is a model car to add
if( strModel->Equals(S"") )
{
MessageBox::Show(S"No car model to add");
return;
}
// Declare an XmlDocument that will be used to add a new item
XmlDocument* XmlDoc = new XmlDocument();
try {
// Remove any item from the data set
this->dsCarInfo->Clear();
// Get the XML file and load it in the XmlDocument variable
XmlDoc->Load(S"Cars.xml");
// Create the new element
XmlElement *Elm = XmlDoc->CreateElement(S"car");
// Create its attributes
Elm->SetAttribute(S"Make", strMake);
Elm->SetAttribute(S"Model", strModel);
// Add the new element to the file...
XmlDoc->DocumentElement->AppendChild(Elm);
// ... and save the document
XmlDoc->Save(S"Cars.xml");
// Reload the XML file in the data set
dsCarInfo->ReadXml(S"Cars.xml");
// Display the new changes in the data grid
this->dataGrid1->DataSource = dsCarInfo;
this->dataGrid1->DataMember = S"car";
// Since the new items have just been added,
// we can empty the text boxes
this->txtMake->Text = S"";
this->txtModel->Text = S"";
this->txtMake->Focus();
}
catch (Exception *e)
{
MessageBox::Show(e->Message);
}
}
|
- Execute the application to test it. Then click the Load button first and
test adding a new item
|
|