 |
Example Application: Algebra |
|
|
This example explores the techniques of using the button
control. The application is used to calculate the factorial of a number, the
permuation, and the combination of two numbers.
|
In the study of statistics, a factorial is a technique
of finding different ways to arrange a series of objects (or values). For
example, imagine you have five colors as red, green, blue, white, and black.
In how many arrangements can you produce a list of those four objects? You
can get the answer by calculating the factorial of the number of objects.
The formula to calculate the factorial is:
F = n!
Imagine you have a set of five objects in different
colors as red, green, blue, white, and black. Imagine you want to arrange
the objects in different sequences but you want each sequence to start with
two specific objects, for example you may want to arrange the objects so
that you always start with any combination of black and white followed by
any combination of the other objects. This type of arrangement is called a
permutation. The formula to calculate a permutation is:

Imagine you have five objects and you want to arrange
the objects in different sequences but you want each sequence to start with
certain two objects. This type of arrangement is called a combination. To
calculate it, you can use the following formula:
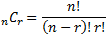
Application:
Creating the Application
|
|
- Start Microsoft Visual C# and create a Windows Application named
Algebra2
- On the main menu, click Project -> Add Class...
- In the middle list, make sure Class is selected.
Change the Name
to Algebra and click Add
- Change the file as follows:
using System;
using System.Collections.Generic;
using System.Linq;
using System.Text;
namespace Algebra2
{
public class Algebra
{
public static long Factorial(long x)
{
if (x <= 1)
return 1;
else
return x * Factorial(x - 1);
}
public static long Permutation(long n, long r)
{
if (r == 0)
return 0;
if (n == 0)
return 0;
if ((r >= 0) && (r <= n))
return Factorial(n) / Factorial(n - r);
else
return 0;
}
public static long Combination(long a, long b)
{
if (a <= 1)
return 1;
return Factorial(a) / (Factorial(b) * Factorial(a - b));
}
}
}
- In the Solution Explorer, right-click Form1.cs and click Rename
- Type Exercise.cs and press Enter twice (to save and to open
the form)
- Click the body of the form to make sure it is selected.
In the
Properties window, change the following characteristics
FormBorderStyle: FixedDialog Text: Factorial, Permutation, and
Combination Size: 304, 208 StartPosition: CenterScreen
MaximizeBox: False MinimizeBox: False
- In the Containers section of the Toolbox, click TabControl and click
the form
- On the form, right-click the right side of tabPage2 and click Add
Page
- Design the form as follows:
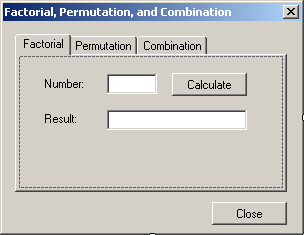 |
Control |
Text |
Name |
Additional Properties |
TabControl |
 |
|
tclAlgebra |
HotTrack: True Location: 12, 12
Size: 304, 235 |
TabPage |
|
Factorial |
tabFactorial |
|
Label |
 |
Number: |
|
Location: 22, 21 |
Button |
 |
Calculate |
btnCalcFactorial |
|
TextBox |
 |
|
txtNumber |
TextAlign: Right Location: 88, 18
Size: 50, 20 |
Label |
 |
Result: |
|
Location: 22, 56 |
TextBox |
 |
|
txtFactorial |
TextAlign: Right Location: 88, 54
Size: 140, 20 |
Button |
 |
Close |
btnClose |
|
|
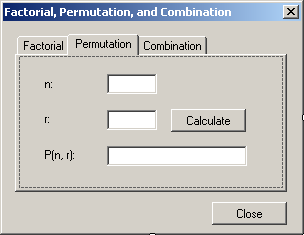 |
Control |
Text |
Name |
Location |
Size |
TabPage |
|
Permutation |
tabPermutation |
|
|
Label |
 |
n: |
|
22, 21 |
|
TextBox |
 |
|
txtPermutationN |
88, 18 |
50, 20 |
Label |
 |
r: |
|
22, 56 |
|
Button |
 |
Calculate |
btnCalcPermutation |
|
|
TextBox |
 |
|
txtPermutationR |
88, 54 |
50, 20 |
Label |
 |
P(n, r): |
|
22, 92 |
|
TextBox |
 |
|
txtPermutation |
88, 90 |
140, 20 |
|
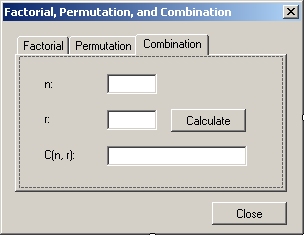 |
Control |
Text |
Name |
Location |
Size |
TabPage |
|
Combination |
tabCombination |
|
|
Label |
 |
n: |
|
22, 21 |
|
TextBox |
 |
|
txtCombinationN |
88, 18 |
50, 20 |
Label |
 |
r: |
|
22, 56 |
|
Button |
 |
Calculate |
btnCalcCombination |
|
|
TextBox |
 |
|
txtCombinationR |
88, 54 |
50, 20 |
Label |
 |
C(n, r): |
|
22, 92 |
|
TextBox |
 |
|
txtCombination |
88, 90 |
140, 20 |
|
- Access the Factorial tab page and double-click its Calculate button
- Implement the event as follows:
private void btnCalcFactorial_Click(object sender, EventArgs e)
{
long number = 0;
long result;
try
{
number = long.Parse(txtFactNumber.Text);
result = Algebra.Factorial(number);
txtFactorial.Text = result.ToString();
}
catch (FormatException)
{
MessageBox.Show("Invalid Number");
}
}
- Return to the form
- Access the Permutation tab page and double-click its Calculate
button
- Implement the event as follows:
private void btnCalcPermutation_Click(object sender, EventArgs e)
{
long n = 0, r = 0;
long result;
try
{
n = long.Parse(txtPermutationN.Text);
}
catch (FormatException)
{
MessageBox.Show("Invalid Number");
}
try
{
r = long.Parse(txtPermutationR.Text);
result = Algebra.Permutation(n, r);
txtPermutation.Text = result.ToString();
}
catch (FormatException)
{
MessageBox.Show("Invalid Number");
}
}
- Return to the form
- Access the Combination tab page and double-click its Calculate
button
- Implement the event as follows:
private void btnCalcCombination_Click(object sender, EventArgs e)
{
long n = 0, r = 0;
long result;
try
{
n = long.Parse(txtCombinationN.Text);
}
catch (FormatException)
{
MessageBox.Show("Invalid Number");
}
try
{
r = long.Parse(txtCombinationR.Text);
result = Algebra.Combination(n, r);
txtCombination.Text = result.ToString();
}
catch (FormatException)
{
MessageBox.Show("Invalid Number");
}
}
- Return to the form and double-click the Close button
- Implement the event as follows:
private void btnClose_Click(object sender, EventArgs e)
{
Close();
}
- Execute the application to test the calculations
- Close the form and return to your programming environment
|
|