 |
Windows Controls: The List Box |
|
Introduction to List Boxes |
|
|
A list box presents a list of items to choose from. Each
item displays on a line. The user makes a selection by clicking in the list.
Once clicked, the item or line on which the mouse landed becomes
highlighted, indicating that it is the current choice. Here is an example:
|
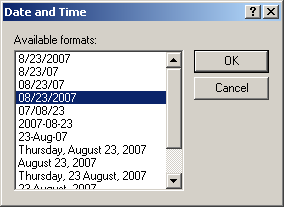
After an item has been selected, to make a different
selection, the user would click another. The new clicked item becomes
selected or highlighted; the previously selected item looses its
highlighting attribute. The user can also change the selection by pressing
the up or down arrow keys.
|
|
List boxes are categorized in two types: single and
multi-selection. The second category allows a user to select more than one
item by pressing Ctrl to select items at random or by pressing Shift to
select items in a range.
One of the main reasons for using a list box is to
display a list of items to the user. Sometimes the list would be very large.
If the list is longer than the available client area of the control, the
control would be equipped with a scroll bar that allows the user to navigate
up and down to access all items of the list. You will have the option of
deciding how many items to display on the list.
Application:
Introducing List Boxes
|
|
- Start Microsoft Visual Studio
- Create a new Windows Forms Application named
MusicalInstrumentStore1
- In the Solution Explorer, right-click Form1.cs and click Rename
- Type MusicStore.cs and press Enter
To support list boxes, the .NET Framework provides the
ListBox class. At design time, to add a list box to an application,
from the Common Controls section of the Toolbox, click the ListBox control
and click the form or the control that will host it. To programmatically
create a list box, declare a variable of type ListBox, use the new operator
to allocate memory it, and add it to the Controls property of its eventual
parent.
using System;
using System.Drawing;
using System.Windows.Forms;
public class Exercise : System.Windows.Forms.Form
{
ListBox lbxFamily;
public Exercise()
{
InitializeComponent();
}
private void InitializeComponent()
{
lbxFamily = new ListBox();
Controls.Add(lbxFamily);
}
}
public class Program
{
static int Main()
{
System.Windows.Forms.Application.Run(new Exercise());
return 0;
}
}
 |
In our applications, the names of the
list-based controls will be in plural. This is not a rule and it is
not based on any preconceived standard. |
Application:
Creating List Boxes
|
|
- Design the form as follows:
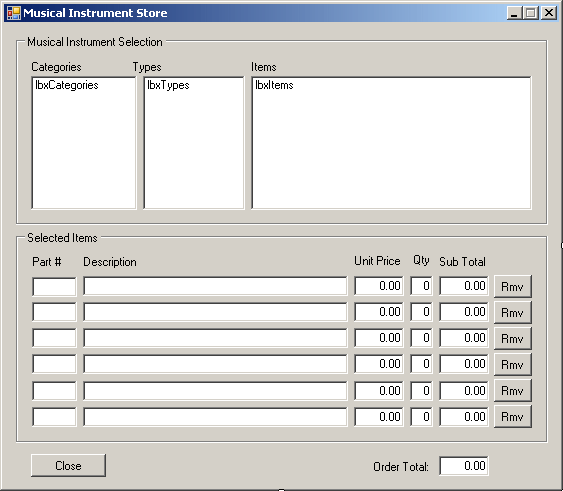 |
Control |
Text |
Name |
Other Properties |
GroupBox |
 |
Musical Instrument Selection |
|
|
Label |
 |
Categories |
|
|
Label |
 |
Types |
|
|
Label |
 |
Items |
|
|
ListBox |
 |
|
lbxCategories |
|
ListBox |
 |
|
lbxTypes |
|
ListBox |
 |
|
lbxItems |
|
GroupBox |
 |
Selected Items |
|
|
Label |
 |
|
Part # |
|
Label |
 |
|
Description |
|
Label |
 |
|
Unit Price |
|
Label |
 |
|
Qty |
|
Label |
 |
|
Sub Total |
|
TextBox |
 |
|
txtPartID1 |
|
TextBox |
 |
|
txtDescription1 |
|
TextBox |
 |
0.00 |
txtUnitPrice1 |
TextAlign: Right |
TextBox |
 |
0 |
txtQantity1 |
TextAlign: Right |
TextBox |
 |
0.00 |
txtSubTotal1 |
TextAlign: Right |
Button |
 |
Rmv |
btnRemove1 |
|
TextBox |
 |
|
txtPartID2 |
|
TextBox |
 |
|
txtDescription2 |
|
TextBox |
 |
0.00 |
txtUnitPrice2 |
TextAlign: Right |
TextBox |
 |
|
txtQuantity2 |
TextAlign: Right |
TextBox |
 |
0.00 |
txtSubTotal2 |
TextAlign: Right |
Button |
 |
Rmv |
btnRemove2 |
|
TextBox |
 |
|
txtPartID3 |
|
TextBox |
 |
|
txtDescription3 |
|
TextBox |
 |
0.00 |
txtUnitPrice3 |
TextAlign: Right |
TextBox |
 |
0 |
txtQuantity3 |
TextAlign: Right |
TextBox |
 |
0.00 |
txtSubTotal3 |
TextAlign: Right |
Button |
 |
Rmv |
btnRemove3 |
|
TextBox |
 |
|
txtPartID4 |
|
TextBox |
 |
|
txtDescription4 |
|
TextBox |
 |
0.00 |
txtUnitPrice4 |
TextAlign: Right |
TextBox |
 |
|
txtQuantity4 |
TextAlign: Right |
TextBox |
 |
0.00 |
txtSubTotal4 |
TextAlign: Right |
Button |
 |
Rmv |
btnRemove4 |
|
TextBox |
 |
|
txtPartID5 |
|
TextBox |
 |
|
txtDescription5 |
|
TextBox |
 |
0.00 |
txtUnitPrice5 |
TextAlign: Right |
TextBox |
 |
0 |
txtQuantity5 |
TextAlign: Right |
TextBox |
 |
0.00 |
txtSubTotal5 |
TextAlign: Right |
Button |
 |
Rmv |
btnRemove5 |
|
TextBox |
 |
|
txtPartID6 |
|
TextBox |
 |
|
txtDescription6 |
|
TextBox |
 |
0.00 |
txtUnitPrice6 |
TextAlign: Right |
TextBox |
 |
0 |
txtQuantity6 |
TextAlign: Right |
TextBox |
 |
0.00 |
txtSubTotal6 |
TextAlign: Right |
Button |
 |
Rmv |
btnRemove6 |
|
Button |
 |
Close |
btnClose |
|
Label |
 |
|
Order Total: |
|
TextBox |
 |
0.00 |
txtTotalOrder |
TextAlign: Right |
|
Like every control, when creating a list box, make sure
you give it a name. Once the list box is positioned on a container, as done
with other controls, you can move it by clicking and dragging the control.
You can also resize it using any of the techniques we learned to add,
position, move, and resize controls. If the list will cover many items,
design it so its height can display 8 items at a time. Otherwise, for a list
of 8 or less items, use only the necessary height that would accommodate all
of the items.
Adding Items to a List Box
|
|
The most important characteristic of a list box is the
list of items it contains. This list is represented by the Items property.
The Items list is created and managed by a ListBox-nested class named
ObjectCollection. ObjectCollection is a collection class that
implements the IList, the ICollection, and the IEnumerable
interfaces.
At design time, to create a list of items, access the
Properties window of the list box and click the ellipsis button of the Items
field. This would open the String Collection Editor:
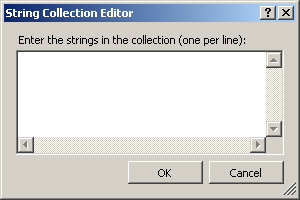
In the empty window, you can type an item, press Enter,
add another, and so on. After creating the list, you can click OK. To
programmatically add an item to the list, access the Items property,
call its Add() method, and pass the new item. You can do this
continually for each item. Here are examples:
private void InitializeComponent()
{
lbxFamily = new ListBox();
lbxFamily.Location = new Point(12, 12);
lbxFamily.Items.Add("Son");
lbxFamily.Items.Add("Daughter");
lbxFamily.Items.Add("Father");
lbxFamily.Items.Add("Mother");
Controls.Add(lbxFamily);
}
This would produce:

You can also first create an array of items and then add
that array to the collection. To support this, the ObjectCollection
class provides the AddRange() method. Here is an example:
private void InitializeComponent()
{
lbxFamily = new ListBox();
lbxFamily.Location = new Point(12, 12);
lbxFamily.Items.Add("Son");
lbxFamily.Items.Add("Daughter");
lbxFamily.Items.Add("Father");
lbxFamily.Items.Add("Mother");
string[] strMembers = { "Niece", "Nephew", "Uncle" };
lbxFamily.Items.AddRange(strMembers);
Controls.Add(lbxFamily);
}
This would produce:
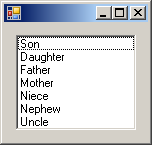
If you use either the Add() or the AddRange()
method to add an item or a group of items, the item or the group would be
added to the end of the list, if a list exists already. To insert a new item
somewhere inside of the list, call the Insert() method.
Application: Adding Items to a List Box
|
|
- On the form, click the Categories list box
- In the Properties window, click Items and click its ellipsis button
- Type Guitars and press Enter
- Type Bass and press Enter
- Complete the list to have the following items:
Guitars Bass Keyboards Drums &
Percussion Band & Orchestra Recording & Sound Folk
Instruments Books & Videos Accessories |
- Click OK
- To programmatically add items to the list boxes, on the form,
double-click an unoccupied area to access its Load event and implement
it as follows:
private void MusicStore_Load(object sender, EventArgs e)
{
lbxTypes.Items.Add("Electric Guitars");
lbxTypes.Items.Add("Acoustic Guitars");
lbxTypes.Items.Add("Acoustic-Electric Guitars");
lbxTypes.Items.Add("Amplifiers");
lbxTypes.Items.Add("Effects");
lbxTypes.Items.Add("Microphones");
lbxTypes.Items.Add("Accessories");
lbxTypes.Items.Add("Value Packages");
}
- Execute the application to test it
- After using the form, close it and return your programming
environment
- On the main menu, click Project -> Add Class...
- In the middle list, make sure Class is select.
Set the name to
PartDescription and click Add
- Complete the class as follows:
using System;
namespace MusicalInstrumentStore1
{
class PartDescription
{
private string number;
private string discr;
private decimal uprice;
public string PartNumber
{
get { return number; }
set { number = value; }
}
public string PartName
{
get { return discr; }
set { discr = value; }
}
public decimal UnitPrice
{
get { return uprice; }
set { uprice = value; }
}
public PartDescription()
{
this.number = "";
this.discr = "";
this.uprice = 0.00M;
}
public PartDescription(string nbr,
string name,
decimal price)
{
this.number = nbr;
this.discr = name;
this.uprice = price;
}
public override string ToString()
{
return this.PartNumber + " " +
this.PartName + " " +
this.UnitPrice.ToString();
}
}
}
- Access the MusicStore.cs file and create a few arrays as follows:
namespace MusicalInstrumentStore1
{
public partial class MusicStore : Form
{
string[] CatBass = {
"Electric Bass", "Acoustic-Electric Bass",
"Amplifiers", "Effects", "Accessories" };
string[] CatKeyboard = {
"Pianos", "Organs", "Synthesizers",
"Portable", "Workstations", "Arrangers",
"Stands", "Amps", "Pedals", "Accessories" };
string[] CatDrums = {
"Acoustic Drums", "Cymbals",
"Electronic Percussion", "World Percussion" };
string[] CatBand = {
"Trumpets", "Trombones", "Saxophones",
"Clarinets", "Flutes", "Baritones", "Tubas",
"Oboes", "Recorders", "Accessories" };
string[] CatAccessories = {
"Headphones", "Strings", "Slides", "Metronomes",
"Tuners", "Music Stands", "Cases", "Cables",
"Hearing Protection", "Electric Guitar Bags",
"Guitar Pedals", "Cleaning/Care" };
PartDescription[] ElectricGuitars =
{
new PartDescription("293027",
"Gibson Les Paul Vintage Solid Guitar",
850.75M),
new PartDescription("972355",
"Fender Standard Stratocaster Electric Guitar",
435.95M),
new PartDescription("390057",
"Gibson Les Paul Standard Left-Handed 50s Neck Electric Guitar",
2400.00M),
new PartDescription("297548",
"Schecter C-1 Hellraiser Electric Guitar",
649.95M),
new PartDescription("284704",
"Gretsch Guitars G5120 Electromatic Hollowbody Electric Guitar",
595.95M),
new PartDescription("293472",
"Rickenbacker 360 12-String Electric Guitar",
2195.95M),
new PartDescription("208476",
"Steinberger Synapse ST-2FPA TranScale Custom Electric Guitar",
1045.50M),
new PartDescription("253463",
"Gibson EDS 1275 Double-Neck Electric Guitar",
3050.25M),
new PartDescription("225747",
"Fender American Stratocaster Left-Handed Electric Guitar",
950.50M),
new PartDescription("274875",
"Epiphone Dot Studio Semi-Hollow Electric Guitar",
295.25M)
};
PartDescription[] AcousticGuitars =
{
new PartDescription("224885",
"Epiphone Hummingbird Acoustic Guitar",
245.55M),
new PartDescription("283407",
"Dean V Coustic Thin Body Acoustic-Electric Guitar",
205.50M),
new PartDescription("275111",
"Yamaha FG720S 12-String Acoustic Guitar",
325.55M),
new PartDescription("249036",
"Rogue RA-100D Dreadnought Acoustic Guitar",
82.95M),
new PartDescription("285507",
"Alvarez RD8 Regent Series Dreadnought Acoustic Guitar",
220.50M),
new PartDescription("283746",
"Epiphone EJ-200 Acoustic Guitar", 350.50M)
};
PartDescription[] ElectricBasses =
{
new PartDescription("248780",
"Epiphone Thunderbird IV Bass",
325.50M),
new PartDescription("203487",
"Squier® Vintage Modified '70s Jazz Bass",
305.95M),
new PartDescription("204633",
"Fender Standard Precision Bass",
450.75M),
new PartDescription("297548",
"Music Man StingRay 5-String Bass Guitar",
1485.95M)
};
PartDescription[] AcousElectBasses =
{
new PartDescription("248780",
"Ibanez AEB10E Acoustic-Electric Bass Guitar with Onboard Tuner",
335.50M),
new PartDescription("203487",
"Dean Playmate EABC 5-String Cutaway Acoustic-Electric Bass",
285.95M),
new PartDescription("204633",
"Fender BG-32 Acoustic/Electric Bass Guitar",
495.75M),
new PartDescription("634974",
"Gibson Thunderbird IV Bass", 1500.00M),
new PartDescription("674950",
"Rogue VB-100 Violin Bass", 255.95M),
new PartDescription("634742",
"Squier Standard P Bass 4-String Bass",
220.75M),
new PartDescription("637904",
"Peavey Millennium BXP 4-String Bass",
210.95M)
};
PartDescription[] Pianos =
{
new PartDescription("584603",
"Williams ETUDE Console Piano",
450.95M),
new PartDescription("504724",
"Rolan EP-760C Digital Piano w/Stand",
650.95M)
};
PartDescription[] Synthetizers =
{
new PartDescription("582970",
"Alesis ION 49-Key 1K DSP Synthesizer",
750.50M),
new PartDescription("524885",
"Korg MicroKORG Synthesizer/Vocoder",
350.75M),
new PartDescription("549085",
"Yamaha YDP223 Digital Piano",
1450.00M),
new PartDescription("529307",
"Access Virus kc 5-Octave Synth",
1915.55M)
};
PartDescription[] Books =
{
new PartDescription("883670",
"Alfred Guitar for the Absolute Beginner",
16.55M),
new PartDescription("837654",
"Hal Leonard Guitar Tab White Pages",
20.95M),
new PartDescription("843047",
"Carl Fischer Guitar Grimoire Progressions and Improvisation",
24.75M),
new PartDescription("845716",
"Bill Edwards Publishing Fretboard logic Spc Ed.",
17.95M),
new PartDescription("833427",
"Walrus Productions Guitar Chord Poster",
6.85M)
};
PartDescription[] Cables =
{
new PartDescription("188370",
"Musician's Friend Professional Cable",
4.55M),
new PartDescription("183614",
"Monster Cable S-100 Straight Cable",
20.95M),
new PartDescription("143047",
"Hosa TRS-TRS Stereo 1/4\" Cable",
4.65M),
new PartDescription("145716",
"Mogami Silver Series Cable",
12.95M)
};
. . . No Change
}
Selecting an Item in a List Box
|
|
To an item from a list box, the user must locate and
click the desired item. That item is said to have been selected. To
programmatically select an item, you can assign the index of the desired
item to the ListBox.SelectedIndex property. The indices of the items
of a list box are stored in a zero-based array. This means that the first
item has an index of 0, the second has an index of 1, and so on. Here is an
example that will select the fourth item of the list:
private void InitializeComponent()
{
lbxFamily = new ListBox();
lbxFamily.Location = new Point(12, 12);
lbxFamily.Items.Add("Son");
lbxFamily.Items.Add("Daughter");
lbxFamily.Items.Add("Father");
lbxFamily.Items.Add("Mother");
string[] strMembers = { "Niece", "Nephew", "Uncle" };
lbxFamily.Items.AddRange(strMembers);
Controls.Add(lbxFamily);
lbxFamily.SelectedIndex = 3;
}
This would produce:
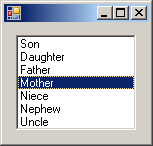
After an item has been selected, to find out the index
of the item that is currently selected, get the value of the
ListBox.SelectedIndex property.
To select an item, the user can click it in the list
box. When an item has been clicked, the list box fires a
SelectedIndexChanged event. Because selecting an item is the most
regularly performed operation on a list box, SelectedIndexChanged is
the default event of a list box. This event is of type EventArgs
which means that it does not provide any significant information other than
to let you know that an item has been selected. Nonetheless, this event
allows you to easily check if an item has been selected and what item has
been selected. To fire a SelectedIndexChanged event and to test
what item has been selected in the list, you can double-click the list box.
The ListBox.SelectedIndex property allows you
either to select an item or to find out what item is selected, using its
index, that is, the numeric position of the item in the list. If you know
the identity, such as the name, of the item you want to select, or if you
want to identify the selected item based on its name, you can use the
ListBox.SelectedItem property instead. This member identifies the item
instead of locating it.
By default, the user can select only one item in the
list. If you want the user to be able to select more than one item, change
the value of the SelectionMode property. This property is based on
the SelectionMode enumeration. After the user has selected more than
one item, to get the indexes of the items that are selected, you can access
the ListBox.SelectedIndices property which holds that list.
Application: Selecting an Item From a List Box
|
|
- Display the form and double-click the Categories list box
- Implement its event as follows:
private void lbxCategories_SelectedIndexChanged(object sender, EventArgs e)
{
if (lbxCategories.SelectedItem == (object)"Guitars")
{
lbxTypes.Items.Add("Electric Guitars");
lbxTypes.Items.Add("Acoustic Guitars");
lbxTypes.Items.Add("Acoustic-Electric Guitars");
lbxTypes.Items.Add("Amplifiers");
lbxTypes.Items.Add("Effects");
lbxTypes.Items.Add("Microphones");
lbxTypes.Items.Add("Accessories");
lbxTypes.Items.Add("Value Packages");
}
else if (lbxCategories.SelectedItem == (object)"Bass")
lbxTypes.Items.AddRange(CatBass);
else if (lbxCategories.SelectedItem == (object)"Keyboards")
lbxTypes.Items.AddRange(CatKeyboard);
else if (lbxCategories.SelectedItem == (object)"Drums & Percussion")
lbxTypes.Items.AddRange(CatDrums);
else if (lbxCategories.SelectedItem == (object)"Band & Orchestra")
lbxTypes.Items.AddRange(CatBand);
else if (lbxCategories.SelectedItem == (object)"Books & Videos")
{
lbxTypes.Items.Add("Books");
lbxTypes.Items.Add("DVDs");
}
else if (lbxCategories.SelectedItem == (object)"Accessories")
lbxTypes.Items.AddRange(CatAccessories);
}
- Return to the form
- On the form, double-click the Types list box and implement its event
as follows:
private void lbxTypes_SelectedIndexChanged(object sender, EventArgs e)
{
if( lbxTypes.SelectedItem == (object)"Electric Guitars" )
foreach(PartDescription part in ElectricGuitars)
lbxItems.Items.Add(part);
else if (lbxTypes.SelectedItem == (object)"Acoustic Guitars")
foreach(PartDescription part in AcousticGuitars )
lbxItems.Items.Add(part);
else if (lbxTypes.SelectedItem == (object)"Electric Bass")
foreach (PartDescription part in ElectricBasses)
lbxItems.Items.Add(part);
else if (lbxTypes.SelectedItem == (object)"Acoustic-Electric Bass")
foreach (PartDescription part in AcousElectBasses)
lbxItems.Items.Add(part);
else if (lbxTypes.SelectedItem == (object)"Pianos")
foreach(PartDescription part in Pianos)
lbxItems.Items.Add(part);
else if (lbxTypes.SelectedItem == (object)"Synthesizers")
foreach(PartDescription part in Synthetizers)
lbxItems.Items.Add(part);
else if (lbxTypes.SelectedItem == (object)"Books")
foreach(PartDescription part in Books)
lbxItems.Items.Add(part);
else if (lbxTypes.SelectedItem == (object)"Cables")
foreach(PartDescription part in Cables)
lbxItems.Items.Add(part);
}
- Execute the application to test it

- After using the form, close it and return to your programming
environment
- On the form, click the Items list box. In the Properties window,
click the Events button
and double-click the DoubleClick field
- Implement the event as follows:
internal void CalculateTotalOrder()
{
decimal subTotal1 = 0.00M, subTotal2 = 0.00M, subTotal3 = 0.00M,
subTotal4 = 0.00M, subTotal5 = 0.00M, subTotal6 = 0.00M;
decimal orderTotal;
// Retrieve the value of each sub total
try
{
subTotal1 = decimal.Parse(this.txtSubTotal1.Text);
}
catch (FormatException)
{
MessageBox.Show("Invalid Value");
}
try
{
subTotal2 = decimal.Parse(this.txtSubTotal2.Text);
}
catch (FormatException)
{
MessageBox.Show("Invalid Value");
}
try
{
subTotal3 = decimal.Parse(this.txtSubTotal3.Text);
}
catch (FormatException)
{
MessageBox.Show("Invalid Value");
}
try
{
subTotal4 = decimal.Parse(this.txtSubTotal4.Text);
}
catch (FormatException)
{
MessageBox.Show("Invalid Value");
}
try
{
subTotal5 = decimal.Parse(this.txtSubTotal5.Text);
}
catch (FormatException)
{
MessageBox.Show("Invalid Value");
}
try
{
subTotal6 = decimal.Parse(this.txtSubTotal6.Text);
}
catch (FormatException)
{
MessageBox.Show("Invalid Value");
}
// Calculate the total value of the sub totals
orderTotal = subTotal1 + subTotal2 + subTotal3 +
subTotal4 + subTotal5 + subTotal6;
// Display the total order in the appropriate text box
this.txtTotalOrder.Text = orderTotal.ToString();
}
private void lbxItems_DoubleClick(object sender, EventArgs e)
{
// We will use a PartDescription object to identify the selected item
PartDescription part = new PartDescription();
// When the user double-clicks an item, retrieve it as
// a PartDescription object
part = (PartDescription)(lbxItems.SelectedItem);
// If the first Part # box is empty, then use it
if (txtPartID1.Text.Equals(""))
{
// Display the item number in the Part # text box
txtPartID1.Text = part.PartNumber;
// Display the name of the selected item in
// the current Description text box
txtDescription1.Text = part.PartName;
// Display the unit price of this item in
// the corresponding Unit Price text box
this.txtUnitPrice1.Text = part.UnitPrice.ToString();
// Enable the Remove button of the current item
btnRemove1.Enabled = true;
// Since an item was selected, set its quantity to 1
this.txtQuantity1.Text = "1";
// Calculate the sub total of the current item item
this.txtSubTotal1.Text = (part.UnitPrice * 1).ToString();
// Give focus to the Qty text box of the current item
this.txtQuantity1.Focus();
}// If the previous Part # text box is not empty, then use the next one
else if (this.txtPartID2.Text.Equals(""))
{
this.txtPartID2.Text = part.PartNumber;
this.txtDescription2.Text = part.PartName;
this.txtUnitPrice2.Text = part.UnitPrice.ToString();
// Enable the Remove button of the current item
btnRemove2.Enabled = true;
this.txtQuantity2.Text = "1";
this.txtSubTotal2.Text = (part.UnitPrice * 1).ToString();
this.txtQuantity2.Focus();
}
else if (this.txtPartID3.Text.Equals(""))
{
this.txtPartID3.Text = part.PartNumber;
this.txtDescription3.Text = part.PartName;
this.txtUnitPrice3.Text = part.UnitPrice.ToString();
// Enable the Remove button of the current item
btnRemove3.Enabled = true;
this.txtQuantity3.Text = "1";
this.txtSubTotal3.Text = (part.UnitPrice * 1).ToString();
this.txtQuantity3.Focus();
}
else if (this.txtPartID4.Text.Equals(""))
{
this.txtPartID4.Text = part.PartNumber;
this.txtDescription4.Text = part.PartName;
this.txtUnitPrice4.Text = part.UnitPrice.ToString();
// Enable the Remove button of the current item
btnRemove4.Enabled = true;
this.txtQuantity4.Text = "1";
this.txtSubTotal4.Text = (part.UnitPrice * 1).ToString();
this.txtQuantity4.Focus();
}
else if (this.txtPartID5.Text.Equals(""))
{
this.txtPartID5.Text = part.PartNumber;
this.txtDescription5.Text = part.PartName;
this.txtUnitPrice5.Text = part.UnitPrice.ToString();
// Enable the Remove button of the current item
btnRemove5.Enabled = true;
this.txtQuantity5.Text = "1";
this.txtSubTotal5.Text = (part.UnitPrice * 1).ToString();
this.txtQuantity5.Focus();
}
else if (this.txtPartID6.Text.Equals(""))
{
this.txtPartID6.Text = part.PartNumber;
this.txtDescription6.Text = part.PartName;
this.txtUnitPrice6.Text = part.UnitPrice.ToString();
// Enable the Remove button of the current item
btnRemove6.Enabled = true;
this.txtQuantity6.Text = "1";
this.txtSubTotal6.Text = (part.UnitPrice * 1).ToString();
this.txtQuantity6.Focus();
} // If all Part # text boxes are filled, don't do anything
else
return;
// Calculate the current total order and update the order
CalculateTotalOrder();
}
- Display the form and click the first text box under Unit Price
- Press and hold Shift
- Click each of the text boxes under Unit Price and each of the text
boxes under Qty
- Release Shit
- In the Properties window and in the Events section, double-click the
Leave field
- Implement the event as follows:
private void txtUnitPrice1_Leave(object sender, EventArgs e)
{
int qty1 = 0, qty2 = 0, qty3 = 0,
qty4 = 0, qty5 = 0, qty6 = 0;
decimal unitPrice1 = 0.00M, unitPrice2 = 0.00M, unitPrice3 = 0.00M,
unitPrice4 = 0.00M, unitPrice5 = 0.00M, unitPrice6 = 0.00M;
decimal subTotal1, subTotal2, subTotal3,
subTotal4, subTotal5, subTotal6;
// Get the quantity of the current item
try
{
qty1 = int.Parse(txtQuantity1.Text);
}
catch (FormatException)
{
MessageBox.Show("Invalid Value");
}
// Get the unit price of the current item
try
{
unitPrice1 = decimal.Parse(txtUnitPrice1.Text);
}
catch (FormatException)
{
MessageBox.Show("Invalid Value");
}
try
{
qty2 = int.Parse(txtQuantity2.Text);
}
catch (FormatException)
{
MessageBox.Show("Invalid Value");
}
try
{
unitPrice2 = decimal.Parse(txtUnitPrice2.Text);
}
catch (FormatException)
{
MessageBox.Show("Invalid Value");
}
try
{
qty3 = int.Parse(txtQuantity3.Text);
}
catch (FormatException)
{
MessageBox.Show("Invalid Value");
}
try
{
unitPrice3 = decimal.Parse(txtUnitPrice3.Text);
}
catch (FormatException)
{
MessageBox.Show("Invalid Value");
}
try
{
qty4 = int.Parse(txtQuantity4.Text);
}
catch (FormatException)
{
MessageBox.Show("Invalid Value");
}
try
{
unitPrice4 = decimal.Parse(txtUnitPrice4.Text);
}
catch (FormatException)
{
MessageBox.Show("Invalid Value");
}
try
{
qty5 = int.Parse(txtQuantity5.Text);
}
catch (FormatException)
{
MessageBox.Show("Invalid Value");
}
try
{
unitPrice5 = decimal.Parse(txtUnitPrice5.Text);
}
catch (FormatException)
{
MessageBox.Show("Invalid Value");
}
try
{
qty6 = int.Parse(txtQuantity6.Text);
}
catch (FormatException)
{
MessageBox.Show("Invalid Value");
}
try
{
unitPrice6 = decimal.Parse(txtUnitPrice6.Text);
}
catch (FormatException)
{
MessageBox.Show("Invalid Value");
}
// Calculate the sub totals
subTotal1 = qty1 * unitPrice1;
subTotal2 = qty2 * unitPrice2;
subTotal3 = qty3 * unitPrice3;
subTotal4 = qty4 * unitPrice4;
subTotal5 = qty5 * unitPrice5;
subTotal6 = qty6 * unitPrice6;
// Display the sub totals in the corresponding text boxes
txtSubTotal1.Text = subTotal1.ToString();
txtSubTotal2.Text = subTotal2.ToString();
txtSubTotal3.Text = subTotal3.ToString();
txtSubTotal4.Text = subTotal4.ToString();
txtSubTotal5.Text = subTotal5.ToString();
txtSubTotal6.Text = subTotal6.ToString();
// Update the order
CalculateTotalOrder();
}
- Return to the form
- Double-click the first Rmv button and implement its Click event as
follows:
private void btnRemove1_Click(object sender, EventArgs e)
{
txtPartID1.Text = "";
txtDescription1.Text = "";
txtUnitPrice1.Text = "0.00";
txtQuantity1.Text = "0";
txtSubTotal1.Text = "0.00";
btnRemove1.Enabled = false;
}
- Return to the form
- Double-click the second Rmv button and implement its Click event as
follows:
private void btnRemove2_Click(object sender, EventArgs e)
{
txtPartID2.Text = "";
txtDescription2.Text = "";
txtUnitPrice2.Text = "0.00";
txtQuantity2.Text = "0";
txtSubTotal2.Text = "0.00";
btnRemove2.Enabled = false;
}
- Return to the form
- Double-click the third Rmv button and implement its Click event as
follows:
private void btnRemove3_Click(object sender, EventArgs e)
{
txtPartID3.Text = "";
txtDescription3.Text = "";
txtUnitPrice3.Text = "0.00";
txtQuantity3.Text = "0";
txtSubTotal3.Text = "0.00";
btnRemove3.Enabled = false;
}
- Return to the form
- Double-click the fourth Rmv button and implement its Click event as
follows:
private void btnRemove4_Click(object sender, EventArgs e)
{
txtPartID4.Text = "";
txtDescription4.Text = "";
txtUnitPrice4.Text = "0.00";
txtQuantity4.Text = "0";
txtSubTotal4.Text = "0.00";
btnRemove4.Enabled = false;
}
- Return to the form
- Double-click the fifth Rmv button and implement its Click event as
follows:
private void btnRemove5_Click(object sender, EventArgs e)
{
txtPartID5.Text = "";
txtDescription5.Text = "";
txtUnitPrice5.Text = "0.00";
txtQuantity5.Text = "0";
txtSubTotal5.Text = "0.00";
btnRemove5.Enabled = false;
}
- Return to the form
- Double-click the sixth Rmv button and implement its Click event as
follows:
private void btnRemove6_Click(object sender, EventArgs e)
{
txtPartID6.Text = "";
txtDescription6.Text = "";
txtUnitPrice6.Text = "0.00";
txtQuantity6.Text = "0";
txtSubTotal6.Text = "0.00";
btnRemove6.Enabled = false;
}
- Return to the form and double-click the Close button
- Implement its Click event as follows:
private void btnClose_Click(object sender, System.EventArgs e)
{
Close();
}
- Execute the application to test it
Removing Items From a List Box
|
|
If you have an undesired item in a list box, you can
remove it. To To support this operation, the ObjectCollection class
provides the Remove() method. When calling it, pass the name of the
item as argument. This means that you must know the item you are trying to
delete. If you call this method, the compiler would look for the item in the
list. If the item is found, it would be deleted.
Instead of removing an item by its name or
identification, you can use its position. To do that, you can call the
RemoveAt() method and pass the zero-based index of the undesired item.
If the index is valid, the item would be deleted from the list.
To remove all items from the list, you can call the
Clear() method.
Application: Removing Items From a List Box
|
|
- Change the SelectedIndex events of the Types and the Items list
boxes as follows:
private void lbxCategories_SelectedIndexChanged(object sender, EventArgs e)
{
lbxTypes.Items.Clear();
lbxItems.Items.Clear();
if (lbxCategories.SelectedItem == (object)"Guitars")
{
lbxTypes.Items.Add("Electric Guitars");
lbxTypes.Items.Add("Acoustic Guitars");
lbxTypes.Items.Add("Acoustic-Electric Guitars");
lbxTypes.Items.Add("Amplifiers");
lbxTypes.Items.Add("Effects");
lbxTypes.Items.Add("Microphones");
lbxTypes.Items.Add("Accessories");
lbxTypes.Items.Add("Value Packages");
}
else if (lbxCategories.SelectedItem == (object)"Bass")
lbxTypes.Items.AddRange(CatBass);
else if (lbxCategories.SelectedItem == (object)"Keyboards")
lbxTypes.Items.AddRange(CatKeyboard);
else if (lbxCategories.SelectedItem == (object)"Drums & Percussion")
lbxTypes.Items.AddRange(CatDrums);
else if (lbxCategories.SelectedItem == (object)"Band & Orchestra")
lbxTypes.Items.AddRange(CatBand);
else if (lbxCategories.SelectedItem == (object)"Books & Videos")
{
lbxTypes.Items.Add("Books");
lbxTypes.Items.Add("DVDs");
}
else if (lbxCategories.SelectedItem == (object)"Accessories")
lbxTypes.Items.AddRange(CatAccessories);
}
private void lbxTypes_SelectedIndexChanged(object sender, EventArgs e)
{
lbxItems.Items.Clear();
if( lbxTypes.SelectedItem == (object)"Electric Guitars" )
foreach(PartDescription part in ElectricGuitars)
lbxItems.Items.Add(part);
else if (lbxTypes.SelectedItem == (object)"Acoustic Guitars")
foreach(PartDescription part in AcousticGuitars )
lbxItems.Items.Add(part);
else if (lbxTypes.SelectedItem == (object)"Electric Bass")
foreach (PartDescription part in ElectricBasses)
lbxItems.Items.Add(part);
else if (lbxTypes.SelectedItem == (object)"Acoustic-Electric Bass")
foreach (PartDescription part in AcousElectBasses)
lbxItems.Items.Add(part);
else if (lbxTypes.SelectedItem == (object)"Pianos")
foreach(PartDescription part in Pianos)
lbxItems.Items.Add(part);
else if (lbxTypes.SelectedItem == (object)"Synthesizers")
foreach(PartDescription part in Synthetizers)
lbxItems.Items.Add(part);
else if (lbxTypes.SelectedItem == (object)"Books")
foreach(PartDescription part in Books)
lbxItems.Items.Add(part);
else if (lbxTypes.SelectedItem == (object)"Cables")
foreach(PartDescription part in Cables)
lbxItems.Items.Add(part);
}
- Execute the application to test it. Here is an example:
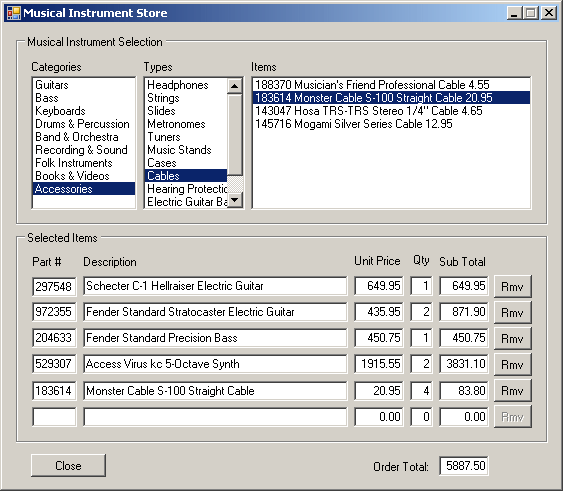
- Close the form and return to your programming environment
After creating the list, by default, each item assumes
the position it received when it was added. If you want, you can rearrange
them in ascending order. To do this, set the ListBox.Sorted Boolean
property to True. If you create an unsorted list, then at one time
get it sorted (for example, you can give the user the ability to sort the
list, by clicking a button), the list would be sorted. If an item is added
to the sorted list, the compiler would automatically insert it to the right
position following the alphabetical, ascending or chronological order. If at
another time you allow the user to "unsort"� the
list, the list would keep its current order. If another item is added when
the list is not sorted, the item would be positioned at the end of the list.
If you want the list to have its original state, you would have to reset it
through code.
Application: Sorting a List Box
|
|
- On the form, click the Items list box
- In the Properties window, double-click Sorted to set its value to
True
Characteristics of a List Box
|
|
If you provide a longer list than the list box' height
can display, it would have a vertical scroll bar. Here is an example:
using System;
using System.Drawing;
using System.Windows.Forms;
public class Exercise : System.Windows.Forms.Form
{
ListBox lbxFamily;
public Exercise()
{
InitializeComponent();
}
private void InitializeComponent()
{
lbxFamily = new ListBox();
lbxFamily.Location = new Point(12, 12);
lbxFamily.Items.Add("Son");
lbxFamily.Items.Add("Daughter");
lbxFamily.Items.Add("Father");
lbxFamily.Items.Add("Mother");
string[] strMembers =
{
"Niece", "Nephew", "Uncle", "Aunt",
"Grand Father", "Grand Mother"
};
lbxFamily.Items.AddRange(strMembers);
Controls.Add(lbxFamily);
}
}
public class Program
{
static int Main()
{
System.Windows.Forms.Application.Run(new Exercise());
return 0;
}
}
This would produce:
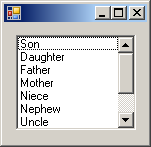
At design time, if just one or a few items are hidden by
the scroll bar, you can heighten it if the form provides more space.
Consider the following example:
public class Exercise : System.Windows.Forms.Form
{
ListBox lbxBook;
Label lblTitle;
public Exercise()
{
InitializeComponent();
}
private void InitializeComponent()
{
lblTitle = new Label();
lblTitle.Text = "Book Titles";
lblTitle.Location = new Point(12, 12);
lbxBook = new ListBox();
lbxBook.Location = new Point(12, 36);
lbxBook.Items.Add("College Algebra");;
lbxBook.Items.Add("Finite Mathematics");
lbxBook.Items.Add("Mathematical Structures");
lbxBook.Items.Add("MCAD 70-316 Training Guide");
lbxBook.Items.Add("C++ Builder 6 Developer's Guide");
Controls.Add(lblTitle);
Controls.Add(lbxBook);
}
}
This would produce:
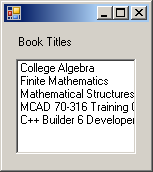
If at least one of the items of the list box is wider
than the width of the control, the right side(s) of that (those) may
disappear. To allow the user to see the hidden part of the item(s), you
should display a horizontal scroll bar. To support this, the ListBox
class is equipped with a Boolean property named HorizontalScrollbar.
To make a list box display a horizontal scroll bar, at design time, access
the Properties window for the list box and set its HorizontalScrollbar
property to True. You can also do this programmatically. Here is an example:
private void InitializeComponent()
{
lblTitle = new Label();
lblTitle.Text = "Book Titles";
lblTitle.Location = new Point(12, 12);
lbxBook = new ListBox();
lbxBook.Location = new Point(12, 36);
lbxBook.Items.Add("College Algebra");;
lbxBook.Items.Add("Finite Mathematics");
lbxBook.Items.Add("Mathematical Structures");
lbxBook.Items.Add("MCAD 70-316 Training Guide");
lbxBook.Items.Add("C++ Builder 6 Developer's Guide");
bxBook.HorizontalScrollbar = true;
Controls.Add(lblTitle);
Controls.Add(lbxBook);
}
This property allows the operating system to find the
widest item in the list and provide a horizontal scroll bar that is long
enough to display each item when the user scrolls to the right. The above
code would produce:
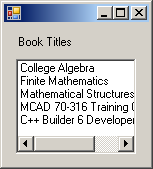
If the list of items requires it, the list box would
display both the vertical and the horizontal scroll bars. Here is an
example:
public class Exercise : System.Windows.Forms.Form
{
ListBox lbxBook;
Label lblTitle;
public Exercise()
{
InitializeComponent();
}
private void InitializeComponent()
{
lblTitle = new Label();
lblTitle.Text = "Book Titles";
lblTitle.Location = new Point(12, 12);
lbxBook = new ListBox();
lbxBook.Location = new Point(12, 36);
lbxBook.Items.Add("College Algebra");;
lbxBook.Items.Add("Finite Mathematics");
lbxBook.Items.Add("Mathematical Structures");
lbxBook.Items.Add("MCAD 70-316 Training Guide");
lbxBook.Items.Add("C++ Builder 6 Developer's Guide");
lbxBook.Items.Add("La Bible de Jérusalem");
lbxBook.Items.Add("Patterns for a Purpose");
lbxBook.HorizontalScrollbar = true;
Controls.Add(lblTitle);
Controls.Add(lbxBook);
}
}
This would produce:
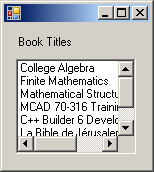
If you prefer to decide how much width should be
allowed, then set the desired value in the HorizontalExtent property.
Application:
Implementing List Boxes
|
|
- Display the form and click the most right list box. Using the
Properties window, set its HorizontalScrollbar property to
True
- Execute the application and process an order
- After using it, close the form and return to your programming
environment
When you create a list of items, they appear in one
column. If the number of items exceeds the height, a scrollbar would appear
on the control. An alternative you can use is to span the list to more than
one column. To support this, the ListBox class is equipped with the
MultiColumn Boolean property. At design time, you can set this
characteristic in the Properties window. By default, the MultiColumn
value is set to False, which means the items appear in one column. If you
set this property to True, then the compiler would decide if or when the
control needs the columns, based on the number of items in the list. You can
then specify the width of each column using the ColumnWidth property.
Intermediate Operations on a List Box
|
|
There are various ways you can involve a list box in a
drag n' drop operation. For example, to add an item to a list box, you can
allow a user to drag from another control or object and drop it in the list
box. You can also allow a user to drag and drop list box elements from one
list box to another.
To support drag n' drop operations, the list box is
equipped with the AllowDrop property. Therefore, if you
want to implement this operation, first set the AllowDrop
property to true. Here is an example:
using System;
using System.Drawing;
using System.Windows.Forms;
public class Exercise : System.Windows.Forms.Form
{
ListBox lbxSource;
ListBox lbxTarget;
public Exercise()
{
InitializeComponent();
}
void InitializeComponent()
{
lbxSource = new ListBox();
lbxSource.Items.Add("James");
lbxSource.Items.Add("Gertrude");
lbxSource.Items.Add("Paul");
lbxSource.Items.Add("Hélène");
lbxSource.Location = new Point(12, 12);
lbxSource.Size = new System.Drawing.Size(100, 100);
lbxTarget = new ListBox();
lbxTarget.AllowDrop = true;
lbxTarget.Location = new Point(120, 12);
lbxTarget.Size = new System.Drawing.Size(100, 100);
Text = "Employees Names";
Controls.Add(lbxSource);
Controls.Add(lbxTarget);
}
}
public class Program
{
[STAThread]
public static int Main()
{
System.Windows.Forms.Application.Run(new Exercise());
return 0;
}
}
As mentioned in Lesson 6, to create a drag n' drop
scenario, you should implement the DragEnter event of the target control to
find out what the user is dragging. To drop the item in the target, you
should implement its DragDrop event. Here is an example of two list boxes
that allows a user to drag an item from the source list box and drop it on
the target list box:
using System;
using System.Drawing;
using System.Windows.Forms;
public class Exercise : System.Windows.Forms.Form
{
ListBox lbxSource;
ListBox lbxTarget;
public Exercise()
{
InitializeComponent();
}
void InitializeComponent()
{
lbxSource = new ListBox();
lbxSource.Location = new Point(12, 12);
lbxSource.Size = new System.Drawing.Size(100, 100);
lbxSource.MouseDown += new MouseEventHandler(lbxSourceMouseDown);
lbxTarget = new ListBox();
lbxTarget.AllowDrop = true;
lbxTarget.Location = new Point(120, 12);
lbxTarget.Size = new System.Drawing.Size(100, 100);
lbxTarget.DragEnter += new DragEventHandler(lbxTargetDragEnter);
lbxTarget.DragDrop += new DragEventHandler(lbxTargetDragDrop);
Text = "Employees Names";
Controls.Add(lbxSource);
Controls.Add(lbxTarget);
lbxSource.Items.Add("James");
lbxSource.Items.Add("Gertrude");
lbxSource.Items.Add("Paul");
lbxSource.Items.Add("Hélène");
}
private void lbxSourceMouseDown(object sender, MouseEventArgs e)
{
// Find out if the user had clicked (with the left mouse button.
// If so, prepare to copy the item the user had clicked
if (e.Button == System.Windows.Forms.MouseButtons.Left)
lbxSource.DoDragDrop(lbxSource.SelectedItem.ToString(), DragDropEffects.Copy);
}
private void lbxTargetDragEnter(object sender, DragEventArgs e)
{
// When the user gets to the target list box, specify that you want to copy
e.Effect = DragDropEffects.Copy;
}
private void lbxTargetDragDrop(object sender, DragEventArgs e)
{
// Before dropping, get the string that the user is dragging
string strSelected = e.Data.GetData(DataFormats.Text).ToString();
// Once you know what string the user is dragging,
// add it to the target list box
if (!strSelected.Equals(""))
((ListBox)sender).Items.Add(strSelected);
}
}
public class Program
{
[STAThread]
public static int Main()
{
System.Windows.Forms.Application.Run(new Exercise());
return 0;
}
}
A Custom Owner-Draw List Box
|
|
A list box is painted based on three types or style.
This characteristic is controlled by the DrawMode property. When its
value is set to Normal, the operating system would regularly draw
each item of the list. If you want each item of the list to display a
graphic or a color, you must set the style to an owner drawn type. The
OwnerDrawFixed value allows you to set a desired but same height for
each item of the list. This height is controlled through the ItemHeight
property. You can set a different height for each item if you set the list
style to OwnerDrawVariable.
|
|