 |
Example Application: Simple Interest |
|
|
The date/time picker control provides a convenient way to select
a date to use in an application. This reduces the likelihood of mistakes. To
illustrate it, we will create an application that calculates the values of
an interest paid on a loan.
|
Application:
Introducing the Application
|
|
- Start a new Window Application named SimpleInterest2
- Design the form as follows:
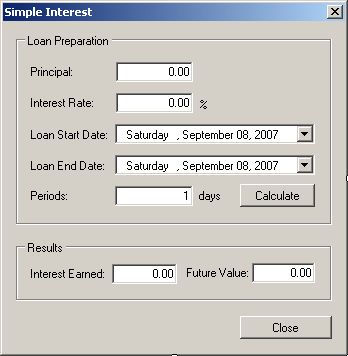 |
Control |
Text |
Name |
TextAlign |
GroupBox |
 |
Loan Preparation |
|
|
Label |
 |
Principal |
|
|
TextBox |
 |
0.00 |
txtPrincipal |
Right |
Label |
 |
Interest Rate: |
|
|
TextBox |
 |
0.00 |
txtInterestRate |
Right |
Label |
 |
% |
|
|
Label |
 |
Loan Start Date: |
|
|
DateTimePicker |
 |
|
dtpStartDate |
|
Label |
 |
Loan End Date: |
|
|
DateTimePicker |
 |
|
dtpLoandEndDate |
|
Label |
 |
Periods: |
|
|
TextBox |
 |
1 |
txtPeriods |
Right |
Label |
 |
days |
|
|
Button |
 |
Calculate |
btnCalculate |
|
GroupBox |
 |
Results |
|
|
Label |
 |
Interest Earned: |
|
|
TextBox |
 |
0.00 |
txtInterestEarned |
Right |
Label |
 |
Future Value: |
|
|
TextBox |
 |
0.00 |
txtFutureValue |
Right |
Button |
 |
Close |
btnClose |
|
|
- On the form, click the dtdEndDate date picker control
- In the Properties window, click the Events button and double-click
CloseUp to generate its event
- Implement the event as follows:
private void dtpEndDate_CloseUp(object sender, EventArgs e)
{
double Principal = 0.00D,
InterestRate = 0.00D,
Periods = 0.00D;
double InterestEarned, FutureValue;
DateTime StartDate, EndDate;
TimeSpan spnPeriods;
// Get the value of the principal
try
{
Principal = double.Parse(txtPrincipal.Text);
}
catch (FormatException)
{
MessageBox.Show("Invalid Principal Value");
}
// Get the interest rate
try
{
InterestRate = double.Parse(txtInterestRate.Text) / 100;
}
catch (FormatException)
{
MessageBox.Show("Invalid Interest Rate");
}
// Get the start and end dates
StartDate = dtpStartDate.Value;
EndDate = dtpEndDate.Value;
// Make sure the end date doesn't occur before the start date
if (EndDate < StartDate)
{
MessageBox.Show("Invalid Date Sequence: " +
"the end date must occur after the start date");
dtpEndDate.Value = DateTime.Today;
return;
}
// Get the difference in days
// The will be the periods
spnPeriods = EndDate.Subtract(StartDate);
int days = spnPeriods.Days;
txtPeriods.Text = days.ToString();
double p = 0.00D;
// Because we will allow the user to directly specify
// the number of days, let's get the period from its text box
try
{
p = double.Parse(txtPeriods.Text);
}
catch (FormatException)
{
MessageBox.Show("Invalid number of days");
}
// The actual period is gotten as follows
Periods = p / 365;
// Now we can perform the calculations
InterestEarned = Principal * InterestRate * Periods;
FutureValue = Principal + InterestEarned;
// Display the values
txtInterestEarned.Text = InterestEarned.ToString("C");
txtFutureValue.Text = FutureValue.ToString("C");
}
- Return to the form and click the Periods text box
- From the Events section of the Properties window, double-click Leave
- Implement the event as follows:
private void txtPeriods_Leave(object sender, EventArgs e)
{
// If the user directly enters the number of days
// in the Periods text box and press Tab,
// we can perform the caltulation
dtpEndDate_CloseUp(sender, e);
}
- Return to the form and double-click the Calculate button
- Implement the event as follows:
private void btnCalculate_Click(object sender, EventArgs e)
{
// If the user directly enters the number of days
// in the Periods text box and clicks the Calculate
// button, we can perform the caltulation
dtpEndDate_CloseUp(sender, e);
}
- Return to the form and double-click the Close button
- Implement the event as follows:
private void btnClose_Click(object sender, EventArgs e)
{
Close();
}
- Return to the form
- In the Properties window, click the Properties button

- On the form, click the top date picker control
- In the Properties window, change the following values:
CalendarForeColor: Blue CalendarMonthBackground: SkyBlue CalendarTitleBackColor: Navy CalendarTitleForeColor: Gold CalendarTrailingForeColor: DodgeBlue
- On the form, click the top date picker control
- In the Properties window, change the following values:
CalendarForeColor: Maroon CalendarMonthBackground: Orange CalendarTitleBackColor: Sienna CalendarTitleForeColor: Khaki CalendarTrailingForeColor: White
- Execute the application and test the application
- Close the form and return to your programming environment
|
|