 |
XML-Based Application: Solas Property Rental |
|
|
Here is an example of an application where values are
saved in XML files. We also review some techniques of creating elements and
updating them.
|
Practical
Learning: Introducing operations on XML Elements
|
|
- Start Microsoft Visual Studio
- To create a new application, on the main menu, click File -> New
Project...
- In the middle list, click Windows Forms Application
- Change the Name to SolasPropertyRental1
- Click OK
- To add a new form to the application, in the Solution Explorer,
right-click SolasPropertyRental1 -> Add -> Windows Form...
- Set the Name to Tenants and click Add
- From the Toolbox, add a ListView to the form
- While the new list view is still selected, in the Properties window,
click the ellipsis button of the Columns field and create the columns as
follows:
(Name) |
Text |
TextAlign |
Width |
colAccountNumber |
Account # |
|
65 |
colFullName |
Full Name |
|
120 |
colMaritalStatus |
Marital Status |
|
85 |
colPhoneNumber |
Phone # |
Center |
85 |
- Design the form as follows:
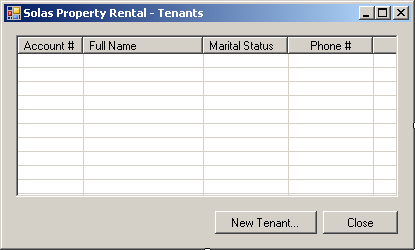 |
Control |
Text |
Name |
Other Properties |
ListView |
|
lvwTenants |
Anchor: Top, Bottom, Left, Right
FullRowSelect: True GridLines: True View:
Details |
Button |
New Tenant... |
btnNewTenant |
Anchor: Bottom, Right |
Button |
Close |
btnClose |
Anchor: Bottom, Right |
|
- Double-click an unoccupied area of the Tenants form
- Implement the Load event as follows:
using System;
using System.Collections.Generic;
using System.ComponentModel;
using System.Data;
using System.Drawing;
using System.Linq;
using System.Text;
using System.Windows.Forms;
using System.IO;
using System.Xml;
namespace SolasPropertyRental1
{
public partial class Tenants : Form
{
public Tenants()
{
InitializeComponent();
}
void ShowTenants()
{
string Filename = @"C:\Solas Property Rental\tenants.xml";
XmlDocument docTenants = new XmlDocument();
if (File.Exists(Filename))
{
lvwTenants.Items.Clear();
docTenants.Load(Filename);
XmlElement elmTenant = docTenants.DocumentElement;
XmlNodeList lstTenants = elmTenant.ChildNodes;
foreach (XmlNode node in lstTenants)
{
ListViewItem lviTenant = new ListViewItem(node.FirstChild.InnerText); // Account Number
lviTenant.SubItems.Add(node.FirstChild.NextSibling.InnerText); // Full Name
lviTenant.SubItems.Add(node.FirstChild.NextSibling.NextSibling.InnerText); // Phone Number
lviTenant.SubItems.Add(node.FirstChild.NextSibling.NextSibling.NextSibling.InnerText); // Marital Status
lvwTenants.Items.Add(lviTenant);
}
}
}
private void Tenants_Load(object sender, EventArgs e)
{
ShowTenants();
}
}
}
- To add another form to the application, in the Solution Explorer,
right- click SolasPropertyRental1 -> Add -> Windows Form...
- Set the Name to TenantEditor and click Add
- Design the form as follows:
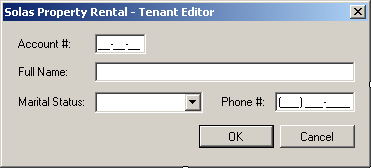 |
Control |
Text |
Name |
Properties |
Label |
&Account #: |
|
|
MaskedTextBox |
|
txtAccountNumber |
Mask: 00-00-00 Modifiers: public |
Label |
&Full Name: |
|
|
TextBox |
|
txtFullName |
Modifiers: public |
Label |
Marital Status: |
|
|
ComboBox |
|
txtMaritalStatus |
Modifiers: public Items:
Single Widow Married Divorced Separated |
Label |
&Phone #: |
|
|
MaskedTextBox |
|
txtPhoneNumber |
Mask: (999) 000-0000 Modifiers:
public |
Button |
OK |
btnOK |
DialogResult: OK |
Button |
Cancel |
btnCancel |
DialogResult: Cancel |
Form |
|
|
AcceptButton: btnOK CancelButton:
btnCancel FormBorderStyle: FixedDialog
MaximizeBox: False MinimizeBox: False
ShowInTaskbar: False |
|
- To add a new form to the application, in the Solution Explorer,
right- click SolasPropertyRental1 -> Add -> Windows Form...
- Set the Name to RentalProperties and press Enter
- From the Toolbox, add a ListView to the form
- While the new list view is still selected, in the Properties window,
click the ellipsis button of the Columns field and create the columns as
follows:
(Name) |
Text |
TextAlign |
Width |
colPropertyCode |
Prop Code |
|
65 |
colPropertyType |
Property Type |
|
85 |
colBedrooms |
Bedrooms |
Right |
65 |
colBathrooms |
Bathrooms |
Right |
65 |
colMonthlyRent |
Monthly Rent |
Right |
75 |
colStatus |
Status |
|
65 |
- Design the form as follows:
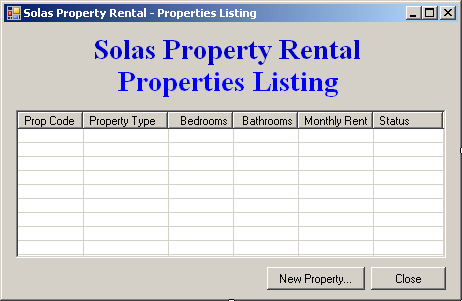 |
Control |
Text |
Name |
Other Properties |
ListView |
|
lvwProperties |
View: Details GridLines: True
FullRowSelect: True Anchor: Top, Bottom, Left,
Right |
Button |
New Property... |
btnNewProperty |
Anchor: Bottom, Right |
Button |
Close |
btnClose |
Anchor: Bottom, Right |
|
- Double-click an unoccupied area of the Rental Properties form and
implement the Load event as follows:
using System;
using System.Collections.Generic;
using System.ComponentModel;
using System.Data;
using System.Drawing;
using System.Linq;
using System.Text;
using System.Windows.Forms;
using System.IO;
using System.Xml;
namespace SolasPropertyRental1
{
public partial class RentalProperties : Form
{
public RentalProperties()
{
InitializeComponent();
}
void ShowProperties()
{
XmlDocument docProperties = new XmlDocument();
string Filename = @"C:\Solas Property Rental\properties.xml";
if (File.Exists(Filename))
{
lvwProperties.Items.Clear();
docProperties.Load(Filename);
XmlElement elmProperty = docProperties.DocumentElement;
XmlNodeList lstProperties = elmProperty.ChildNodes;
foreach (XmlNode node in lstProperties)
{
ListViewItem lviProperty = new ListViewItem(node.FirstChild.InnerText); // Property Code
lviProperty.SubItems.Add(node.FirstChild.NextSibling.InnerText); // Property Type
lviProperty.SubItems.Add(node.FirstChild.NextSibling.NextSibling.InnerText); // Bedrooms
lviProperty.SubItems.Add(node.FirstChild.NextSibling.NextSibling.NextSibling.InnerText); // Bathrooms
lviProperty.SubItems.Add(node.FirstChild.NextSibling.NextSibling.NextSibling.NextSibling.InnerText); // Monthly Rent
lviProperty.SubItems.Add(node.FirstChild.NextSibling.NextSibling.NextSibling.NextSibling.NextSibling.InnerText); // Status
lvwProperties.Items.Add(lviProperty);
}
}
}
private void RentalProperties_Load(object sender, EventArgs e)
{
ShowProperties();
}
}
}
- To add another form to the application, in the Solution Explorer,
right- click SolasPropertyRental1 -> Add -> Windows Form...
- Set the Name to PropertyEditor and click Add
- Design the form as follows:
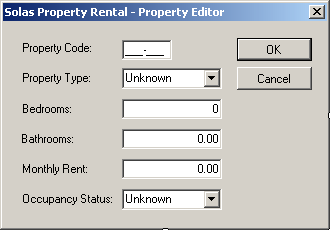 |
Control |
Text |
Name |
Properties |
Label |
Property Code: |
|
|
MaskedTextBox |
|
txtPropertyCode |
Mask: 000-000 Modifiers: Public |
Button |
OK |
btnOK |
DialogResult: OK |
Label |
Property Type: |
|
|
ComboBox |
|
cbxPropertyTypes |
Modifiers: Public Items: Unknown
Apartment Townhouse Single Family |
Button |
Cancel |
btnCancel |
DialogResult: Cancel |
Label |
Bedrooms: |
|
|
TextBox |
0 |
txtBedrooms |
TextAlign: Right Modifiers:
Public |
Label |
Bathrooms: |
|
|
TextBox |
0.00 |
txtBathrooms |
TextAlign: Right Modifiers:
Public |
Label |
Monthly Rent: |
|
|
TextBox |
0.00 |
txtMonthlyRent |
TextAlign: Right Modifiers:
Public |
Label |
Occupancy Status: |
|
|
ComboBox |
Unknown |
cbxStatus |
Modifiers: Public Items:
Unknown Available Occupied Needs Repair |
Form |
|
|
AcceptButton: btnOK CancelButton:
btnCancel FormBorderStyle: FixedDialog
MaximizeBox: False MinimizeBox: False
ShowInTaskbar: False |
|
- To add a new form to the application, in the Solution Explorer,
right-click SolasPropertyRental1 -> Add -> Windows Form...
- Set the Name to RentalAllocation and click Add
- From the Toolbox, add a ListView to the form
- Design the form as follows:
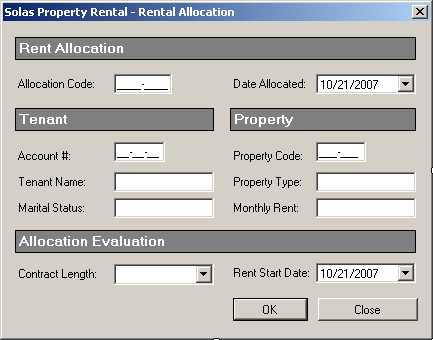 |
Control |
Text |
Name |
Other Properties |
Label |
Rent Allocation |
|
AutoSize: False BackColor: Gray
BorderStyle: FixedSingle ForeColor: White
TextAlign: MiddleLeft |
Label |
Allocation Code: |
|
|
MaskedTextBox |
|
txtAllocationCode |
Mask: 0000-9999 Modifiers: Public |
Label |
Date Allocated: |
|
|
DateTimePicker |
|
dtpDateAllocated |
Format: Short Modifiers: Public |
Label |
Tenant |
|
AutoSize: False BackColor: Gray
BorderStyle: FixedSingle ForeColor: White
TextAlign: MiddleLeft |
Label |
Property |
|
AutoSize: False BackColor: Gray
BorderStyle: FixedSingle ForeColor: White
TextAlign: MiddleLeft |
Label |
Account #: |
|
|
MaskedTextBox |
|
txtTenantAcntNbr |
Mask: 00-00-00 Modifiers: Public |
Label |
Property #: |
|
|
MaskedTextBox |
|
txtPropertyCode |
Mask: 000-000 Modifiers: Public |
Label |
Tenant Name |
|
|
TextBox |
|
txtTenantName |
Modifiers: Public |
Label |
Property Type: |
|
|
TextBox |
|
txtPropertyType |
Modifiers: Public |
Label |
Marital Status: |
|
|
TextBox |
|
txtMaritalStatus |
Modifiers: Public |
Label |
Monthly Rent: |
|
|
TextBox |
|
txtMonthlyRent |
|
Label |
Allocation Evaluation |
|
AutoSize: False BackColor: Gray
BorderStyle: FixedSingle ForeColor: White
TextAlign: MiddleLeft |
Label |
Contract Length: |
|
|
ComboBox |
|
cbxContractLength |
Items: Monthly 3 Months 6
Months 12 Months Modifiers: Public |
Label |
Rent Start Date: |
|
|
DateTimePicker |
|
dtpRentStartDate |
Modifiers: Public |
Button |
OK |
btnOK |
DialogResult: OK |
Button |
Close |
btnClose |
DialogResult: Cancel |
Form |
|
|
AcceptButton: btnOK CancelButton:
btnClose FormBorderStyle: FixedDialog MaximizeBox:
False MinimizeBox: False ShowInTaskbar: False |
|
- On the form, click the Account # text box and, in the Properties
window, double-click Leave
- Implement the event as follows:
using System;
using System.Collections.Generic;
using System.ComponentModel;
using System.Data;
using System.Drawing;
using System.Linq;
using System.Text;
using System.Windows.Forms;
using System.IO;
using System.Xml;
namespace SolasPropertyRental1
{
public partial class RentalAllocation : Form
{
public RentalAllocation()
{
InitializeComponent();
}
private void txtTenantAcntNbr_Leave(object sender, EventArgs e)
{
string Filename = @"C:\Solas Property Rental\tenants.xml";
XmlDocument docTenants = new XmlDocument();
if (File.Exists(Filename))
{
docTenants.Load(Filename);
XmlElement elmTenant = docTenants.DocumentElement;
XmlNodeList lstTenants = elmTenant.ChildNodes;
bool TenantFound = false;
for (int i = 0; i < lstTenants.Count; i++)
{
XmlNode node = lstTenants[i];
if (node.FirstChild.InnerText == txtTenantAcntNber.Text)
{
TenantFound = true;
txtTenantName.Text = node.FirstChild.NextSibling.InnerText;
txtMaritalStatus.Text = node.FirstChild.NextSibling.NextSibling.InnerText;
}
}
if (TenantFound == false)
{
MessageBox.Show("There is no tenant with that account number");
txtTenantAcntNber.Text = "";
}
}
else
MessageBox.Show("There is no list of tenants to check.");
}
}
}
- Return to the Rent Allocation form and click the Property Code text
box
- In the Events section of the Properties window, double-click Leave
- Implement the event as follows:
private void txtPropertyCode_Leave(object sender, EventArgs e)
{
string Filename = @"C:\Solas Property Rental\properties.xml";
XmlDocument docProperties = new XmlDocument();
if (File.Exists(Filename))
{
docProperties.Load(Filename);
XmlElement elmProperty = docProperties.DocumentElement;
XmlNodeList lstProperties = elmProperty.ChildNodes;
bool PropertyFound = false;
for (int i = 0; i < lstProperties.Count; i++)
{
XmlNode node = lstProperties[i];
if (node.FirstChild.InnerText == txtPropertyCode.Text)
{
PropertyFound = true;
txtPropertyType.Text = node.FirstChild.NextSibling.InnerText;
txtMonthlyRent.Text = node.FirstChild.NextSibling.NextSibling.NextSibling.NextSibling.InnerText;
}
}
if (PropertyFound == false)
{
MessageBox.Show("There is no Property with that code");
txtPropertyType.Text = "";
}
}
else
MessageBox.Show("There is no list of properties to check.");
}
- To add a new form to the application, in the Solution Explorer,
right- click SolasPropertyRental1 -> Add -> Windows Form...
- Set the Name to RentalAllocations and press Enter
- From the Toolbox, add a ListView to the form
- While the new list view is still selected, in the Properties window,
click the ellipsis button of the Columns field and create the columns as
follows:
(Name) |
Text |
TextAlign |
Width |
colAllocationCode |
Alloc Code |
|
65 |
colDateAllocated |
Date Allocated |
Center |
85 |
colTenantAccount |
Tenant # |
Center |
65 |
colTenantName |
Tenant Name |
|
100 |
colPropertyCode |
Prop Code |
Center |
65 |
colPropertyType |
Prop Type |
|
75 |
colContractLength |
Contract Length |
|
88 |
colRentStartDate |
Rent Start Date |
Center |
88 |
colMonthlyRent |
Monthly Rent |
Right |
76 |
- Design the form as follows:
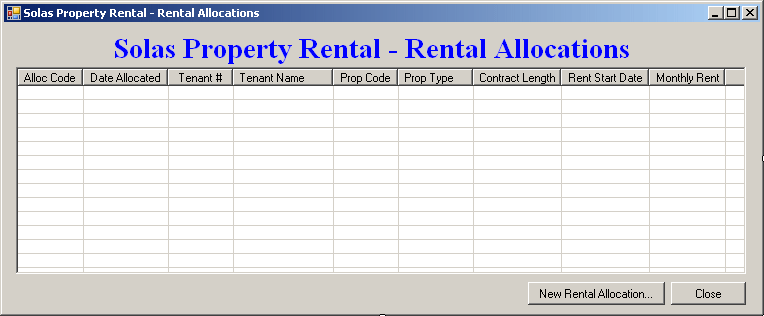
|
Control |
Text |
Name |
Other Properties |
ListView |
|
lvwAllocations |
View: Details GridLines: True
FullRowSelect: True Anchor: Top, Bottom, Left,
Right |
Button |
New Rental Allocation... |
btnNewAllocation |
Anchor: Bottom, Right |
Button |
Close |
btnClose |
|
|
- Double-click an unoccupied area of the RentalAllocations form and
implement the Load event as follows:
using System;
using System.Collections.Generic;
using System.ComponentModel;
using System.Data;
using System.Drawing;
using System.Linq;
using System.Text;
using System.Windows.Forms;
using System.IO;
using System.Xml;
namespace SolasPropertyRental1
{
public partial class RentalAllocations : Form
{
public RentalAllocations()
{
InitializeComponent();
}
void ShowRentalAllocations()
{
string Filename = @"C:\Solas Property Rental\contracts.xml";
XmlDocument docAllocations = new XmlDocument();
if (File.Exists(Filename))
{
lvwAllocations.Items.Clear();
docAllocations.Load(Filename);
XmlElement elmAllocation = docAllocations.DocumentElement;
XmlNodeList lstAllocations = elmAllocation.ChildNodes;
foreach (XmlNode node in lstAllocations)
{
ListViewItem lviAllocation = new ListViewItem(node.FirstChild.InnerText); // Allocation Code
lviAllocation.SubItems.Add(node.FirstChild.NextSibling.InnerText); // Date Allocated
lviAllocation.SubItems.Add(node.FirstChild.NextSibling.NextSibling.InnerText); // Tenant Account Number
lviAllocation.SubItems.Add(node.FirstChild.NextSibling.NextSibling.NextSibling.InnerText); // Tenant Name
lviAllocation.SubItems.Add(node.FirstChild.NextSibling.NextSibling.NextSibling.NextSibling.NextSibling.InnerText); // Property Code
lviAllocation.SubItems.Add(node.FirstChild.NextSibling.NextSibling.NextSibling.NextSibling.NextSibling.NextSibling.InnerText); // Property Type
lviAllocation.SubItems.Add(node.FirstChild.NextSibling.NextSibling.NextSibling.NextSibling.NextSibling.NextSibling.NextSibling.InnerText); // Contract Length
lviAllocation.SubItems.Add(node.FirstChild.NextSibling.NextSibling.NextSibling.NextSibling.NextSibling.NextSibling.NextSibling.NextSibling.InnerText); // Rent Start Date
lviAllocation.SubItems.Add(node.FirstChild.NextSibling.NextSibling.NextSibling.NextSibling.NextSibling.NextSibling.NextSibling.NextSibling.NextSibling.InnerText); // Monthly Rent
lvwAllocations.Items.Add(lviAllocation);
}
}
}
private void RentalAllocations_Load(object sender, EventArgs e)
{
ShowRentalAllocations();
}
}
}
- To add a new form to the application, in the Solution Explorer,
right-click SolasPropertyRental1 -> Add -> Windows Form...
- Set the Name to RentPayment and click Add
- Design the form as follows:
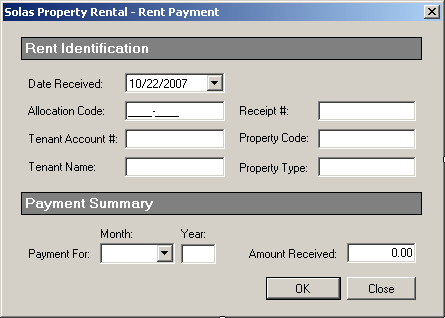 |
Control |
Text |
Name |
Other Properties |
Label |
Rent Allocation |
|
AutoSize: False BackColor: Gray
BorderStyle: FixedSingle ForeColor: White
TextAlign: MiddleLeft |
Label |
Date Received: |
|
|
DateTimePicker |
|
dtpDateReceived |
Format: Short Modifiers: Public |
Label |
Allocation Code: |
|
|
MaskedTextBox |
|
txtAllocationCode |
Mask: 0000-9999 Modifiers: Public |
Label |
Receipt #: |
|
|
TextBox |
|
txtReceiptNumber |
Modifiers: Public |
Label |
Tenant Account #: |
|
|
TextBox |
|
txtTenantAcntNber |
Modifiers: Public |
Label |
Property Code: |
|
|
TextBox |
|
txtPropertyCode |
Modifiers: Public |
Label |
Tenant Name: |
|
|
TextBox |
|
txtTenantName |
Modifiers: Public |
Label |
Property Type: |
|
|
TextBox |
|
txtPropertyType |
Modifiers: Public |
Label |
Payment Summary |
|
AutoSize: False BackColor: Gray
BorderStyle: FixedSingle ForeColor: White
TextAlign: MiddleLeft |
Label |
Month: |
|
|
Label |
Year: |
|
|
Label |
Payment For: |
|
|
ComboBox |
|
cbxMonths |
Modifiers: Public Items: January
February March April May June July
August September October November December |
TextBox |
|
txtYear |
Modifiers: Public |
Label |
Amount Received: |
|
|
TextBox |
0.00 |
txtAmountReceived |
TextAlign: Right Modifiers: Public |
Button |
OK |
btnOK |
DialogResult: OK |
Button |
Close |
btnClose |
DialogResult: Cancel |
Form |
|
|
AcceptButton: btnOK CancelButton:
btnClose FormBorderStyle: FixedDialog MaximizeBox:
False MinimizeBox: False ShowInTaskbar: False |
|
- On the form, click the Allocation Code text box and, in the Events
section of the Properties window, double-click Leave
- Implement the event as follows:
using System;
using System.Collections.Generic;
using System.ComponentModel;
using System.Data;
using System.Drawing;
using System.Linq;
using System.Text;
using System.Windows.Forms;
using System.IO;
using System.Xml;
namespace SolasPropertyRental1
{
public partial class RentPayment : Form
{
public RentPayment()
{
InitializeComponent();
}
private void txtAllocationCode_Leave(object sender, EventArgs e)
{
string Filename = @"C:\Solas Property Rental\contracts.xml";
XmlDocument docAllocations = new XmlDocument();
if (File.Exists(Filename))
{
docAllocations.Load(Filename);
XmlElement elmAllocation = docAllocations.DocumentElement;
XmlNodeList lstAllocations = elmAllocation.ChildNodes;
bool ContractFound = false;
for (int i = 0; i < lstAllocations.Count; i++)
{
XmlNode node = lstAllocations[i];
if (node.FirstChild.InnerText == txtAllocationCode.Text)
{
ContractFound = true;
txtTenantAcntNber.Text = node.FirstChild.NextSibling.NextSibling.InnerText;
txtTenantName.Text = node.FirstChild.NextSibling.NextSibling.NextSibling.InnerText;
txtPropertyCode.Text = node.FirstChild.NextSibling.NextSibling.NextSibling.NextSibling.NextSibling.InnerText;
txtPropertyType.Text = node.FirstChild.NextSibling.NextSibling.NextSibling.NextSibling.NextSibling.NextSibling.InnerText;
txtAmountReceived.Text = node.FirstChild.NextSibling.NextSibling.NextSibling.NextSibling.NextSibling.NextSibling.NextSibling.NextSibling.NextSibling.InnerText;
}
}
if (ContractFound == false)
{
MessageBox.Show("There is no rental contral with that number");
txtTenantAcntNber.Text = "";
}
}
else
MessageBox.Show("There is no list of rental contracts to check.");
}
}
}
- To add a new form to the application, in the Solution Explorer,
right- click SolasPropertyRental1 -> Add -> Windows Form...
- Set the Name to RentPayments and press Enter
- From the Toolbox, add a ListView to the form
- While the new list view is still selected, in the Properties window,
click the ellipsis button of the Columns field and create the columns as
follows:
(Name) |
Text |
TextAlign |
Width |
colReceiptNumber |
Receipt # |
Right |
|
colDateReceived |
Date Received |
Center |
85 |
colAllocationCode |
Alloc Code |
Center |
65 |
colTenantAccount |
Tenant # |
Center |
65 |
colTenantName |
Tenant Name |
|
100 |
colPropertyCode |
Prop Code |
Center |
65 |
colPropertyType |
Prop Type |
|
75 |
colPaymentFor |
Payment For |
|
88 |
colAmountReceived |
Amount |
Right |
50 |
- Design the form as follows:
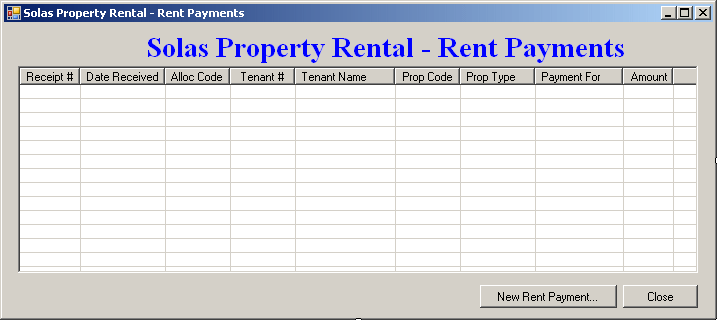
Control |
Text |
Name |
Other Properties |
ListView |
|
lvwRentPayments |
View: Details GridLines: True
FullRowSelect: True Anchor: Top, Bottom, Left, Right |
Button |
New Rent Payment... |
btnNewPayment |
Anchor: Bottom, Right |
Button |
Close |
btnClose |
Anchor: Bottom, Right |
- Double-click an unoccupied area of the RentPayments form and
implement the Load event as follows:
using System;
using System.Collections.Generic;
using System.ComponentModel;
using System.Data;
using System.Drawing;
using System.Linq;
using System.Text;
using System.Windows.Forms;
using System.IO;
using System.Xml;
namespace SolasPropertyRental1
{
public partial class RentPayments : Form
{
public RentPayments()
{
InitializeComponent();
}
void ShowRentPayments()
{
string Filename = @"C:\Solas Property Rental\payments.xml";
XmlDocument docPayments = new XmlDocument();
if (File.Exists(Filename))
{
lvwRentPayments.Items.Clear();
docPayments.Load(Filename);
XmlElement elmAllocation = docPayments.DocumentElement;
XmlNodeList lstAllocations = elmAllocation.ChildNodes;
foreach (XmlNode node in lstAllocations)
{
ListViewItem lviPayment = new ListViewItem(node.FirstChild.InnerText); // Receipt Number
lviPayment.SubItems.Add(node.FirstChild.NextSibling.InnerText); // Date Received
lviPayment.SubItems.Add(node.FirstChild.NextSibling.NextSibling.InnerText); // Allocation Code
lviPayment.SubItems.Add(node.FirstChild.NextSibling.NextSibling.NextSibling.InnerText); // Tenant Account Number
lviPayment.SubItems.Add(node.FirstChild.NextSibling.NextSibling.NextSibling.NextSibling.InnerText); // Tenant Name
lviPayment.SubItems.Add(node.FirstChild.NextSibling.NextSibling.NextSibling.NextSibling.NextSibling.InnerText); // Property Code
lviPayment.SubItems.Add(node.FirstChild.NextSibling.NextSibling.NextSibling.NextSibling.NextSibling.NextSibling.InnerText); // Property Type
lviPayment.SubItems.Add(node.FirstChild.NextSibling.NextSibling.NextSibling.NextSibling.NextSibling.NextSibling.NextSibling.InnerText); // Payment For
lviPayment.SubItems.Add(node.FirstChild.NextSibling.NextSibling.NextSibling.NextSibling.NextSibling.NextSibling.NextSibling.NextSibling.InnerText); // Amount
lvwRentPayments.Items.Add(lviPayment);
}
}
}
private void RentPayments_Load(object sender, EventArgs e)
{
ShowRentPayments();
}
}
}
- In the Solution Explorer, right-click Form1.cs and click Rename
- Set the name to Central.cs and press Enter twice to display
the form
- Design the form as follows:
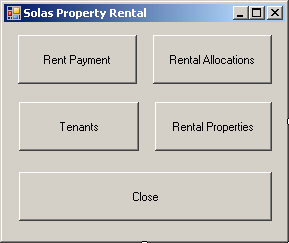 |
Control |
Text |
Name |
Button |
Tenants |
btnTenants |
Button |
Rental Properties |
btnRentalProperties |
Button |
Rental Allocations |
btnRentalAllocations |
Button |
C&lose |
btnClose |
|
- Double-click the Rent Payment button and implement its event as
follows:
private void btnRentPayment_Click(object sender, EventArgs e)
{
RentPayments frmPayment = new RentPayments();
frmPayment.Show();
}
- Return to the Central form
- Double-click the Rental Allocations button and implement its event
as follows:
private void btnRentalAllocations_Click(object sender, EventArgs e)
{
RentalAllocations frmAllocations = new RentalAllocations();
frmAllocations.ShowDialog();
}
- Return to the Central form
- Double-click the Tenants button and implement its event as follows:
private void btnTenants_Click(object sender, EventArgs e)
{
Tenants frmTenants = new Tenants();
frmTenants.ShowDialog();
}
- Return to the Central form
- Double-click the Rental Properties button and implement its event as
follows:
private void btnRentalProperties_Click(object sender, EventArgs e)
{
RentalProperties frmProperties = new RentalProperties();
frmProperties.ShowDialog();
}
- Return to the Central form
- Double-click the Close button and implement its event as follows:
private void btnClose_Click(object sender, EventArgs e)
{
Close();
}
- Save all
Practical Learning: Introducing operations on XML Elements
|
|
- Access the Tenants.cs [Design] tab and double-click the New Tenant
button
- Implement the Click event as follows:
private void btnNewTenant_Click(object sender, EventArgs e)
{
string AccountNumber;
string FullName, MaritalStatus, PhoneNumber;
// If this directory doesn't exist, create it
Directory.CreateDirectory(@"C:\Solas Property Rental\");
// This is the XML file that holds the list of tenants
string Filename = @"C:\Solas Property Rental\tenants.xml";
// This is the dialog box from where a tenant is created
TenantEditor editor = new TenantEditor();
// Display the Tenant Editor Dialog and find out if the
// user clicked OK after filling its controls
if (editor.ShowDialog() == DialogResult.OK)
{
// We will need a reference to the XML document
XmlDocument docTenant = new XmlDocument();
// Find out if the file exists already
// If it doesn't, then create it
if (!File.Exists(Filename))
{
docTenant.LoadXml("<?xml version=\"1.0\" encoding=\"utf-8\"?>" +
"<Tenants></Tenants>");
docTenant.Save(Filename);
}
// At this time, we have a Tenants.xml file. Open it
docTenant.Load(Filename);
// Get a reference to the root node
XmlElement nodRoot = docTenant.DocumentElement;
// Prepare the values of the XML element
AccountNumber = editor.txtAccountNumber.Text;
FullName = editor.txtFullName.Text;
MaritalStatus = editor.cbxMaritalStatus.Text;
PhoneNumber = editor.txtPhoneNumber.Text;
// Create an element named Tenant
XmlElement elmXML = docTenant.CreateElement("Tenant");
// Create the XML code of the child element of Tenant
string strNewCustomer = "<AccountNumber>" + AccountNumber +
"</AccountNumber>" +
"<FullName>" + FullName +
"</FullName>" +
"<MaritalStatus>" + MaritalStatus
+ "</MaritalStatus>" +
"<PhoneNumber>" + PhoneNumber +
"</PhoneNumber>";
elmXML.InnerXml = strNewCustomer;
// Append the new element as a child of Tenant
docTenant.DocumentElement.AppendChild(elmXML);
// Save the XML file
docTenant.Save(@"C:\Solas Property Rental\tenants.xml");
// Since the content of the XML file has just been changed,
// re-display the list of tenants
ShowTenants();
}
}
- Return to the Tenants form and double-click the Close button
- Implement its Click event as follows:
private void btnClose_Click(object sender, EventArgs e)
{
Close();
}
- Access the RentalProperties.cs [Design] tab and double-click the New
Property button
- Implement the Click event as follows:
private void btnNewProperty_Click(object sender, EventArgs e)
{
// If this directory doesn't exist, create it
Directory.CreateDirectory(@"C:\Solas Property Rental\");
// This is the XML file that holds the list of proeprties
string Filename = @"C:\Solas Property Rental\properties.xml";
PropertyEditor editor = new PropertyEditor();
Random rnd = new Random();
editor.txtPropertyCode.Text = rnd.Next(100000, 999999).ToString();
if (editor.ShowDialog() == DialogResult.OK)
{
string PropertyCode, PropertyType, Status;
int Bedrooms;
float Bathrooms;
double MonthlyRent;
// We will need a reference to the XML document
XmlDocument docProperty = new XmlDocument();
// Find out if the file exists already
// If it doesn't, then create it
if (!File.Exists(Filename))
{
docProperty.LoadXml("<?xml version=\"1.0\" encoding=\"utf-8\"?>" +
"<Properties></Properties>");
docProperty.Save(Filename);
}
// Open the XML file
docProperty.Load(Filename);
// Get a reference to the root node
XmlElement nodRoot = docProperty.DocumentElement;
PropertyCode = editor.txtPropertyCode.Text;
PropertyType = editor.cbxPropertyTypes.Text;
Bedrooms = int.Parse(editor.txtBedrooms.Text);
Bathrooms = float.Parse(editor.txtBathrooms.Text);
MonthlyRent = double.Parse(editor.txtMonthlyRent.Text);
Status = editor.cbxStatus.Text;
XmlElement elmXML = docProperty.CreateElement("Property");
string strNewCustomer = "<PropertyCode>" + PropertyCode +
"</PropertyCode>" + "<PropertyType>" +
PropertyType + "</PropertyType>" +
"<Bedrooms>" + Bedrooms + "</Bedrooms>" +
"<Bathrooms>" + Bathrooms.ToString("F") +
"</Bathrooms>" + "<MonthlyRent>" +
MonthlyRent.ToString("F") +
"</MonthlyRent>" +
"<Status>" + Status + "</Status>";
elmXML.InnerXml = strNewCustomer;
docProperty.DocumentElement.AppendChild(elmXML);
docProperty.Save(@"C:\Solas Property Rental\properties.xml");
ShowProperties();
}
}
- Return to the Rental Properties form and double-click the Close
button
- Implement its Click event as follows:
private void btnClose_Click(object sender, EventArgs e)
{
Close();
}
- Execute the application
- Use the Tenants button and its New Tenant button to create the
following Tenants:
Account # |
Full Name |
Marital Status |
Phone # |
20-48-46 |
Lenny Crandon |
Single |
(240) 975-9093 |
57-97-15 |
Joan Ramey |
Married |
(301) 304-5845 |
19-38-84 |
Peter Sellars |
Married |
(240) 801-7974 |
24-68-84 |
Alberta Sanson |
Separated |
(202) 917-0095 |
47-80-95 |
Urlus Flack |
Single |
(703) 203-7947 |
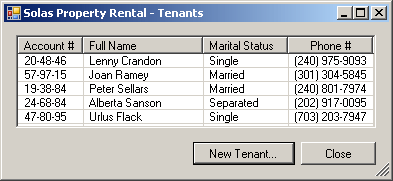 |
- Use the Rental Properties button and its New Property button to
create the following properties:
Prop Code |
Property Types |
Bedrooms |
Bathrooms |
Monthly Rent |
Status |
527-992 |
Apartment |
1 |
1 |
925 |
Available |
726-454 |
Apartment |
2 |
1 |
1150.5 |
Available |
476-473 |
Single Family |
5 |
3.5 |
2250.85 |
Occupied |
625-936 |
Townhouse |
3 |
2.5 |
1750 |
Available |
179-738 |
Townhouse |
4 |
2.5 |
1920.5 |
Available |
727-768 |
Single Family |
4 |
2.5 |
2140.5 |
Needs Repair |
371-801 |
Apartment |
3 |
2 |
1250.25 |
Available |
241-536 |
Townhouse |
3 |
1.5 |
1650.5 |
Occupied |
|
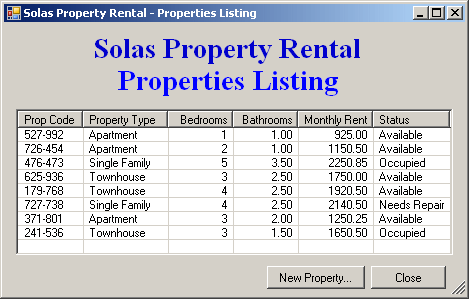 |
- Close the forms and return to your programming environment
Practical Learning: Programmatically Adding an Element
|
|
- Access the Rental Allocations form and double-click the New
Allocation button
- Implement the event as follows:
private void btnNewAllocation_Click(object sender, EventArgs e)
{
string AllocationCode, TenantAccountNumber,
TenantName, MaritalStatus,
PropertyCode, PropertyType, ContractLength;
DateTime DateAllocated, RentStartDate;
double MonthlyRent;
RentalAllocation editor = new RentalAllocation();
Directory.CreateDirectory(@"C:\Solas Property Rental");
string Filename = @"C:\Solas Property Rental\contracts.xml";
if (editor.ShowDialog() == DialogResult.OK)
{
XmlDocument docAllocation = new XmlDocument();
if (!File.Exists(Filename))
{
docAllocation.LoadXml("<?xml version=\"1.0\" encoding=\"utf-8\"?>" +
"<Allocations></Allocations>");
docAllocation.Save(Filename);
}
docAllocation.Load(Filename);
XmlElement nodRoot = docAllocation.DocumentElement;
AllocationCode = editor.txtAllocationCode.Text;
DateAllocated = editor.dtpDateAllocated.Value;
TenantAccountNumber = editor.txtTenantAcntNber.Text;
TenantName = editor.txtTenantName.Text;
MaritalStatus = editor.txtMaritalStatus.Text;
PropertyCode = editor.txtPropertyCode.Text;
PropertyType = editor.txtPropertyType.Text;
MonthlyRent = double.Parse(editor.txtMonthlyRent.Text);
ContractLength = editor.cbxContractLengths.Text;
RentStartDate = editor.dtpRentStartDate.Value;
XmlElement elmRoot = docAllocation.DocumentElement;
XmlElement elmAllocation =
docAllocation.CreateElement("RentalAllocation");
elmRoot.AppendChild(elmAllocation);
elmRoot = docAllocation.DocumentElement;
elmAllocation = docAllocation.CreateElement("AllocationCode");
XmlText txtAllocation =
docAllocation.CreateTextNode(AllocationCode);
elmRoot.LastChild.AppendChild(elmAllocation);
elmRoot.LastChild.LastChild.AppendChild(txtAllocation);
elmAllocation = docAllocation.CreateElement("DateAllocated");
txtAllocation =
docAllocation.CreateTextNode(DateAllocated.ToString("d"));
elmRoot.LastChild.AppendChild(elmAllocation);
elmRoot.LastChild.LastChild.AppendChild(txtAllocation);
elmAllocation = docAllocation.CreateElement("TenantAccountNumber");
txtAllocation = docAllocation.CreateTextNode(TenantAccountNumber);
elmRoot.LastChild.AppendChild(elmAllocation);
elmRoot.LastChild.LastChild.AppendChild(txtAllocation);
elmAllocation = docAllocation.CreateElement("TenantName");
txtAllocation = docAllocation.CreateTextNode(TenantName);
elmRoot.LastChild.AppendChild(elmAllocation);
elmRoot.LastChild.LastChild.AppendChild(txtAllocation);
elmAllocation = docAllocation.CreateElement("MaritalStatus");
txtAllocation = docAllocation.CreateTextNode(MaritalStatus);
elmRoot.LastChild.AppendChild(elmAllocation);
elmRoot.LastChild.LastChild.AppendChild(txtAllocation);
elmAllocation = docAllocation.CreateElement("PropertyCode");
txtAllocation = docAllocation.CreateTextNode(PropertyCode);
elmRoot.LastChild.AppendChild(elmAllocation);
elmRoot.LastChild.LastChild.AppendChild(txtAllocation);
elmAllocation = docAllocation.CreateElement("PropertyType");
txtAllocation = docAllocation.CreateTextNode(PropertyType);
elmRoot.LastChild.AppendChild(elmAllocation);
elmRoot.LastChild.LastChild.AppendChild(txtAllocation);
elmAllocation = docAllocation.CreateElement("ContractLength");
txtAllocation = docAllocation.CreateTextNode(ContractLength);
elmRoot.LastChild.AppendChild(elmAllocation);
elmRoot.LastChild.LastChild.AppendChild(txtAllocation);
elmAllocation = docAllocation.CreateElement("RentStartDate");
txtAllocation =
docAllocation.CreateTextNode(RentStartDate.ToString("d"));
elmRoot.LastChild.AppendChild(elmAllocation);
elmRoot.LastChild.LastChild.AppendChild(txtAllocation);
elmAllocation = docAllocation.CreateElement("MonthlyRent");
txtAllocation =
docAllocation.CreateTextNode(MonthlyRent.ToString("F"));
elmRoot.LastChild.AppendChild(elmAllocation);
elmRoot.LastChild.LastChild.AppendChild(txtAllocation);
docAllocation.Save(Filename);
ShowRentalAllocations();
}
}
- Return to the Rental Allocation form and double-click the Close
button
- Implement the event as follows:
private void btnClose_Click(object sender, EventArgs e)
{
Close();
}
- Access the Rent Payments form and double-click the New Rent Payment
button
- Implement the event as follows:
private void btnNewPayment_Click(object sender, EventArgs e)
{
int ReceiptNumber;
string AllocationCode,
TenantAccountNumber, TenantName,
PaymentFor, PropertyCode, PropertyType;
DateTime DateReceived;
double AmountReceived;
RentPayment editor = new RentPayment();
XmlDocument docPayment = new XmlDocument();
Directory.CreateDirectory(@"C:\Solas Property Rental");
string Filename = @"C:\Solas Property Rental\payments.xml";
// If some payments were previously made
if (File.Exists(Filename))
{
// Open the payments.xml file
docPayment.Load(Filename);
// Locate the root element
XmlElement elmAllocation = docPayment.DocumentElement;
// Get a list of the child nodes
XmlNodeList lstAllocations = elmAllocation.ChildNodes;
// Get the last receipt number
ReceiptNumber =
int.Parse(lstAllocations[lstAllocations.Count
- 1].FirstChild.InnerText) + 1;
editor.txtReceiptNumber.Text = ReceiptNumber.ToString();
}
else
{
// If no payment has ever been made,
// create a new XML file for the payments
docPayment.LoadXml("<?xml version=\"1.0\" encoding=\"utf-8\"?>" +
"<RentPayments></RentPayments>");
// We will start the receipt numbers at 101
ReceiptNumber = 101;
editor.txtReceiptNumber.Text = ReceiptNumber.ToString();
}
// Display the Rent Payment dialog box
if (editor.ShowDialog() == DialogResult.OK)
{
// If the user had clicked OK,
// Prepare the elements of the XML file
ReceiptNumber = int.Parse(editor.txtReceiptNumber.Text);
DateReceived = editor.dtpDateReceived.Value;
AllocationCode = editor.txtAllocationCode.Text;
TenantAccountNumber = editor.txtTenantAcntNber.Text;
TenantName = editor.txtTenantName.Text;
PropertyCode = editor.txtPropertyCode.Text;
PropertyType = editor.txtPropertyType.Text;
PaymentFor = editor.cbxMonths.Text + " " + editor.txtYear.Text;
AmountReceived = double.Parse(editor.txtAmountReceived.Text);
// Get a reference to the root element
XmlElement elmRoot = docPayment.DocumentElement;
// Create an element named Payment
XmlElement elmPayment = docPayment.CreateElement("Payment");
// Add the new element as the last node of the root element
elmRoot.AppendChild(elmPayment);
// Get a reference to the root element
elmRoot = docPayment.DocumentElement;
// Create an element
elmPayment = docPayment.CreateElement("ReceiptNumber");
// Create the value of the new element
XmlText txtPayment = docPayment.CreateTextNode(ReceiptNumber.ToString());
// Add the new element as a child of the Payment element
elmRoot.LastChild.AppendChild(elmPayment);
// Specify the value of the new element
elmRoot.LastChild.LastChild.AppendChild(txtPayment);
// Follow the same logic for the other elements
elmPayment = docPayment.CreateElement("DateReceived");
txtPayment = docPayment.CreateTextNode(DateReceived.ToString("d"));
elmRoot.LastChild.AppendChild(elmPayment);
elmRoot.LastChild.LastChild.AppendChild(txtPayment);
elmPayment = docPayment.CreateElement("AllocationCode");
XmlText txtAllocation = docPayment.CreateTextNode(AllocationCode);
elmRoot.LastChild.AppendChild(elmPayment);
elmRoot.LastChild.LastChild.AppendChild(txtAllocation);
elmPayment = docPayment.CreateElement("TenantAccountNumber");
txtAllocation = docPayment.CreateTextNode(TenantAccountNumber);
elmRoot.LastChild.AppendChild(elmPayment);
elmRoot.LastChild.LastChild.AppendChild(txtAllocation);
elmPayment = docPayment.CreateElement("TenantName");
txtAllocation = docPayment.CreateTextNode(TenantName);
elmRoot.LastChild.AppendChild(elmPayment);
elmRoot.LastChild.LastChild.AppendChild(txtAllocation);
elmPayment = docPayment.CreateElement("PropertyCode");
txtAllocation = docPayment.CreateTextNode(PropertyCode);
elmRoot.LastChild.AppendChild(elmPayment);
elmRoot.LastChild.LastChild.AppendChild(txtAllocation);
elmPayment = docPayment.CreateElement("PropertyType");
txtAllocation = docPayment.CreateTextNode(PropertyType);
elmRoot.LastChild.AppendChild(elmPayment);
elmRoot.LastChild.LastChild.AppendChild(txtAllocation);
elmPayment = docPayment.CreateElement("PaymentFor");
txtAllocation = docPayment.CreateTextNode(PaymentFor);
elmRoot.LastChild.AppendChild(elmPayment);
elmRoot.LastChild.LastChild.AppendChild(txtAllocation);
elmPayment = docPayment.CreateElement("AmountReceived");
txtAllocation = docPayment.CreateTextNode(AmountReceived.ToString("F"));
elmRoot.LastChild.AppendChild(elmPayment);
elmRoot.LastChild.LastChild.AppendChild(txtAllocation);
docPayment.Save(Filename);
ShowRentPayments();
}
}
- Return to the Rental Allocation form and double-click the Close
button
- Implement the event as follows:
private void btnClose_Click(object sender, EventArgs e)
{
Close();
}
- Execute the application
- Create the following Rental Allocations:
|
Allocation 1 |
Allocation 2 |
Allocation 3 |
Allocation 4 |
Allocation Code |
4205-8274 |
5920-9417 |
2792-4075 |
7957-7294 |
Date Allocated |
8/12/2002 |
2/18/2004 |
3/24/2004 |
10/26/2007 |
Account # |
24-68-84 |
57-97-15 |
19-38-84 |
20-48-46 |
Prop Code |
726-454 |
625-936 |
371-801 |
727-768 |
Contract Length |
12 Months |
3 Months |
12 Months |
6 Months |
Start Date |
10/1/2002 |
4/1/2004 |
6/1/2004 |
2/1/2008 |
- Create the following Rent Payments:
|
Date Received |
Allocation
Code |
Payment For |
Amount |
Month |
Year |
Payment 1 |
10/25/2002 |
4205-8274 |
October |
2002 |
1150.50 |
Payment 2 |
11/28/2002 |
4205-8274 |
November |
2002 |
1150.50 |
Payment 3 |
4/28/2004 |
5920-9417 |
April |
2004 |
1750.00 |
Payment 4 |
7/5/2004 |
2792-4075 |
June |
2004 |
1250.25 |
Payment 5 |
6/3/2004 |
5920-9417 |
May |
2004 |
1750.00 |
Payment 6 |
7/30/2004 |
2792-4075 |
July |
2004 |
1250.25 |
Payment 7 |
7/5/2004 |
5920-9417 |
June |
2004 |
1750.00 |
Payment 8 |
12/30/2002 |
4205-8274 |
December |
2002 |
1150.50 |
- Close the DOS window
- Open the Solas Property Rental database from this lesson
- Configure the Rental Allocations form so that, after the user has
entered a property code and press Tab (or when the control looses
focus), the database will first check the status of the property. If the
property is occupied, a message box will be displayed to the user and
that particular property cannot be selected (the property code text box
should be set empty)
- Configure the Rent Payments so that the user can decide to see only
the payments for one particular tenant (you can create a contextual menu
so that if the user right-clicks a certain row and click View Tenant's
Payments, the list view would display only that tenant's payments. Of
course, make sure the user has a way to display all payments again)
|
|